Python下载网络图片的大小:使用requests库、使用urllib库、使用aiohttp库
在Python中,有多种方法可以下载网络图片的大小。使用requests库、使用urllib库、使用aiohttp库。下面将详细介绍如何使用这些方法实现目标,并通过代码示例进行说明。首先,我们将介绍如何使用requests库下载网络图片的大小。
一、使用requests库
requests库是一个简单易用的HTTP库,用于发送HTTP请求。在使用requests库下载网络图片时,可以先发送HEAD请求获取图片的大小信息,然后再决定是否下载图片。以下是使用requests库的详细步骤:
- 安装requests库
pip install requests
- 使用requests库下载图片大小
import requests
def get_image_size(url):
response = requests.head(url)
size = response.headers.get('content-length', None)
if size:
return int(size)
else:
return None
示例
url = 'https://example.com/image.jpg'
size = get_image_size(url)
if size:
print(f'图片大小为: {size} 字节')
else:
print('无法获取图片大小')
在上述代码中,我们使用requests.head()方法发送HEAD请求,并从响应头中获取content-length字段,以确定图片的大小。如果content-length字段不存在,则返回None。
二、使用urllib库
urllib是Python标准库中的一个模块,用于处理URL和HTTP请求。与requests库类似,urllib库也可以发送HTTP请求并获取响应头信息。以下是使用urllib库下载网络图片大小的详细步骤:
- 导入urllib库
import urllib.request
- 使用urllib库下载图片大小
def get_image_size(url):
request = urllib.request.Request(url, method='HEAD')
with urllib.request.urlopen(request) as response:
size = response.getheader('Content-Length')
if size:
return int(size)
else:
return None
示例
url = 'https://example.com/image.jpg'
size = get_image_size(url)
if size:
print(f'图片大小为: {size} 字节')
else:
print('无法获取图片大小')
在上述代码中,我们使用urllib.request.Request()方法创建一个HEAD请求,并通过urllib.request.urlopen()方法发送请求。然后,从响应头中获取Content-Length字段,以确定图片的大小。如果Content-Length字段不存在,则返回None。
三、使用aiohttp库
aiohttp是一个支持异步HTTP请求的库,适用于需要并发处理的场景。在使用aiohttp库下载网络图片大小时,可以先发送HEAD请求获取图片的大小信息,然后再决定是否下载图片。以下是使用aiohttp库的详细步骤:
- 安装aiohttp库
pip install aiohttp
- 使用aiohttp库下载图片大小
import aiohttp
import asyncio
async def get_image_size(url):
async with aiohttp.ClientSession() as session:
async with session.head(url) as response:
size = response.headers.get('Content-Length', None)
if size:
return int(size)
else:
return None
示例
url = 'https://example.com/image.jpg'
size = asyncio.run(get_image_size(url))
if size:
print(f'图片大小为: {size} 字节')
else:
print('无法获取图片大小')
在上述代码中,我们使用aiohttp.ClientSession()创建一个会话,并通过session.head()方法发送HEAD请求。然后,从响应头中获取Content-Length字段,以确定图片的大小。如果Content-Length字段不存在,则返回None。
四、下载图片并获取大小
在某些情况下,我们可能需要下载图片并获取其大小。以下是使用requests库、urllib库和aiohttp库下载图片并获取大小的示例代码。
使用requests库下载图片并获取大小
import requests
def download_image(url, save_path):
response = requests.get(url, stream=True)
size = response.headers.get('content-length')
if size:
size = int(size)
else:
size = None
with open(save_path, 'wb') as file:
for chunk in response.iter_content(1024):
file.write(chunk)
return size
示例
url = 'https://example.com/image.jpg'
save_path = 'image.jpg'
size = download_image(url, save_path)
if size:
print(f'图片大小为: {size} 字节')
else:
print('无法获取图片大小')
在上述代码中,我们使用requests.get()方法发送GET请求,并通过stream=True参数实现流式下载。然后,将响应内容写入本地文件,并从响应头中获取Content-Length字段,以确定图片的大小。
使用urllib库下载图片并获取大小
import urllib.request
def download_image(url, save_path):
request = urllib.request.Request(url)
with urllib.request.urlopen(request) as response:
size = response.getheader('Content-Length')
if size:
size = int(size)
else:
size = None
with open(save_path, 'wb') as file:
file.write(response.read())
return size
示例
url = 'https://example.com/image.jpg'
save_path = 'image.jpg'
size = download_image(url, save_path)
if size:
print(f'图片大小为: {size} 字节')
else:
print('无法获取图片大小')
在上述代码中,我们使用urllib.request.Request()方法创建一个GET请求,并通过urllib.request.urlopen()方法发送请求。然后,将响应内容写入本地文件,并从响应头中获取Content-Length字段,以确定图片的大小。
使用aiohttp库下载图片并获取大小
import aiohttp
import asyncio
