在Python中,我们可以使用变量来代表文件夹的路径,以便于更灵活地进行文件和目录操作。这通常通过字符串变量来实现,该字符串变量存储文件夹的路径。这样可以使代码更加动态和易于维护。使用变量代表文件夹路径的步骤包括:创建变量、赋值文件夹路径、使用os模块操作文件夹。下面详细介绍这些步骤并给出示例代码。
一、创建变量并赋值文件夹路径
首先,我们需要创建一个字符串变量,并将文件夹的路径赋值给该变量。可以使用相对路径或绝对路径,视具体需求而定。
import os
绝对路径
folder_path = "/home/user/documents"
相对路径
relative_folder_path = "./documents"
二、使用os模块操作文件夹
Python的os
模块提供了丰富的函数用于操作文件和目录。通过将文件夹路径赋值给变量,我们可以使用这些函数更灵活地进行操作。
1. 检查文件夹是否存在
if os.path.exists(folder_path):
print(f"The folder '{folder_path}' exists.")
else:
print(f"The folder '{folder_path}' does not exist.")
2. 创建文件夹
if not os.path.exists(folder_path):
os.makedirs(folder_path)
print(f"The folder '{folder_path}' has been created.")
else:
print(f"The folder '{folder_path}' already exists.")
3. 列出文件夹中的所有文件和子文件夹
if os.path.exists(folder_path):
items = os.listdir(folder_path)
print(f"Items in the folder '{folder_path}': {items}")
else:
print(f"The folder '{folder_path}' does not exist.")
4. 删除文件夹
if os.path.exists(folder_path):
os.rmdir(folder_path)
print(f"The folder '{folder_path}' has been deleted.")
else:
print(f"The folder '{folder_path}' does not exist.")
三、使用os.path模块获取更多信息
os.path
模块提供了更多关于文件和目录的信息,比如文件名、扩展名、绝对路径等。
1. 获取绝对路径
absolute_path = os.path.abspath(relative_folder_path)
print(f"The absolute path is: {absolute_path}")
2. 分离文件夹路径和文件名
file_path = "/home/user/documents/file.txt"
folder, file = os.path.split(file_path)
print(f"Folder: {folder}, File: {file}")
3. 获取文件扩展名
file_name = "example.txt"
name, extension = os.path.splitext(file_name)
print(f"File name: {name}, Extension: {extension}")
四、使用pathlib模块
Python 3.4 引入了pathlib
模块,这是一个面向对象的文件系统路径操作模块。相比于os
模块,pathlib
更加直观和易用。
1. 创建Path对象
from pathlib import Path
绝对路径
folder_path = Path("/home/user/documents")
相对路径
relative_folder_path = Path("./documents")
2. 检查文件夹是否存在
if folder_path.exists():
print(f"The folder '{folder_path}' exists.")
else:
print(f"The folder '{folder_path}' does not exist.")
3. 创建文件夹
if not folder_path.exists():
folder_path.mkdir(parents=True, exist_ok=True)
print(f"The folder '{folder_path}' has been created.")
else:
print(f"The folder '{folder_path}' already exists.")
4. 列出文件夹中的所有文件和子文件夹
if folder_path.exists():
items = list(folder_path.iterdir())
print(f"Items in the folder '{folder_path}': {items}")
else:
print(f"The folder '{folder_path}' does not exist.")
5. 删除文件夹
if folder_path.exists():
folder_path.rmdir()
print(f"The folder '{folder_path}' has been deleted.")
else:
print(f"The folder '{folder_path}' does not exist.")
五、使用变量代表文件夹路径的最佳实践
1. 使用常量和环境变量
在项目中,可以通过定义常量或使用环境变量来存储文件夹路径,以便更灵活地管理配置。例如:
import os
使用环境变量
folder_path = os.getenv('DOCUMENTS_FOLDER', '/default/path')
2. 使用配置文件
对于较大的项目,建议使用配置文件来管理文件夹路径。可以使用JSON、YAML等格式的配置文件,并在代码中读取配置文件来获取路径。
import json
读取配置文件
with open('config.json', 'r') as f:
config = json.load(f)
folder_path = config.get('documents_folder', '/default/path')
3. 处理路径中的特殊字符
在路径中可能会包含特殊字符或空格,这时需要特别注意处理。例如:
# 使用原始字符串
folder_path = r"C:\Users\User\My Documents"
或者使用双反斜杠
folder_path = "C:\\Users\\User\\My Documents"
六、示例代码综合应用
下面是一个综合示例,展示了如何使用变量代表文件夹路径,并进行常见的文件夹操作。
import os
from pathlib import Path
定义文件夹路径
folder_path = "/home/user/documents"
检查文件夹是否存在
if os.path.exists(folder_path):
print(f"The folder '{folder_path}' exists.")
else:
print(f"The folder '{folder_path}' does not exist.")
