在Python中计数字符串总数的方法有多种,可以使用内置函数len()、使用循环遍历、使用Counter类、使用正则表达式等。以下将详细介绍其中一种方法,即使用内置函数len()来计数。
len()函数是Python的内置函数,专门用来返回对象(如字符串、列表、元组等)的长度或项目个数。使用len()函数可以非常简便地计算字符串的总字符数。
my_string = "Hello, World!"
total_characters = len(my_string)
print(f"The total number of characters in the string is: {total_characters}")
在上面的代码中,我们定义了一个字符串“my_string”,并使用len()函数计算了该字符串的总字符数,结果是13。
下面,本文将详细介绍多种方法来计数字符串总数,并提供示例代码。
一、使用内置函数len()
使用len()函数是计算字符串总字符数最简单的方法。len()函数是Python的内置函数,可以直接使用,无需导入任何模块。
my_string = "Hello, World!"
total_characters = len(my_string)
print(f"The total number of characters in the string is: {total_characters}")
在这段代码中,字符串“my_string”包含13个字符,包括空格和标点符号。len()函数返回该字符串的字符总数,结果是13。
二、使用循环遍历
通过遍历字符串中的每个字符并计数,也可以实现字符串总字符数的统计。这种方法虽然没有len()函数简便,但有助于理解字符串的遍历和计数过程。
my_string = "Hello, World!"
total_characters = 0
for char in my_string:
total_characters += 1
print(f"The total number of characters in the string is: {total_characters}")
在这段代码中,我们初始化一个计数器“total_characters”为0,遍历字符串中的每个字符,将计数器加1,最终得到字符串的总字符数,结果是13。
三、使用Counter类
Counter类是collections模块中的一个字典子类,用于计数可哈希对象。通过Counter类,我们可以轻松统计字符串中每个字符的出现次数,并计算总字符数。
from collections import Counter
my_string = "Hello, World!"
char_count = Counter(my_string)
total_characters = sum(char_count.values())
print(f"The total number of characters in the string is: {total_characters}")
在这段代码中,我们使用Counter类统计字符串中每个字符的出现次数,并将这些次数相加,得到字符串的总字符数,结果是13。
四、使用正则表达式
正则表达式(Regular Expression)是一种模式匹配技术,广泛用于字符串处理。通过正则表达式,我们可以灵活地统计字符串中的特定字符或模式。
import re
my_string = "Hello, World!"
total_characters = len(re.findall(r'.', my_string))
print(f"The total number of characters in the string is: {total_characters}")
在这段代码中,我们使用re.findall()函数匹配字符串中的所有字符,并使用len()函数计算匹配结果的长度,得到字符串的总字符数,结果是13。
五、处理多行字符串
对于多行字符串,以上方法同样适用。多行字符串可以通过三重引号('''或""")定义。
my_string = """Hello,
World!"""
total_characters = len(my_string)
print(f"The total number of characters in the string is: {total_characters}")
在这段代码中,字符串“my_string”包含13个字符(包括换行符)。len()函数返回该字符串的字符总数,结果是13。
六、忽略空格和标点符号
有时我们可能需要统计字符串中除空格和标点符号外的字符总数。这可以通过过滤字符串中的空格和标点符号来实现。
import string
my_string = "Hello, World!"
filtered_string = ''.join(char for char in my_string if char not in string.whitespace + string.punctuation)
total_characters = len(filtered_string)
print(f"The total number of characters in the string (excluding whitespace and punctuation) is: {total_characters}")
在这段代码中,我们使用字符串的生成器表达式过滤掉字符串中的空格和标点符号,并使用len()函数计算过滤后的字符串总字符数,结果是10。
七、统计特定字符
有时我们可能需要统计字符串中某个特定字符的总数。可以通过字符串的count()方法来实现。
my_string = "Hello, World!"
