Python获取接口返回值的方法有多种,通常包括使用requests库、http.client库、urllib库等。我们主要推荐使用requests库,因为它简单易用、功能强大。 下面我们将重点详细介绍如何使用requests库获取接口返回值。
一、安装和导入requests库
在开始使用requests库之前,需要先安装它。如果你尚未安装,可以使用以下命令安装requests库:
pip install requests
安装完成后,在你的Python脚本中导入requests库:
import requests
二、发送GET请求
GET请求是最常见的HTTP请求类型之一,用于从服务器获取数据。下面是一个示例,展示如何使用requests库发送GET请求并获取接口返回值:
import requests
发送GET请求
response = requests.get('https://jsonplaceholder.typicode.com/posts/1')
获取返回值(JSON格式)
data = response.json()
print(data)
在上面的示例中,我们发送了一个GET请求到一个示例API,并使用response.json()
方法将返回值解析为Python字典。
注意:确保接口返回的是JSON格式的数据,否则需要根据具体情况解析返回值。
三、发送POST请求
POST请求通常用于向服务器提交数据。下面是一个示例,展示如何使用requests库发送POST请求并获取接口返回值:
import requests
请求头和数据
headers = {'Content-Type': 'application/json'}
data = {'title': 'foo', 'body': 'bar', 'userId': 1}
发送POST请求
response = requests.post('https://jsonplaceholder.typicode.com/posts', headers=headers, json=data)
获取返回值(JSON格式)
response_data = response.json()
print(response_data)
在上面的示例中,我们向一个示例API发送了一个POST请求,并使用response.json()
方法将返回值解析为Python字典。
四、处理其他HTTP方法
除了GET和POST请求,requests库还支持其他HTTP方法,如PUT、DELETE等。下面是一些示例:
PUT请求
PUT请求通常用于更新服务器上的资源:
import requests
请求头和数据
headers = {'Content-Type': 'application/json'}
data = {'id': 1, 'title': 'foo', 'body': 'bar', 'userId': 1}
发送PUT请求
response = requests.put('https://jsonplaceholder.typicode.com/posts/1', headers=headers, json=data)
获取返回值(JSON格式)
response_data = response.json()
print(response_data)
DELETE请求
DELETE请求用于删除服务器上的资源:
import requests
发送DELETE请求
response = requests.delete('https://jsonplaceholder.typicode.com/posts/1')
获取返回值(状态码)
status_code = response.status_code
print(status_code)
五、处理请求异常
在实际使用中,网络请求可能会遇到各种异常情况,如超时、连接错误等。requests库提供了异常处理机制,以下是一些常见的异常处理示例:
import requests
from requests.exceptions import HTTPError, Timeout, RequestException
try:
response = requests.get('https://jsonplaceholder.typicode.com/posts/1', timeout=5)
response.raise_for_status() # 检查是否有HTTP错误
data = response.json()
print(data)
except HTTPError as http_err:
print(f'HTTP error occurred: {http_err}')
except Timeout as timeout_err:
print(f'Timeout error occurred: {timeout_err}')
except RequestException as req_err:
print(f'Request error occurred: {req_err}')
在上面的示例中,我们处理了HTTP错误、超时错误和其他请求错误。
六、解析不同格式的返回值
接口返回的数据格式可能会有所不同,常见的数据格式包括JSON、XML、HTML等。requests库提供了多种方法来解析不同格式的返回值:
JSON格式
对于JSON格式的数据,可以使用response.json()
方法将其解析为Python字典:
import requests
response = requests.get('https://jsonplaceholder.typicode.com/posts/1')
data = response.json()
print(data)
XML格式
对于XML格式的数据,可以使用xml.etree.ElementTree
库进行解析:
import requests
import xml.etree.ElementTree as ET
response = requests.get('https://www.w3schools.com/xml/note.xml')
root = ET.fromstring(response.content)
for child in root:
print(child.tag, child.text)
HTML格式
对于HTML格式的数据,可以使用BeautifulSoup
库进行解析:
import requests
from bs4 import BeautifulSoup
response = requests.get('https://www.example.com')
soup = BeautifulSoup(response.content, 'html.parser')
print(soup.title.text)
七、总结
通过本文的介绍,我们详细讲解了如何使用Python获取接口的返回值。我们重点介绍了requests库的使用,包括发送GET请求、POST请求、PUT请求、DELETE请求等;处理请求异常;解析不同格式的返回值。希望这些内容对你有所帮助,能够在实际开发中灵活运用。
无论是发送请求还是解析返回值,使用requests库都非常方便和简洁,是Python开发者进行HTTP请求的首选工具。
相关问答FAQs:
如何使用Python调用API并获取返回值?
