在Python中调用字符串,可以使用单引号或双引号、使用转义字符、使用多行字符串、使用字符串操作方法、使用格式化字符串。在Python中,字符串是非常常用的数据类型,掌握调用字符串的方法和技巧是非常重要的。其中,使用格式化字符串是一种非常有效和灵活的方法,下面我们详细讨论这一点。
使用格式化字符串,我们可以在字符串中嵌入变量或者表达式。Python提供了几种格式化字符串的方法,其中最常用的是f-string(格式化字符串字面值),它从Python 3.6版本开始引入。f-string使用简单的语法,灵活且高效。使用f-string时,只需要在字符串前加上字母f
或F
,然后在字符串中使用花括号{}
包裹变量或表达式即可。
例如:
name = "Alice"
age = 30
message = f"My name is {name} and I am {age} years old."
print(message)
在上面的代码中,name
和age
这两个变量被嵌入到字符串中,最终输出结果是"My name is Alice and I am 30 years old."
下面我们将详细介绍在Python中调用字符串的各种方法和技巧。
一、使用单引号或双引号
在Python中,可以使用单引号'
或双引号"
来定义字符串。两者在功能上没有区别,可以根据个人习惯或代码规范选择使用。
str1 = 'Hello, World!'
str2 = "Hello, Python!"
使用单引号或双引号的一个好处是,如果字符串本身包含单引号或双引号,可以使用另一种引号来避免转义。
例如:
str1 = "It's a beautiful day!"
str2 = 'She said, "Hello!"'
二、使用转义字符
有时候字符串中需要包含一些特殊字符,如换行符\n
、制表符\t
、单引号\'
、双引号\"
等,这时可以使用转义字符来表示。
str1 = "Hello,\nWorld!"
str2 = "She said, \"Hello!\""
在上面的代码中,\n
表示换行符,\"
表示双引号。
三、使用多行字符串
如果字符串需要跨多行,可以使用三重引号(单引号'''
或双引号"""
)来定义多行字符串。这种方法在编写文档字符串(docstring)时非常常用。
例如:
str1 = '''This is a
multi-line
string.'''
str2 = """This is another
multi-line
string."""
四、字符串操作方法
Python的字符串类提供了很多内置的方法,可以对字符串进行各种操作,如查找、替换、分割、连接等。常用的字符串操作方法包括:
upper()
:将字符串转换为大写lower()
:将字符串转换为小写strip()
:移除字符串两端的空白字符replace(old, new)
:将字符串中的old
子串替换为new
split(sep)
:根据指定的分隔符sep
将字符串分割成列表join(iterable)
:将可迭代对象中的元素连接成一个字符串
例如:
str1 = " Hello, World! "
str2 = str1.strip() # 移除两端空白字符
print(str2) # 输出: "Hello, World!"
str3 = "Hello, Python!"
str4 = str3.replace("Python", "World")
print(str4) # 输出: "Hello, World!"
str5 = "apple,banana,orange"
list1 = str5.split(",")
print(list1) # 输出: ['apple', 'banana', 'orange']
list2 = ["apple", "banana", "orange"]
str6 = ", ".join(list2)
print(str6) # 输出: "apple, banana, orange"
五、格式化字符串
在Python中,格式化字符串是一种非常常用和强大的功能。常用的格式化字符串方法包括%
操作符、str.format()
方法和f-string。
1. 使用%
操作符
%
操作符用于格式化字符串,类似于C语言的printf
。它支持多种格式化类型,如整数%d
、字符串%s
、浮点数%f
等。
例如:
name = "Alice"
age = 30
message = "My name is %s and I am %d years old." % (name, age)
print(message) # 输出: "My name is Alice and I am 30 years old."
2. 使用str.format()
方法
str.format()
方法是Python 2.6及以上版本提供的字符串格式化方法。它使用花括号{}
作为占位符,通过format()
方法传入参数进行格式化。
例如:
name = "Alice"
age = 30
message = "My name is {} and I am {} years old.".format(name, age)
print(message) # 输出: "My name is Alice and I am 30 years old."
str.format()
方法还支持命名参数和位置参数:
name = "Alice"
age = 30
message = "My name is {name} and I am {age} years old.".format(name=name, age=age)
print(message) # 输出: "My name is Alice and I am 30 years old."
