在Python中读取100G大文件的方法包括使用生成器、内存映射、分块读取等。 推荐的方法是使用生成器,因为它能够在不占用大量内存的前提下逐行读取文件内容,适用于处理大文件的场景。
一、生成器读取
生成器在读取大文件时非常高效,因为它可以在内存中逐行处理文件内容,而不是一次性加载整个文件。这样,即使是100G的大文件,也不会占用过多的内存。
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line
file_path = 'large_file.txt'
for line in read_large_file(file_path):
# Process the line
print(line)
这个方法利用了Python的生成器特性,通过yield
关键字逐行读取文件。这样做的好处在于内存占用非常低,即使文件非常大,也能顺利处理。
二、分块读取
分块读取也是处理大文件的常用方法。它通过分块读取文件内容,避免一次性加载整个文件,从而减少内存占用。
def read_file_in_chunks(file_path, chunk_size=1024):
with open(file_path, 'r') as file:
while True:
chunk = file.read(chunk_size)
if not chunk:
break
yield chunk
file_path = 'large_file.txt'
for chunk in read_file_in_chunks(file_path):
# Process the chunk
print(chunk)
在这个例子中,我们定义了一个read_file_in_chunks
函数,它接受文件路径和块大小作为参数。通过yield
关键字逐块读取文件内容,从而减少内存占用。
三、内存映射
内存映射(Memory Mapping)是一种将文件内容映射到内存的技术。这样可以提高文件读取速度,并且减少内存占用。
import mmap
def read_large_file_with_mmap(file_path):
with open(file_path, 'r') as file:
with mmap.mmap(file.fileno(), length=0, access=mmap.ACCESS_READ) as mmap_obj:
for line in iter(mmap_obj.readline, b""):
yield line.decode('utf-8')
file_path = 'large_file.txt'
for line in read_large_file_with_mmap(file_path):
# Process the line
print(line)
在这个例子中,我们使用了mmap
模块将文件内容映射到内存,并逐行读取文件内容。这样不仅提高了读取速度,还减少了内存占用。
四、Pandas库
Pandas库是一个强大的数据处理工具,虽然它通常用于处理结构化数据,但也可以用于读取大文件。Pandas的read_csv
函数支持分块读取。
import pandas as pd
def read_large_file_with_pandas(file_path, chunk_size=100000):
for chunk in pd.read_csv(file_path, chunksize=chunk_size):
# Process the chunk
print(chunk)
file_path = 'large_file.csv'
read_large_file_with_pandas(file_path)
在这个例子中,我们使用了Pandas的read_csv
函数,并设置了chunksize
参数来分块读取文件内容。这样做的好处在于可以利用Pandas强大的数据处理功能来处理大文件。
五、Dask库
Dask库是一个并行计算库,适用于处理大数据。它可以将大文件分成多个小块,并并行处理这些小块,从而提高处理速度。
import dask.dataframe as dd
def read_large_file_with_dask(file_path):
df = dd.read_csv(file_path)
# Process the dataframe
df.compute()
file_path = 'large_file.csv'
read_large_file_with_dask(file_path)
在这个例子中,我们使用了Dask的read_csv
函数读取大文件,并将其转换为Dask DataFrame。通过compute
方法,我们可以并行处理这些数据,提高处理速度。
六、异步读取
异步读取是处理大文件的另一种高效方法。它可以在不阻塞主线程的情况下读取文件内容,从而提高处理速度。
import aiofiles
import asyncio
async def read_large_file(file_path):
async with aiofiles.open(file_path, 'r') as file:
async for line in file:
# Process the line
print(line)
file_path = 'large_file.txt'
asyncio.run(read_large_file(file_path))
在这个例子中,我们使用了aiofiles
库进行异步文件读取。通过async
和await
关键字,我们可以在不阻塞主线程的情况下读取文件内容。
七、总结
处理100G大文件在Python中有多种方法,包括生成器、分块读取、内存映射、Pandas库、Dask库和异步读取。每种方法都有其优势和适用场景。推荐使用生成器,因为它能够在不占用大量内存的前提下逐行读取文件内容,适用于处理大文件的场景。当然,根据具体需求,可以选择最适合的方法来处理大文件。
相关问答FAQs:
如何使用Python有效读取大型文件?
在处理100GB的大文件时,建议使用逐行读取或分块读取的方法。您可以使用open()
函数结合循环,逐行读取文件内容,从而减少内存占用。示例代码如下:
with open('large_file.txt', 'r') as file:
for line in file:
# 处理每一行
另一种方法是使用pandas
库的read_csv()
函数,并设置chunksize
参数,这样可以分块读取数据,适合处理较大的数据集。
使用Python读取大文件时应该注意哪些性能优化?
读取大文件时,I/O操作往往是瓶颈。可以通过以下几种方式来优化性能:
- 使用
mmap
模块将文件映射到内存中,这样可以加快文件的读取速度。 - 调整文件的读取缓冲区大小,增大缓冲区可以减少读取次数,提高效率。
- 如果文件格式支持,可以考虑使用二进制格式,通常读取速度会更快。
在读取大文件时,如何处理内存不足的问题?
当内存不足时,可以采用以下策略:
- 分批次读取文件数据,处理完一批后再读取下一批,避免一次性加载整个文件。
- 使用
dask
库,它能够处理超出内存限制的数据集,提供类似于pandas
的API。 - 将数据写入数据库,而不是加载到内存中,处理完成后再进行查询和分析。
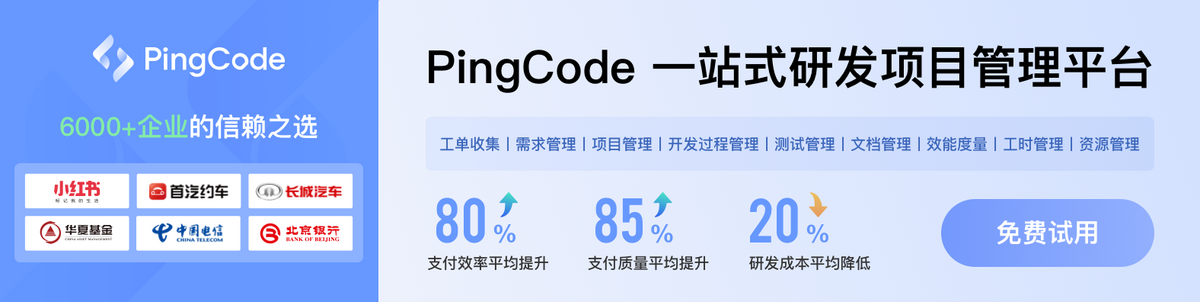