async def download_image(url, save_path):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
size = response.headers.get('Content-Length', None)
if size:
size = int(size)
else:
size = None
with open(save_path, 'wb') as file:
while True:
chunk = await response.content.read(1024)
if not chunk:
break
file.write(chunk)
return size
示例
url = 'https://example.com/image.jpg'
save_path = 'image.jpg'
size = asyncio.run(download_image(url, save_path))
if size:
print(f'图片大小为: {size} 字节')
else:
print('无法获取图片大小')
在上述代码中,我们使用aiohttp.ClientSession()创建一个会话,并通过session.get()方法发送GET请求。然后,将响应内容写入本地文件,并从响应头中获取Content-Length字段,以确定图片的大小。
五、处理图片大小获取失败的情况
在某些情况下,我们可能无法从响应头中获取图片的大小。例如,某些服务器可能不会返回Content-Length字段。在这种情况下,我们可以下载图片并计算其大小。以下是使用requests库、urllib库和aiohttp库下载图片并计算其大小的示例代码。
使用requests库下载图片并计算大小
import requests
def download_image_and_calculate_size(url, save_path):
response = requests.get(url, stream=True)
size = 0
with open(save_path, 'wb') as file:
for chunk in response.iter_content(1024):
file.write(chunk)
size += len(chunk)
return size
示例
url = 'https://example.com/image.jpg'
save_path = 'image.jpg'
size = download_image_and_calculate_size(url, save_path)
print(f'图片大小为: {size} 字节')
在上述代码中,我们使用requests.get()方法发送GET请求,并通过stream=True参数实现流式下载。然后,将响应内容写入本地文件,并计算下载的字节数,以确定图片的大小。
使用urllib库下载图片并计算大小
import urllib.request
def download_image_and_calculate_size(url, save_path):
request = urllib.request.Request(url)
size = 0
with urllib.request.urlopen(request) as response:
with open(save_path, 'wb') as file:
while True:
chunk = response.read(1024)
if not chunk:
break
file.write(chunk)
size += len(chunk)
return size
示例
url = 'https://example.com/image.jpg'
save_path = 'image.jpg'
size = download_image_and_calculate_size(url, save_path)
print(f'图片大小为: {size} 字节')
在上述代码中,我们使用urllib.request.Request()方法创建一个GET请求,并通过urllib.request.urlopen()方法发送请求。然后,将响应内容写入本地文件,并计算下载的字节数,以确定图片的大小。
使用aiohttp库下载图片并计算大小
import aiohttp
import asyncio
async def download_image_and_calculate_size(url, save_path):
size = 0
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
with open(save_path, 'wb') as file:
while True:
chunk = await response.content.read(1024)
if not chunk:
break
file.write(chunk)
size += len(chunk)
return size
示例
url = 'https://example.com/image.jpg'
save_path = 'image.jpg'
size = asyncio.run(download_image_and_calculate_size(url, save_path))
print(f'图片大小为: {size} 字节')
在上述代码中,我们使用aiohttp.ClientSession()创建一个会话,并通过session.get()方法发送GET请求。然后,将响应内容写入本地文件,并计算下载的字节数,以确定图片的大小。
总结
本文详细介绍了如何使用requests库、urllib库和aiohttp库下载网络图片的大小。使用requests库、使用urllib库、使用aiohttp库都是实现这一目标的有效方法。通过这些方法,我们可以先发送HEAD请求获取图片的大小信息,然后再决定是否下载图片。此外,在无法获取图片大小的情况下,我们还可以下载图片并计算其大小。希望本文对您有所帮助,并能解决您在下载网络图片大小时遇到的问题。
相关问答FAQs:
如何使用Python下载特定大小的网络图片?
要下载特定大小的网络图片,您可以使用Python的requests库获取图片,并结合PIL库(Pillow)来检查和调整图片的大小。以下是一个简单的示例代码,您可以根据需要修改目标尺寸:
import requests
from PIL import Image
from io import BytesIO
url = '图片URL'
response = requests.get(url)
img = Image.open(BytesIO(response.content))
# 调整到指定大小
target_size = (宽度, 高度) # 例如 (800, 600)
img = img.resize(target_size)
img.save('downloaded_image.jpg')
如何检查图片下载后的文件大小?
下载图片后,您可以使用os库来检查文件大小。以下是一个示例代码:
import os
file_path = 'downloaded_image.jpg'
file_size = os.path.getsize(file_path)
print(f"文件大小为: {file_size} 字节")
使用Python下载图片时,有哪些常见的错误需要避免?
在下载网络图片时,常见错误包括:
- URL错误:确保提供的图片URL是有效的,并且能够公开访问。
- 网络问题:网络连接不稳定可能导致下载失败,建议添加异常处理代码以应对网络错误。
- 图片格式处理:确保您能够处理各种图片格式,使用PIL库可以轻松地实现格式转换和处理。
通过适当的异常处理和格式检查,您可以提高下载成功的概率。
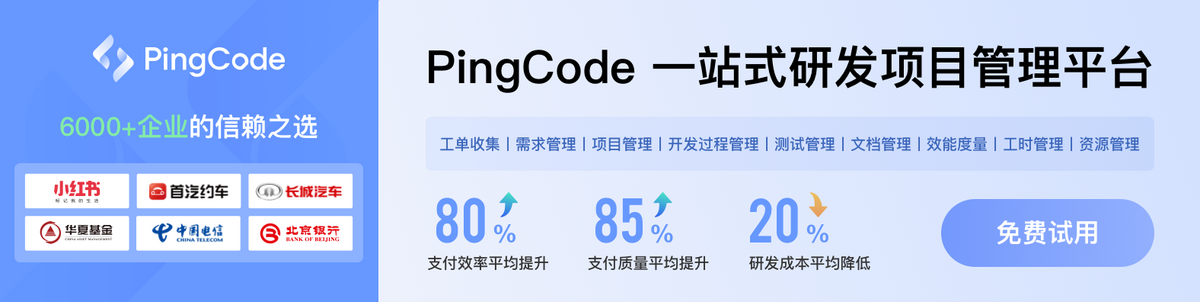