在Python中,你可以通过多种方式来拉取或下载一个文件,例如使用内置的urllib
库、第三方的requests
库、或者通过FTP协议等。这些方法各有优缺点,根据具体需求选择合适的方法。在本文中,我们将详细探讨几种常见的方法,并提供代码示例。
一、使用 urllib
库
Python 内置的 urllib
库提供了简便的方法来处理URL的操作,包括文件下载。
使用 urllib.request.urlretrieve
这是 urllib
中最简单的方法之一。它可以直接将文件下载到本地。
import urllib.request
url = 'http://example.com/somefile.txt'
local_filename = 'somefile.txt'
下载文件
urllib.request.urlretrieve(url, local_filename)
print(f"File downloaded as {local_filename}")
使用 urllib.request.urlopen
如果需要更多的控制,可以使用 urlopen
方法来获取文件内容。
import urllib.request
url = 'http://example.com/somefile.txt'
local_filename = 'somefile.txt'
打开URL并读取内容
response = urllib.request.urlopen(url)
data = response.read()
将内容写入本地文件
with open(local_filename, 'wb') as file:
file.write(data)
print(f"File downloaded as {local_filename}")
二、使用 requests
库
requests
库是一个功能强大的第三方HTTP库,使用起来更加直观和方便。
安装 requests
库
在使用 requests
库之前,需要确保已经安装它。可以使用以下命令安装:
pip install requests
使用 requests.get
这是最常见的下载文件的方法之一。
import requests
url = 'http://example.com/somefile.txt'
local_filename = 'somefile.txt'
发送GET请求
response = requests.get(url)
将内容写入本地文件
with open(local_filename, 'wb') as file:
file.write(response.content)
print(f"File downloaded as {local_filename}")
使用流式下载
对于大文件,可以使用流式下载来节省内存。
import requests
url = 'http://example.com/largefile.zip'
local_filename = 'largefile.zip'
发送GET请求并启用流式下载
response = requests.get(url, stream=True)
将内容写入本地文件
with open(local_filename, 'wb') as file:
for chunk in response.iter_content(chunk_size=1024):
if chunk:
file.write(chunk)
print(f"File downloaded as {local_filename}")
三、使用 FTP 协议
如果文件存储在FTP服务器上,可以使用 ftplib
库来下载文件。
使用 ftplib.FTP
from ftplib import FTP
ftp_server = 'ftp.example.com'
ftp_user = 'username'
ftp_password = 'password'
remote_file_path = '/path/to/remote/file.txt'
local_filename = 'file.txt'
连接到FTP服务器
ftp = FTP(ftp_server)
ftp.login(user=ftp_user, passwd=ftp_password)
打开本地文件以写入
with open(local_filename, 'wb') as file:
# 下载远程文件
ftp.retrbinary(f'RETR {remote_file_path}', file.write)
ftp.quit()
print(f"File downloaded as {local_filename}")
四、使用 SFTP 协议
如果文件存储在SFTP服务器上,可以使用 paramiko
库来下载文件。
安装 paramiko
库
在使用 paramiko
库之前,需要确保已经安装它。可以使用以下命令安装:
pip install paramiko
使用 paramiko
下载文件
import paramiko
hostname = 'sftp.example.com'
port = 22
username = 'username'
password = 'password'
remote_file_path = '/path/to/remote/file.txt'
local_filename = 'file.txt'
创建SSH客户端
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
连接到SFTP服务器
ssh.connect(hostname, port=port, username=username, password=password)
sftp = ssh.open_sftp()
下载文件
sftp.get(remote_file_path, local_filename)
关闭连接
sftp.close()
ssh.close()
print(f"File downloaded as {local_filename}")
