Python 给文档加数字签名的方法有:使用PyCryptodome库、使用PyOpenSSL库、使用M2Crypto库、使用cryptography库。
数字签名是一种用于验证信息完整性和身份验证的技术。通过生成数字签名,可以确保文档在传输过程中未被篡改,并确认发送者的身份。下面我们将详细介绍如何使用Python给文档加数字签名。
一、使用PyCryptodome库
PyCryptodome是一个功能强大的加密库,支持多种加密算法。我们可以使用它来生成和验证数字签名。
- 安装PyCryptodome库
pip install pycryptodome
- 生成密钥对
from Crypto.PublicKey import RSA
key = RSA.generate(2048)
private_key = key.export_key()
public_key = key.publickey().export_key()
with open("private.pem", "wb") as f:
f.write(private_key)
with open("public.pem", "wb") as f:
f.write(public_key)
- 生成数字签名
from Crypto.Signature import pkcs1_15
from Crypto.Hash import SHA256
message = b'Hello, this is a signed message.'
key = RSA.import_key(open('private.pem').read())
h = SHA256.new(message)
signature = pkcs1_15.new(key).sign(h)
with open("signature.bin", "wb") as f:
f.write(signature)
- 验证数字签名
from Crypto.Signature import pkcs1_15
from Crypto.Hash import SHA256
from Crypto.PublicKey import RSA
message = b'Hello, this is a signed message.'
key = RSA.import_key(open('public.pem').read())
h = SHA256.new(message)
with open("signature.bin", "rb") as f:
signature = f.read()
try:
pkcs1_15.new(key).verify(h, signature)
print("The signature is valid.")
except (ValueError, TypeError):
print("The signature is not valid.")
二、使用PyOpenSSL库
PyOpenSSL是Python的OpenSSL库的封装,可以处理SSL/TLS协议以及各种加密任务。
- 安装PyOpenSSL库
pip install pyopenssl
- 生成密钥对并保存
from OpenSSL import crypto
def generate_keys():
key = crypto.PKey()
key.generate_key(crypto.TYPE_RSA, 2048)
with open("private_key.pem", "wb") as f:
f.write(crypto.dump_privatekey(crypto.FILETYPE_PEM, key))
with open("public_key.pem", "wb") as f:
f.write(crypto.dump_publickey(crypto.FILETYPE_PEM, key))
generate_keys()
- 生成数字签名
from OpenSSL import crypto
def sign_message(message):
with open("private_key.pem", "rb") as f:
private_key = crypto.load_privatekey(crypto.FILETYPE_PEM, f.read())
signature = crypto.sign(private_key, message, "sha256")
with open("signature.bin", "wb") as f:
f.write(signature)
message = b"Hello, this is a signed message."
sign_message(message)
- 验证数字签名
from OpenSSL import crypto
def verify_message(message, signature):
with open("public_key.pem", "rb") as f:
public_key = crypto.load_publickey(crypto.FILETYPE_PEM, f.read())
try:
crypto.verify(public_key, signature, message, "sha256")
print("The signature is valid.")
except crypto.Error:
print("The signature is not valid.")
message = b"Hello, this is a signed message."
with open("signature.bin", "rb") as f:
signature = f.read()
verify_message(message, signature)
三、使用M2Crypto库
M2Crypto是一个Python的OpenSSL的封装库,提供了更丰富的加密功能。
- 安装M2Crypto库
pip install m2crypto
- 生成密钥对并保存
from M2Crypto import RSA
def generate_keys():
key = RSA.gen_key(2048, 65537)
key.save_key('private.pem', None)
key.save_pub_key('public.pem')
generate_keys()
- 生成数字签名
from M2Crypto import EVP
def sign_message(message):
key = EVP.load_key('private.pem')
key.sign_init()
key.sign_update(message)
signature = key.sign_final()
with open("signature.bin", "wb") as f:
f.write(signature)
message = b"Hello, this is a signed message."
sign_message(message)
- 验证数字签名
from M2Crypto import EVP
def verify_message(message, signature):
key = EVP.PKey()
key.assign_rsa(EVP.RSA.load_pub_key('public.pem'))
key.verify_init()
key.verify_update(message)
try:
if key.verify_final(signature) == 1:
print("The signature is valid.")
else:
print("The signature is not valid.")
except EVP.EVPError:
print("The signature is not valid.")
message = b"Hello, this is a signed message."
