Python中可以通过以下几种方式将日期对象转换为日期字符串:使用strftime
方法、使用date.isoformat()
方法、使用format
方法、使用f-string
格式化。本文将详细介绍这些方法,并展示如何使用它们将日期对象转换为日期字符串。
一、使用strftime
方法
strftime
方法是Python中常用的日期格式化方法。该方法可以将日期对象转换为指定格式的字符串。以下是使用strftime
方法的示例:
from datetime import datetime
获取当前日期时间
now = datetime.now()
转换为字符串
date_str = now.strftime("%Y-%m-%d %H:%M:%S")
print(date_str)
在上面的示例中,strftime
方法接受一个格式化字符串作为参数,用于指定日期时间的输出格式。格式化字符串中的各个部分分别表示年、月、日、时、分、秒等。
二、使用date.isoformat()
方法
isoformat
方法是Python日期对象的内置方法,该方法可以将日期对象转换为ISO 8601格式的字符串。以下是使用isoformat
方法的示例:
from datetime import date
获取当前日期
today = date.today()
转换为ISO 8601格式字符串
date_str = today.isoformat()
print(date_str)
在上面的示例中,isoformat
方法将日期对象转换为"YYYY-MM-DD"格式的字符串。
三、使用format
方法
format
方法是Python字符串的内置方法,可以用于格式化日期时间字符串。以下是使用format
方法的示例:
from datetime import datetime
获取当前日期时间
now = datetime.now()
转换为字符串
date_str = "{:%Y-%m-%d %H:%M:%S}".format(now)
print(date_str)
在上面的示例中,format
方法接受一个日期对象作为参数,并将其转换为指定格式的字符串。
四、使用f-string
格式化
f-string
是Python 3.6引入的一种新的字符串格式化方式,可以将表达式嵌入到字符串中。以下是使用f-string
格式化日期时间字符串的示例:
from datetime import datetime
获取当前日期时间
now = datetime.now()
转换为字符串
date_str = f"{now:%Y-%m-%d %H:%M:%S}"
print(date_str)
在上面的示例中,f-string
通过在大括号内嵌入表达式来格式化日期时间字符串。
五、综合示例
下面是一个综合示例,展示了如何使用上述方法将日期对象转换为不同格式的字符串:
from datetime import datetime, date
获取当前日期时间
now = datetime.now()
使用strftime方法
date_str1 = now.strftime("%Y-%m-%d %H:%M:%S")
print(f"strftime: {date_str1}")
使用isoformat方法
today = date.today()
date_str2 = today.isoformat()
print(f"isoformat: {date_str2}")
使用format方法
date_str3 = "{:%Y-%m-%d %H:%M:%S}".format(now)
print(f"format: {date_str3}")
使用f-string格式化
date_str4 = f"{now:%Y-%m-%d %H:%M:%S}"
print(f"f-string: {date_str4}")
在这个综合示例中,我们展示了如何使用strftime
、isoformat
、format
方法和f-string
格式化方式将日期对象转换为字符串,并输出不同格式的日期字符串。
总结
本文介绍了Python中将日期对象转换为日期字符串的几种方法,包括strftime
方法、isoformat
方法、format
方法和f-string
格式化方式。通过这些方法,我们可以轻松地将日期对象转换为不同格式的字符串,以满足不同的需求。希望本文对您有所帮助,如果有任何问题或建议,欢迎在评论区留言。
相关问答FAQs:
如何在Python中将日期对象转换为字符串?
在Python中,可以使用strftime()
方法将日期对象转换为字符串。这个方法允许您指定日期字符串的格式。例如,date_object.strftime("%Y-%m-%d")
将日期对象格式化为“YYYY-MM-DD”的字符串格式。
在Python中支持哪些日期格式化符号?
Python的strftime()
方法支持多种格式化符号,例如:
%Y
:四位年份%m
:两位月份%d
:两位日期%H
:24小时制的小时%M
:分钟%S
:秒钟
通过组合这些符号,您可以创建自定义的日期字符串格式。
如何处理不合法的日期格式或转换错误?
在进行日期转换时,确保输入的日期对象是有效的。可以通过try...except
语句来捕捉可能的异常,例如ValueError
,这样可以处理无效日期导致的错误。提供清晰的错误提示可以帮助用户理解问题所在。
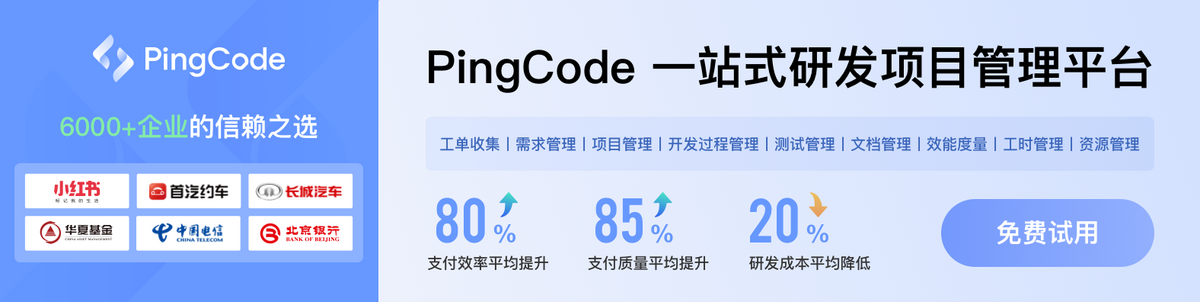