Python批量修改一份文件的方法有多种,包括使用文件读取与写入功能、利用正则表达式进行模式匹配和替换、以及结合文件操作库进行批量处理等。本文将详细介绍这些方法,并提供相应的代码示例。
在Python中,批量修改文件的核心步骤通常包括:读取文件内容、进行修改、将修改后的内容写回文件。下面我们将详细介绍这些步骤,并提供具体的代码示例。
一、读取文件内容
要对文件进行修改,首先需要读取文件的内容。在Python中,可以使用内置的open
函数来打开文件,并使用read
方法来读取文件内容。
# 读取文件内容
with open('example.txt', 'r', encoding='utf-8') as file:
content = file.read()
二、修改文件内容
读取文件内容后,下一步就是对内容进行修改。修改的方法可以根据具体需求选择,例如:字符串替换、正则表达式匹配替换等。下面介绍几种常见的修改方法。
1. 字符串替换
字符串替换是最简单的修改方法,使用Python的str.replace
方法可以轻松实现。
# 字符串替换
modified_content = content.replace('old_string', 'new_string')
2. 正则表达式匹配替换
正则表达式适用于更复杂的模式匹配和替换任务,Python的re
模块提供了强大的正则表达式功能。
import re
正则表达式匹配替换
pattern = re.compile(r'old_pattern')
modified_content = pattern.sub('new_string', content)
三、将修改后的内容写回文件
修改完成后,需要将修改后的内容写回文件。可以使用open
函数以写模式打开文件,并使用write
方法写入修改后的内容。
# 写入修改后的内容
with open('example.txt', 'w', encoding='utf-8') as file:
file.write(modified_content)
四、批量处理多个文件
如果需要批量处理多个文件,可以使用os
模块遍历指定目录下的所有文件,并对每个文件进行上述读取、修改、写入操作。
import os
指定目录
directory = 'path/to/directory'
遍历目录下的所有文件
for filename in os.listdir(directory):
filepath = os.path.join(directory, filename)
# 读取文件内容
with open(filepath, 'r', encoding='utf-8') as file:
content = file.read()
# 修改文件内容
modified_content = content.replace('old_string', 'new_string')
# 写入修改后的内容
with open(filepath, 'w', encoding='utf-8') as file:
file.write(modified_content)
五、处理大文件
对于大文件,建议逐行读取和处理,以避免内存占用过大。可以使用fileinput
模块逐行读取文件,并进行修改和写入。
import fileinput
指定大文件路径
file_path = 'large_file.txt'
逐行读取和修改
with fileinput.FileInput(file_path, inplace=True, backup='.bak', encoding='utf-8') as file:
for line in file:
# 修改每一行内容
modified_line = line.replace('old_string', 'new_string')
# 写入修改后的内容
print(modified_line, end='')
六、使用第三方库
除了使用Python内置的文件操作功能,还可以借助第三方库,如pandas
、pathlib
等,来简化文件处理任务。
1. 使用pandas处理CSV文件
对于CSV文件,可以使用pandas
库进行读取、修改和保存。
import pandas as pd
读取CSV文件
df = pd.read_csv('example.csv')
修改数据
df['column_name'] = df['column_name'].str.replace('old_string', 'new_string')
保存修改后的数据
df.to_csv('example.csv', index=False)
2. 使用pathlib遍历文件
pathlib
库提供了更直观的路径操作接口,可以结合os
模块进行文件遍历和处理。
from pathlib import Path
指定目录
directory = Path('path/to/directory')
遍历目录下的所有文件
for filepath in directory.iterdir():
if filepath.is_file():
# 读取文件内容
with open(filepath, 'r', encoding='utf-8') as file:
content = file.read()
# 修改文件内容
modified_content = content.replace('old_string', 'new_string')