创建文件夹
if not os.path.exists(folder_path):
os.makedirs(folder_path)
print(f"The folder '{folder_path}' has been created.")
else:
print(f"The folder '{folder_path}' already exists.")
列出文件夹中的所有文件和子文件夹
if os.path.exists(folder_path):
items = os.listdir(folder_path)
print(f"Items in the folder '{folder_path}': {items}")
else:
print(f"The folder '{folder_path}' does not exist.")
删除文件夹
if os.path.exists(folder_path):
os.rmdir(folder_path)
print(f"The folder '{folder_path}' has been deleted.")
else:
print(f"The folder '{folder_path}' does not exist.")
使用pathlib模块
folder_path = Path("/home/user/documents")
检查文件夹是否存在
if folder_path.exists():
print(f"The folder '{folder_path}' exists.")
else:
print(f"The folder '{folder_path}' does not exist.")
创建文件夹
if not folder_path.exists():
folder_path.mkdir(parents=True, exist_ok=True)
print(f"The folder '{folder_path}' has been created.")
else:
print(f"The folder '{folder_path}' already exists.")
列出文件夹中的所有文件和子文件夹
if folder_path.exists():
items = list(folder_path.iterdir())
print(f"Items in the folder '{folder_path}': {items}")
else:
print(f"The folder '{folder_path}' does not exist.")
删除文件夹
if folder_path.exists():
folder_path.rmdir()
print(f"The folder '{folder_path}' has been deleted.")
else:
print(f"The folder '{folder_path}' does not exist.")
通过以上示例代码,可以看到如何在Python中使用变量代表文件夹路径,并进行文件夹的创建、检查、列出和删除等操作。通过这种方式,可以使代码更加动态和易于维护。希望这些内容对你有所帮助。
相关问答FAQs:
如何在Python中创建一个代表文件夹的变量?
在Python中,您可以通过简单地将文件夹路径赋值给变量来创建一个代表文件夹的变量。例如,您可以使用以下代码:
folder_path = "C:/Users/YourUsername/Documents/MyFolder"
这样,folder_path
变量就可以用于后续的文件操作,如创建、删除或访问该文件夹。
如何使用Python中的变量动态访问文件夹内容?
您可以使用os
模块来动态访问文件夹中的内容。通过将文件夹路径存储在变量中,您可以轻松列出该目录中的所有文件和子文件夹。以下是一个示例代码:
import os
folder_path = "C:/Users/YourUsername/Documents/MyFolder"
files = os.listdir(folder_path)
print(files)
这段代码将输出指定文件夹内的所有文件和文件夹名称。
在Python中,如何使用变量代表文件夹路径进行文件操作?
使用变量代表文件夹路径后,您可以执行多种文件操作,如创建新文件、删除文件或移动文件。以下是一个创建新文件的示例:
folder_path = "C:/Users/YourUsername/Documents/MyFolder"
file_path = os.path.join(folder_path, "new_file.txt")
with open(file_path, 'w') as file:
file.write("Hello, World!")
这段代码将在指定的文件夹内创建一个名为new_file.txt
的新文件,并写入内容。
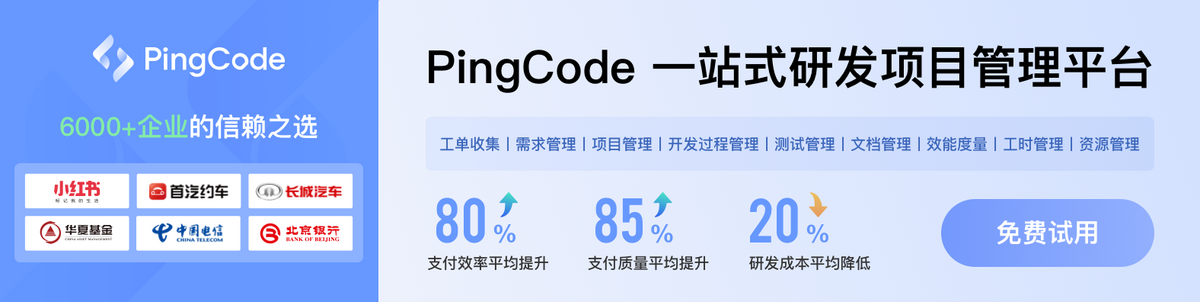