with open("signature.bin", "rb") as f:
signature = f.read()
verify_message(message, signature)
四、使用cryptography库
cryptography库是Python中广泛使用的加密库,提供了简洁的API和强大的功能。
- 安装cryptography库
pip install cryptography
- 生成密钥对并保存
from cryptography.hazmat.primitives.asymmetric import rsa
from cryptography.hazmat.primitives import serialization
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048,
)
with open("private_key.pem", "wb") as f:
f.write(private_key.private_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PrivateFormat.TraditionalOpenSSL,
encryption_algorithm=serialization.NoEncryption()
))
public_key = private_key.public_key()
with open("public_key.pem", "wb") as f:
f.write(public_key.public_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PublicFormat.SubjectPublicKeyInfo
))
- 生成数字签名
from cryptography.hazmat.primitives import hashes
from cryptography.hazmat.primitives.asymmetric import padding
def sign_message(message):
with open("private_key.pem", "rb") as f:
private_key = serialization.load_pem_private_key(f.read(), password=None)
signature = private_key.sign(
message,
padding.PSS(
mgf=padding.MGF1(hashes.SHA256()),
salt_length=padding.PSS.MAX_LENGTH
),
hashes.SHA256()
)
with open("signature.bin", "wb") as f:
f.write(signature)
message = b"Hello, this is a signed message."
sign_message(message)
- 验证数字签名
from cryptography.hazmat.primitives import hashes
from cryptography.hazmat.primitives.asymmetric import padding
from cryptography.hazmat.primitives import serialization
def verify_message(message, signature):
with open("public_key.pem", "rb") as f:
public_key = serialization.load_pem_public_key(f.read())
try:
public_key.verify(
signature,
message,
padding.PSS(
mgf=padding.MGF1(hashes.SHA256()),
salt_length=padding.PSS.MAX_LENGTH
),
hashes.SHA256()
)
print("The signature is valid.")
except cryptography.exceptions.InvalidSignature:
print("The signature is not valid.")
message = b"Hello, this is a signed message."
with open("signature.bin", "rb") as f:
signature = f.read()
verify_message(message, signature)
通过以上介绍,我们详细讲解了如何使用PyCryptodome、PyOpenSSL、M2Crypto和cryptography库来给文档加数字签名。每个库都有各自的特点和使用方法,您可以根据实际需求选择合适的库进行实现。数字签名技术在保证数据完整性和身份验证方面起到了至关重要的作用,希望本文能帮助您更好地理解和应用数字签名技术。
相关问答FAQs:
如何在Python中实现数字签名的过程?
在Python中,实现数字签名的过程通常涉及创建一个密钥对(公钥和私钥),然后使用私钥对文档进行签名。可以利用如PyCryptodome
、cryptography
等库来生成密钥并进行签名。签名后,接收方可以使用公钥验证签名的有效性,从而确保文档未被篡改。
使用Python为文档添加数字签名时,如何确保安全性?
确保安全性可以通过多个方面进行考虑。首先,选择一个强大的加密算法是至关重要的,比如RSA或ECDSA。其次,私钥应当妥善保管,不应暴露给任何未授权的用户。此外,可以对文档进行哈希处理,使用SHA-256等安全哈希算法,这样即使文档内容发生了微小变化,签名也会失效,从而增加安全性。
在Python中如何验证数字签名的有效性?
验证数字签名的过程涉及使用公钥来解密签名并与文档的哈希值进行比较。如果解密后的哈希值与文档生成的哈希值相同,则签名有效。可以利用Python中的cryptography
库来轻松实现这一过程,确保文档的完整性和来源的真实性。
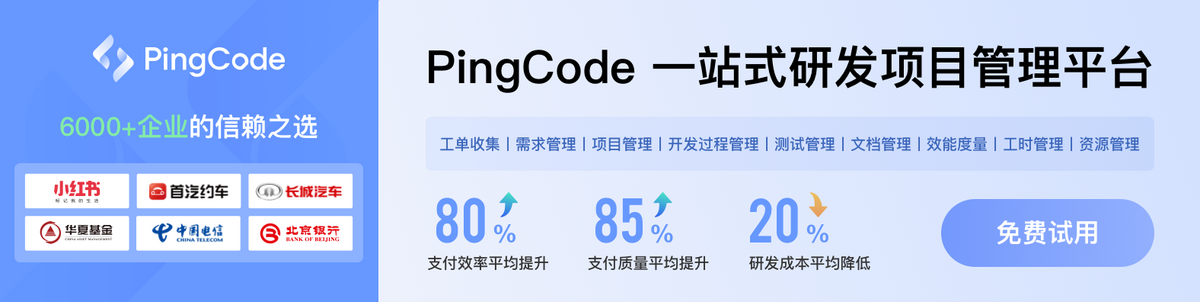