3. 使用f-string
f-string(格式化字符串字面值)是Python 3.6及以上版本引入的格式化字符串方法。它使用简单的语法,灵活且高效。使用f-string时,只需要在字符串前加上字母f
或F
,然后在字符串中使用花括号{}
包裹变量或表达式。
例如:
name = "Alice"
age = 30
message = f"My name is {name} and I am {age} years old."
print(message) # 输出: "My name is Alice and I am 30 years old."
f-string还支持各种表达式和格式化选项:
import math
name = "Alice"
age = 30
pi = math.pi
message = f"My name is {name.upper()} and I am {age} years old. The value of pi is approximately {pi:.2f}."
print(message) # 输出: "My name is ALICE and I am 30 years old. The value of pi is approximately 3.14."
六、字符串切片
字符串切片是从字符串中提取子字符串的一种方法。它使用切片语法[start:stop:step]
,其中start
是起始索引,stop
是结束索引(不包含),step
是步长。
例如:
str1 = "Hello, World!"
sub_str1 = str1[7:12] # 提取子字符串"World"
print(sub_str1) # 输出: "World"
sub_str2 = str1[:5] # 提取子字符串"Hello"
print(sub_str2) # 输出: "Hello"
sub_str3 = str1[::2] # 每隔一个字符提取一次
print(sub_str3) # 输出: "Hlo ol!"
七、字符串的不可变性
需要注意的是,Python中的字符串是不可变的(immutable)。这意味着一旦字符串创建后,就不能修改其中的字符。任何对字符串的操作都会返回一个新的字符串,而不会修改原字符串。
例如:
str1 = "Hello, World!"
str2 = str1.replace("World", "Python")
print(str1) # 输出: "Hello, World!"
print(str2) # 输出: "Hello, Python!"
在上面的代码中,replace()
方法返回一个新的字符串str2
,而原字符串str1
保持不变。
八、字符串的编码和解码
在处理不同编码的字符串时,可能需要对字符串进行编码和解码。Python提供了encode()
和decode()
方法来实现这一功能。
例如:
str1 = "Hello, 世界!"
encoded_str = str1.encode('utf-8') # 将字符串编码为UTF-8字节序列
print(encoded_str) # 输出: b'Hello, \xe4\xb8\x96\xe7\x95\x8c!'
decoded_str = encoded_str.decode('utf-8') # 将UTF-8字节序列解码为字符串
print(decoded_str) # 输出: "Hello, 世界!"
在上面的代码中,encode('utf-8')
方法将字符串编码为UTF-8字节序列,decode('utf-8')
方法将UTF-8字节序列解码为字符串。
九、字符串的常见操作
以下是一些字符串的常见操作和技巧:
1. 判断字符串是否包含子字符串
可以使用in
运算符判断字符串是否包含子字符串:
str1 = "Hello, World!"
contains_world = "World" in str1
print(contains_world) # 输出: True
2. 判断字符串是否以特定前缀或后缀开头/结尾
可以使用startswith()
和endswith()
方法判断字符串是否以特定前缀或后缀开头/结尾:
str1 = "Hello, World!"
starts_with_hello = str1.startswith("Hello")
print(starts_with_hello) # 输出: True
ends_with_world = str1.endswith("World!")
print(ends_with_world) # 输出: True
3. 查找子字符串的位置
可以使用find()
和index()
方法查找子字符串在字符串中的位置:
str1 = "Hello, World!"
pos = str1.find("World") # 返回子字符串"World"的起始位置
print(pos) # 输出: 7
需要注意的是,如果子字符串不存在,find()
方法返回-1
,而index()
方法会引发ValueError
异常。
4. 统计子字符串的出现次数
可以使用count()
方法统计子字符串在字符串中出现的次数:
str1 = "Hello, World! Hello, Python!"
count_hello = str1.count("Hello")
print(count_hello) # 输出: 2
5. 替换子字符串
可以使用replace()
方法替换字符串中的子字符串:
str1 = "Hello, World!"
str2 = str1.replace("World", "Python")
print(str2) # 输出: "Hello, Python!"