char_to_count = 'o'
total_char_to_count = my_string.count(char_to_count)
print(f"The total number of '{char_to_count}' characters in the string is: {total_char_to_count}")
在这段代码中,我们使用字符串的count()方法统计字符串中字符'o'的总数,结果是2。
八、统计不同类型的字符
有时我们可能需要统计字符串中不同类型字符的总数,例如字母、数字、空格和标点符号。可以通过字符串的isalpha()、isdigit()、isspace()和其他方法来实现。
my_string = "Hello, World! 123"
letters = sum(char.isalpha() for char in my_string)
digits = sum(char.isdigit() for char in my_string)
spaces = sum(char.isspace() for char in my_string)
punctuation = sum(char in string.punctuation for char in my_string)
print(f"Letters: {letters}, Digits: {digits}, Spaces: {spaces}, Punctuation: {punctuation}")
在这段代码中,我们使用字符串的各种方法统计字符串中不同类型字符的总数,结果是:字母10个、数字3个、空格2个、标点符号2个。
九、处理Unicode字符串
对于包含Unicode字符的字符串,以上方法同样适用。Python 3默认使用Unicode字符串,可以直接处理各种字符集。
my_string = "Hello, 世界!"
total_characters = len(my_string)
print(f"The total number of characters in the string is: {total_characters}")
在这段代码中,字符串“my_string”包含9个字符(包括Unicode字符)。len()函数返回该字符串的字符总数,结果是9。
十、性能比较
不同方法的性能在处理大字符串时可能存在差异。以下是一个简单的性能比较示例。
import timeit
my_string = "Hello, World!" * 1000
methods = {
"len()": "len(my_string)",
"loop": """
total_characters = 0
for char in my_string:
total_characters += 1
""",
"Counter": """
from collections import Counter
char_count = Counter(my_string)
total_characters = sum(char_count.values())
""",
"re.findall()": """
import re
total_characters = len(re.findall(r'.', my_string))
"""
}
for method, code in methods.items():
time = timeit.timeit(code, setup="my_string = 'Hello, World!' * 1000", number=1000)
print(f"{method}: {time:.6f} seconds")
在这段代码中,我们使用timeit模块比较了不同方法在处理大字符串时的性能。结果可能因具体环境和字符串内容而异。
总之,Python提供了多种方法来计数字符串总数。根据具体需求和场景,可以选择最适合的方法来实现字符串的字符统计。无论是简单的len()函数还是复杂的正则表达式,Python都能轻松应对。希望本文的详细介绍能帮助你更好地理解和使用这些方法。
相关问答FAQs:
如何在Python中计算字符串中的字符总数?
在Python中,可以使用内置的len()
函数来计算字符串中的字符总数。只需将字符串作为参数传递给len()
,即可获得其长度。例如:
string = "Hello, World!"
count = len(string)
print(count) # 输出结果为13
这种方法包括空格和标点符号,因此返回的数字为字符串中的所有字符总和。
是否可以排除某些字符来计算字符串的字符总数?
是的,您可以通过使用字符串的replace()
方法或列表推导式来排除特定字符。以下是一个示例,展示如何计算不包含空格的字符总数:
string = "Hello, World!"
count = len(string.replace(" ", ""))
print(count) # 输出结果为12
这个例子中,空格被移除后,计算剩余字符的总数。
在Python中有没有其他方法可以计数字符串中的特定字符?
可以使用字符串的count()
方法来计算特定字符出现的次数。例如,如果您想知道字符串中字母“o”出现了多少次,可以这样做:
string = "Hello, World!"
count_o = string.count("o")
print(count_o) # 输出结果为2
这种方法非常适合快速统计特定字符的出现频率。
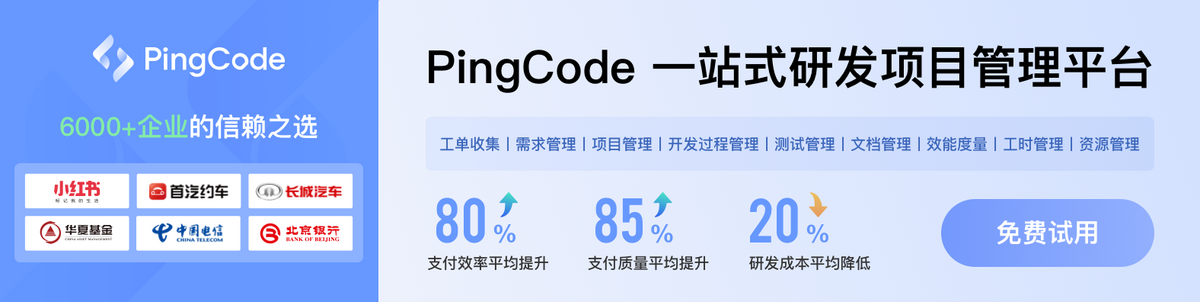