# 写入修改后的内容
with open(filepath, 'w', encoding='utf-8') as file:
file.write(modified_content)
七、处理不同类型的文件
根据文件类型的不同,读取和修改方法也会有所不同。以下是几种常见文件类型的处理方法。
1. 处理JSON文件
对于JSON文件,可以使用Python的json
模块进行读取、修改和保存。
import json
读取JSON文件
with open('example.json', 'r', encoding='utf-8') as file:
data = json.load(file)
修改数据
data['key'] = data['key'].replace('old_string', 'new_string')
保存修改后的数据
with open('example.json', 'w', encoding='utf-8') as file:
json.dump(data, file, ensure_ascii=False, indent=4)
2. 处理XML文件
对于XML文件,可以使用xml.etree.ElementTree
模块进行解析和修改。
import xml.etree.ElementTree as ET
解析XML文件
tree = ET.parse('example.xml')
root = tree.getroot()
修改数据
for elem in root.iter('tag_name'):
elem.text = elem.text.replace('old_string', 'new_string')
保存修改后的数据
tree.write('example.xml', encoding='utf-8', xml_declaration=True)
3. 处理Excel文件
对于Excel文件,可以使用openpyxl
库进行读取、修改和保存。
from openpyxl import load_workbook
读取Excel文件
wb = load_workbook('example.xlsx')
ws = wb.active
修改数据
for row in ws.iter_rows():
for cell in row:
if isinstance(cell.value, str):
cell.value = cell.value.replace('old_string', 'new_string')
保存修改后的数据
wb.save('example.xlsx')
八、优化和扩展
在实际应用中,可以根据具体需求优化和扩展代码。例如,可以将文件处理逻辑封装成函数,便于复用和维护。
import os
import re
def modify_file_content(filepath, old_string, new_string):
with open(filepath, 'r', encoding='utf-8') as file:
content = file.read()
modified_content = content.replace(old_string, new_string)
with open(filepath, 'w', encoding='utf-8') as file:
file.write(modified_content)
def batch_modify_files(directory, old_string, new_string):
for filename in os.listdir(directory):
filepath = os.path.join(directory, filename)
if os.path.isfile(filepath):
modify_file_content(filepath, old_string, new_string)
示例用法
batch_modify_files('path/to/directory', 'old_string', 'new_string')
总结
通过本文的介绍,读者应该已经掌握了Python批量修改文件的多种方法,包括字符串替换、正则表达式匹配替换、逐行处理大文件、使用第三方库等。根据具体需求,选择合适的方法,可以高效地完成文件修改任务。
相关问答FAQs:
如何使用Python自动化批量修改文件内容?
使用Python进行批量文件修改时,可以利用内置的文件操作功能和模块,如os
和shutil
。首先,读取目标文件的内容,使用字符串方法进行修改,再将更新后的内容写回文件。可以通过循环遍历指定目录下的所有文件,实现批量处理。
在批量修改文件时,如何确保数据的安全性?
在进行批量文件修改时,建议先备份原始文件,以防止意外数据丢失或错误修改。可以创建一个新的文件夹,将原始文件复制到该文件夹中。使用shutil.copy()
方法可以简化备份过程,确保在修改过程中有恢复的选项。
Python中有哪些库可以帮助我更高效地批量处理文件?
除了内置的os
和shutil
库,pandas
库也非常适合处理数据文件,特别是CSV和Excel格式。它提供了丰富的数据处理功能,可以轻松读取、修改和保存数据文件。对于文本文件,使用re
模块进行正则表达式匹配与替换也会大大提高效率。
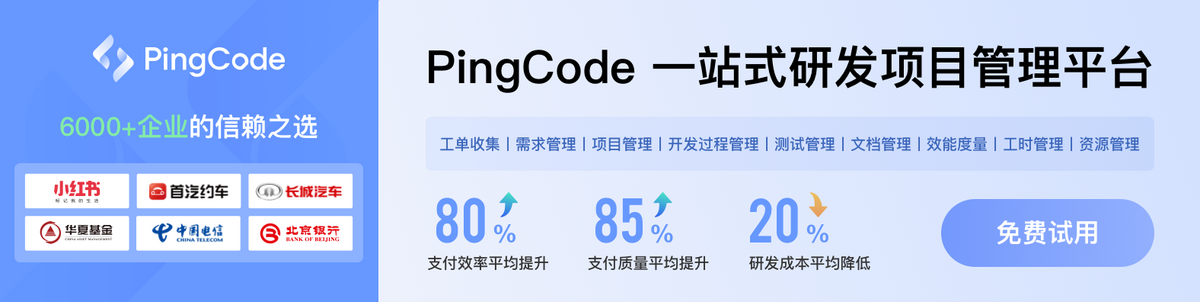