在Python中,给字符串加星号的方法有:使用字符串拼接、使用f-string、使用字符串格式化方法、使用正则表达式。 其中,使用字符串拼接是最常见的一种方法,这种方法简单直观,适合初学者。通过将星号与字符串拼接,可以实现各种不同的效果,例如在字符串的开头、结尾或中间加星号。接下来,我们将详细探讨这些方法。
一、使用字符串拼接
字符串拼接是最基础的操作之一。我们可以使用加号+
将星号与字符串拼接,从而实现字符串加星号的效果。
在字符串前后加星号
s = "hello"
s_with_stars = "*" + s + "*"
print(s_with_stars) # 输出:*hello*
在字符串中间加星号
s = "hello"
s_with_stars = s[:2] + "*" + s[2:]
print(s_with_stars) # 输出:he*llo
在字符串每个字符之间加星号
s = "hello"
s_with_stars = "*".join(s)
print(s_with_stars) # 输出:h*e*l*l*o
二、使用f-string
Python 3.6引入了f-string,通过在字符串前添加f
并在大括号中放置变量或表达式,可以实现字符串的格式化。
在字符串前后加星号
s = "hello"
s_with_stars = f"*{s}*"
print(s_with_stars) # 输出:*hello*
在字符串中间加星号
s = "hello"
s_with_stars = f"{s[:2]}*{s[2:]}"
print(s_with_stars) # 输出:he*llo
三、使用字符串格式化方法
Python提供了多种字符串格式化方法,如str.format()
和%
格式化。
使用str.format()
s = "hello"
s_with_stars = "*{}*".format(s)
print(s_with_stars) # 输出:*hello*
使用%格式化
s = "hello"
s_with_stars = "*%s*" % s
print(s_with_stars) # 输出:*hello*
四、使用正则表达式
正则表达式提供了强大的字符串操作能力,可以用来在字符串中插入星号。
在每个字符之间加星号
import re
s = "hello"
s_with_stars = re.sub(r'(.)', r'\1*', s)[:-1]
print(s_with_stars) # 输出:h*e*l*l*o
在特定位置加星号
import re
s = "hello"
s_with_stars = re.sub(r'(.{2})', r'\1*', s, 1)
print(s_with_stars) # 输出:he*llo
总结
在Python中,给字符串加星号的方法有很多,具体选择哪种方法可以根据实际需求和个人习惯来决定。字符串拼接是最基础且最直观的方法,非常适合初学者。f-string提供了更简洁的写法,适合对代码简洁性有要求的场景。字符串格式化方法提供了更多的格式化选项,适合复杂字符串格式化需求。而正则表达式则提供了强大的字符串操作能力,适合复杂的字符串处理场景。
相关问答FAQs:
如何在Python中为字符串添加星号?
在Python中,可以通过字符串连接或格式化的方式轻松地为字符串添加星号。例如,使用字符串拼接的方法可以将星号直接添加到字符串的前后或中间,示例代码如下:
original_string = "Hello"
modified_string = "*" + original_string + "*"
print(modified_string) # 输出: *Hello*
此外,使用格式化字符串也是一个不错的选择,如下所示:
original_string = "Hello"
modified_string = f"*{original_string}*"
print(modified_string) # 输出: *Hello*
如何在字符串的特定位置添加星号?
如果您想在字符串的特定位置插入星号,可以使用切片操作。例如,想在"HelloWorld"的中间插入星号,可以这样实现:
original_string = "HelloWorld"
position = 5 # 插入位置
modified_string = original_string[:position] + "*" + original_string[position:]
print(modified_string) # 输出: Hello*World
这种方法灵活且易于调整插入的位置。
在Python中如何处理包含多个星号的字符串?
如果需要为字符串中的每个字符添加星号,可以使用循环或列表推导式来实现。例如,以下代码会为"Hello"中的每个字符添加星号:
original_string = "Hello"
modified_string = "*".join(original_string)
modified_string = "*" + modified_string + "*"
print(modified_string) # 输出: *H*e*l*l*o*
这种方法能有效处理并生成一个包含星号的字符串,使其看起来更加特别。
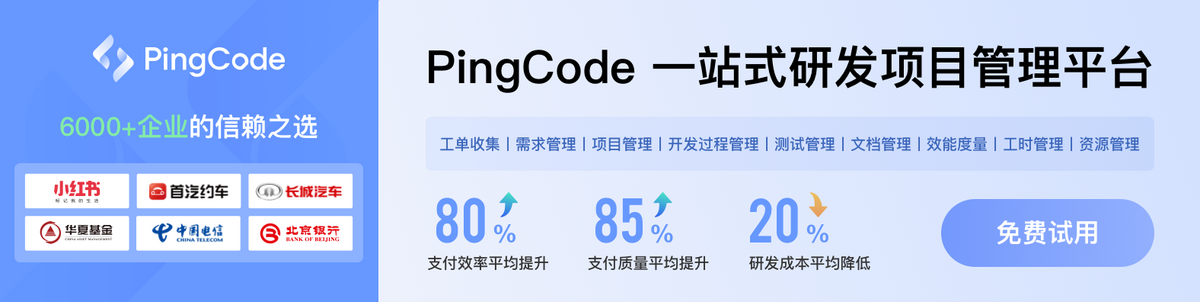