6. 分割字符串
可以使用split()
方法根据指定的分隔符将字符串分割成列表:
str1 = "apple,banana,orange"
list1 = str1.split(",")
print(list1) # 输出: ['apple', 'banana', 'orange']
7. 连接字符串
可以使用join()
方法将可迭代对象中的元素连接成一个字符串:
list2 = ["apple", "banana", "orange"]
str2 = ", ".join(list2)
print(str2) # 输出: "apple, banana, orange"
十、字符串的高级操作
1. 使用正则表达式
正则表达式(regular expression)是一种强大的字符串匹配工具,Python的re
模块提供了对正则表达式的支持。可以使用正则表达式进行复杂的字符串匹配、查找、替换等操作。
例如:
import re
str1 = "Hello, World! Hello, Python!"
pattern = r"Hello"
matches = re.findall(pattern, str1)
print(matches) # 输出: ['Hello', 'Hello']
在上面的代码中,re.findall()
函数根据正则表达式pattern
查找字符串中的所有匹配项,并返回匹配项的列表。
2. 使用模板字符串
模板字符串(template string)是一种简单的字符串替换工具,Python的string
模块提供了对模板字符串的支持。可以使用模板字符串进行基本的字符串替换操作。
例如:
from string import Template
template = Template("My name is $name and I am $age years old.")
message = template.substitute(name="Alice", age=30)
print(message) # 输出: "My name is Alice and I am 30 years old."
在上面的代码中,Template.substitute()
方法根据模板字符串template
进行变量替换,并返回替换后的字符串。
结论
在Python中调用字符串的方法多种多样,包括使用单引号或双引号、使用转义字符、使用多行字符串、使用字符串操作方法、使用格式化字符串、字符串切片、字符串的编码和解码、字符串的常见操作和高级操作等。掌握这些方法和技巧,可以帮助我们在实际编程中更高效地处理字符串数据,提高代码的可读性和可维护性。希望通过本文的详细介绍,读者能够更深入地理解和灵活应用Python中的字符串操作。
相关问答FAQs:
在Python中,如何定义和使用字符串变量?
在Python中,字符串可以通过单引号、双引号或三引号来定义。例如,可以使用name = "Alice"
来定义一个字符串变量。使用print(name)
可以输出这个字符串。字符串可以包含字母、数字、符号和空格,并且支持多行文本的定义。
字符串拼接和格式化在Python中是如何实现的?
Python提供了多种方法来拼接字符串,例如使用+
运算符或join()
方法。格式化字符串可以通过f-string
(在Python 3.6及以上版本)或者format()
方法来实现。例如,使用f"Hello, {name}"
可以将变量name
的值嵌入到字符串中,生成一个新的字符串。
如何在Python中对字符串进行切片和操作?
字符串在Python中是可以切片的,这意味着你可以提取字符串的特定部分。例如,text = "Hello, World!"
,使用text[0:5]
可以获取“Hello”。此外,字符串提供了许多内置方法,如upper()
、lower()
、replace()
等,允许用户轻松地对字符串进行变换和处理。
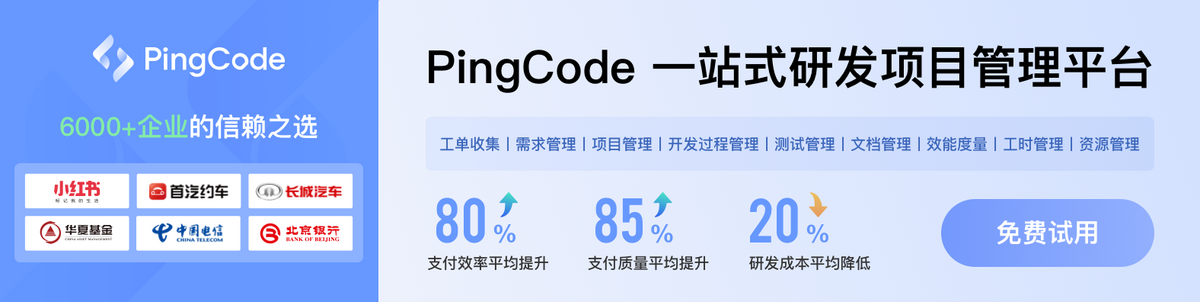