在Python中,调用API通常使用requests
库。您可以使用requests.get()
或requests.post()
方法来发送请求。发送请求后,您可以通过.json()
方法(如果返回的是JSON格式)或.text
方法(如果返回的是文本格式)获取响应内容。以下是一个简单示例:
import requests
response = requests.get('https://api.example.com/data')
if response.status_code == 200:
data = response.json() # 获取JSON格式的返回值
print(data)
else:
print(f"请求失败,状态码:{response.status_code}")
在获取API返回值时如何处理错误或异常?
在进行API请求时,处理可能出现的错误非常重要。您可以使用try
和except
语句来捕获异常。如果请求失败,您可以根据返回的状态码或异常类型进行处理。例如,您可以为常见的HTTP错误代码(如404或500)编写特定的错误处理逻辑。示例代码如下:
import requests
try:
response = requests.get('https://api.example.com/data')
response.raise_for_status() # 检查请求是否成功
data = response.json()
except requests.exceptions.HTTPError as errh:
print(f"HTTP错误:{errh}")
except requests.exceptions.ConnectionError as errc:
print(f"连接错误:{errc}")
except requests.exceptions.Timeout as errt:
print(f"超时错误:{errt}")
except requests.exceptions.RequestException as err:
print(f"请求错误:{err}")
是否可以使用Python处理不同格式的API响应?
确实可以,Python的requests
库支持多种响应格式。对于JSON格式,您可以直接使用.json()
方法获取数据。对于XML格式,可以使用xml.etree.ElementTree
或BeautifulSoup
库进行解析。对于文本数据,可以使用.text
属性直接获取。下面是处理不同格式的示例:
response = requests.get('https://api.example.com/data')
if response.headers['Content-Type'] == 'application/json':
data = response.json()
elif response.headers['Content-Type'] == 'application/xml':
import xml.etree.ElementTree as ET
data = ET.fromstring(response.content)
else:
data = response.text # 处理文本格式
print(data)
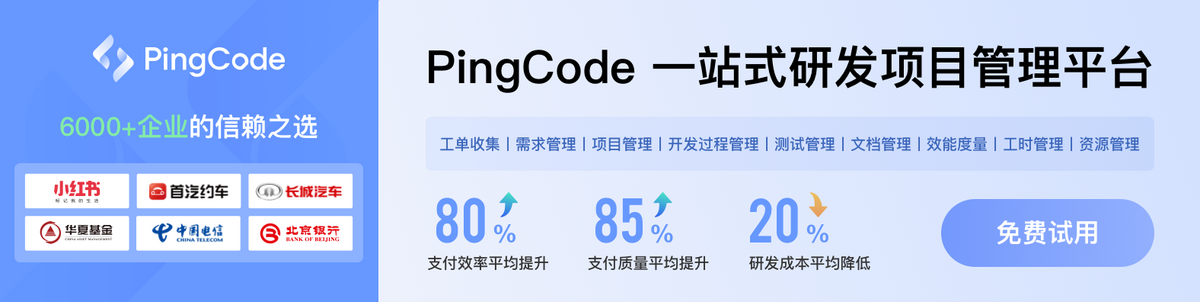