五、使用 pandas
库下载数据文件
如果你需要下载并处理CSV或Excel文件,可以使用 pandas
库。
安装 pandas
库
在使用 pandas
库之前,需要确保已经安装它。可以使用以下命令安装:
pip install pandas
使用 pandas.read_csv
和 pandas.read_excel
import pandas as pd
下载CSV文件
csv_url = 'http://example.com/somefile.csv'
csv_data = pd.read_csv(csv_url)
csv_data.to_csv('somefile.csv', index=False)
print(f"CSV file downloaded as somefile.csv")
下载Excel文件
excel_url = 'http://example.com/somefile.xlsx'
excel_data = pd.read_excel(excel_url)
excel_data.to_excel('somefile.xlsx', index=False)
print(f"Excel file downloaded as somefile.xlsx")
六、使用 aiohttp
库实现异步下载
对于需要异步处理的情况,可以使用 aiohttp
库来下载文件。
安装 aiohttp
库
在使用 aiohttp
库之前,需要确保已经安装它。可以使用以下命令安装:
pip install aiohttp
使用 aiohttp
实现异步下载
import aiohttp
import asyncio
async def download_file(url, local_filename):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
with open(local_filename, 'wb') as file:
while True:
chunk = await response.content.read(1024)
if not chunk:
break
file.write(chunk)
print(f"File downloaded as {local_filename}")
url = 'http://example.com/somefile.txt'
local_filename = 'somefile.txt'
运行异步下载任务
asyncio.run(download_file(url, local_filename))
七、使用 gdown
库下载Google Drive文件
如果需要从Google Drive下载文件,可以使用 gdown
库。
安装 gdown
库
在使用 gdown
库之前,需要确保已经安装它。可以使用以下命令安装:
pip install gdown
使用 gdown.download
import gdown
file_id = 'your-google-drive-file-id'
url = f'https://drive.google.com/uc?id={file_id}'
local_filename = 'file_from_drive.txt'
下载文件
gdown.download(url, local_filename, quiet=False)
print(f"File downloaded as {local_filename}")
总结
在Python中,拉取或下载文件的方法多种多样。使用内置的 urllib
库、第三方的 requests
库、FTP或SFTP协议、pandas
库处理数据文件、aiohttp
实现异步下载,以及 gdown
下载Google Drive文件,这些方法都能够满足不同场景下的需求。选择合适的方法将提高你的工作效率,确保下载过程顺利进行。
相关问答FAQs:
如何使用Python从网络上下载文件?
可以使用requests
库来轻松实现文件的下载。首先,确保安装了requests库。然后,使用以下代码示例来下载文件:
import requests
url = 'https://example.com/file.txt' # 替换为实际文件的URL
response = requests.get(url)
with open('file.txt', 'wb') as f:
f.write(response.content)
上述代码将文件下载到当前工作目录,并保存为file.txt
。确保URL正确且文件可访问。
Python中有哪些库可以用于文件下载?
除了requests
库,Python还提供了urllib
和wget
等库用于文件下载。urllib
是Python内置的库,使用示例如下:
import urllib.request
url = 'https://example.com/file.txt'
urllib.request.urlretrieve(url, 'file.txt')
而wget
库需要单独安装,但使用起来也非常简单:
import wget
url = 'https://example.com/file.txt'
wget.download(url)
根据需求选择适合的库来实现文件下载。
如何处理下载文件时的异常情况?
在文件下载过程中,可能会遇到网络问题或URL错误等情况。可以通过捕获异常来处理这些问题,例如:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
with open('file.txt', 'wb') as f:
f.write(response.content)
except requests.exceptions.RequestException as e:
print(f"下载文件时出现错误: {e}")
这样的处理方式可以确保程序在遇到问题时不会崩溃,同时提供错误信息,便于调试。
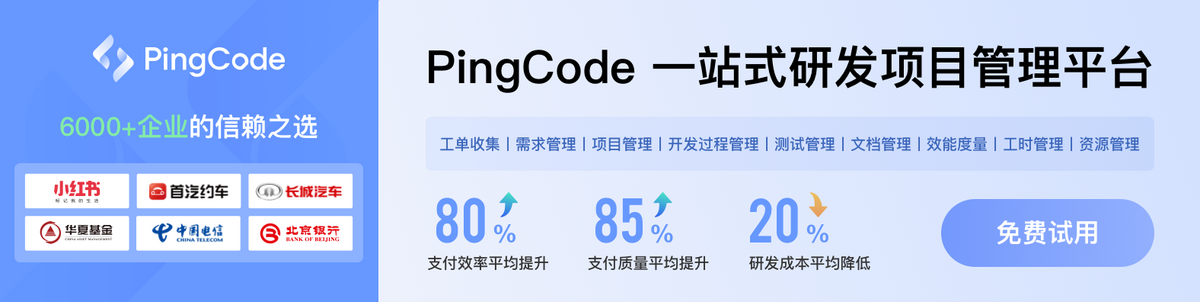