Python查找程序中关键词的方法有:使用字符串方法、使用正则表达式、使用内置模块re、使用第三方库等。 在本文中,我们将重点介绍其中一种方法:使用正则表达式。
一、使用字符串方法查找关键词
字符串方法是一种简单而直接的方法,可以用来查找关键词。Python 提供了一些字符串方法,比如 find()
、index()
和 in
运算符,可以帮助我们在字符串中查找关键词。
1.1 使用 find()
方法
find()
方法返回子字符串在字符串中的最低索引,如果没有找到子字符串,则返回 -1。
text = "This is a sample text for keyword search in Python."
keyword = "keyword"
index = text.find(keyword)
if index != -1:
print(f"Keyword found at index: {index}")
else:
print("Keyword not found.")
1.2 使用 index()
方法
index()
方法与 find()
类似,但如果没有找到子字符串,则会抛出 ValueError
异常。
try:
index = text.index(keyword)
print(f"Keyword found at index: {index}")
except ValueError:
print("Keyword not found.")
1.3 使用 in
运算符
in
运算符可以用来检查子字符串是否存在于字符串中。
if keyword in text:
print("Keyword found.")
else:
print("Keyword not found.")
二、使用正则表达式查找关键词
正则表达式是一种强大而灵活的工具,可以用来匹配字符串中的模式。Python 的 re
模块提供了对正则表达式的支持。
2.1 基本用法
使用 re.search()
方法可以在字符串中查找正则表达式模式。如果找到匹配项,则返回一个匹配对象,否则返回 None
。
import re
text = "This is a sample text for keyword search in Python."
keyword = "keyword"
pattern = re.compile(keyword)
match = pattern.search(text)
if match:
print(f"Keyword found at index: {match.start()}")
else:
print("Keyword not found.")
2.2 查找所有匹配项
使用 re.findall()
方法可以查找字符串中所有匹配的模式,返回一个包含所有匹配项的列表。
matches = pattern.findall(text)
if matches:
print(f"Keyword found {len(matches)} times.")
else:
print("Keyword not found.")
2.3 使用捕获组
捕获组可以用来提取匹配中的特定部分。使用括号 ()
定义捕获组。
pattern = re.compile(r"(key)(word)")
match = pattern.search(text)
if match:
print(f"Full match: {match.group(0)}")
print(f"Group 1: {match.group(1)}")
print(f"Group 2: {match.group(2)}")
else:
print("Keyword not found.")
三、使用内置模块 re 查找关键词
除了上面介绍的基本用法,re
模块还提供了一些高级功能,比如分组、替换和分割等。
3.1 替换字符串中的关键词
使用 re.sub()
方法可以替换字符串中的关键词。
new_text = re.sub(keyword, "replacement", text)
print(new_text)
3.2 分割字符串
使用 re.split()
方法可以根据模式分割字符串。
parts = re.split(r"\s+", text)
print(parts)
四、使用第三方库查找关键词
除了 Python 内置的字符串方法和 re
模块,还有一些第三方库可以用来查找关键词,比如 regex
模块和 pyahocorasick
库。
4.1 使用 regex
模块
regex
模块是 re
模块的一个增强版,提供了一些额外的功能。
import regex
pattern = regex.compile(keyword)
match = pattern.search(text)
if match:
print(f"Keyword found at index: {match.start()}")
else:
print("Keyword not found.")
4.2 使用 pyahocorasick
库
pyahocorasick
是一个快速多模式匹配库,适用于需要在大文本中查找多个关键词的情况。
import ahocorasick
A = ahocorasick.Automaton()
A.add_word(keyword, (0, keyword))
A.make_automaton()
for end_index, (insert_order, original_value) in A.iter(text):
print(f"Keyword '{original_value}' found at index: {end_index - len(original_value) + 1}")
五、总结
在本文中,我们介绍了多种在 Python 程序中查找关键词的方法,包括使用字符串方法、正则表达式、内置模块 re
和第三方库。每种方法都有其优点和适用场景,选择哪种方法取决于具体需求和上下文。
使用字符串方法简单直接,适用于基本的关键词查找;使用正则表达式灵活强大,适用于复杂的模式匹配;使用内置模块 re
提供了高级功能,适用于需要额外处理的情况;使用第三方库如 regex
和 pyahocorasick
则提供了更高的性能和更多的功能,适用于大文本或多关键词的查找。
相关问答FAQs:
如何在Python中实现关键词查找功能?
在Python中,可以使用字符串的内置方法如find()
、index()
或正则表达式模块re
来查找程序中的关键词。通过这些方法,您可以指定要查找的关键词,并在字符串中获取其位置,从而实现简单的关键词查找功能。
在文本文件中如何查找关键词?
要在文本文件中查找关键词,您可以使用Python的文件操作功能。首先,打开文件并读取其内容,然后使用字符串的查找方法或正则表达式来匹配关键词。这种方法适用于搜索大文件中的特定单词或短语。
如何提高关键词查找的效率?
为了提高关键词查找的效率,可以考虑使用更高级的数据结构,如字典或集合来存储关键词。此外,使用多线程或异步编程可以在处理大型文本数据时显著提升性能。选择合适的算法和数据结构是提高查找效率的关键。
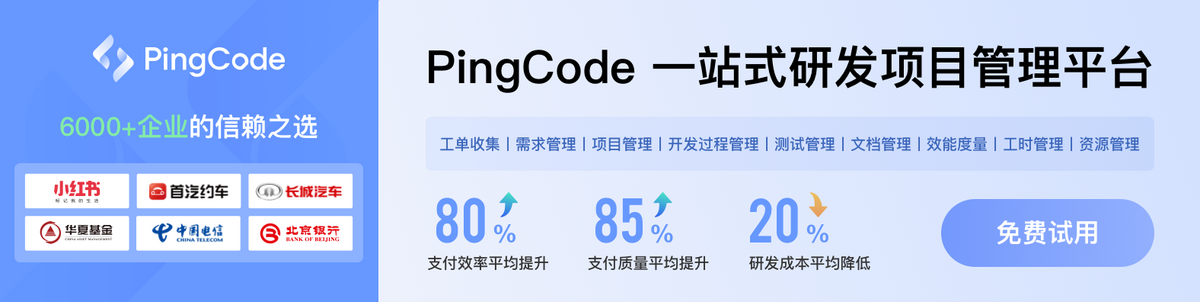