在Python中,可以通过多种方式将一个变量拆开,包括字符串拆分、列表拆分、字典拆分等。常用的方法有切片、split()方法、正则表达式、解包等。其中,字符串拆分是最常见的操作,我们可以使用split()方法将一个字符串按指定分隔符拆分成一个列表。下面将详细介绍几种常见的拆分方法。
一、字符串拆分
1. 使用split()方法
split()方法是Python中用于字符串拆分的最常用方法。它可以按照指定的分隔符将字符串拆分成多个子字符串,并返回一个列表。
text = "Hello,world,Python"
split_text = text.split(',')
print(split_text)
输出:
['Hello', 'world', 'Python']
2. 使用正则表达式
正则表达式可以更灵活地匹配和拆分字符串。Python的re模块提供了多种正则表达式操作方法。
import re
text = "Hello123world456Python"
split_text = re.split(r'\d+', text)
print(split_text)
输出:
['Hello', 'world', 'Python']
3. 字符串切片
字符串切片可以根据索引位置将字符串拆分成多个部分。
text = "HelloWorld"
part1 = text[:5]
part2 = text[5:]
print(part1, part2)
输出:
Hello World
二、列表拆分
1. 使用切片
列表切片是将一个列表拆分成多个子列表的常用方法。
numbers = [1, 2, 3, 4, 5, 6]
part1 = numbers[:3]
part2 = numbers[3:]
print(part1, part2)
输出:
[1, 2, 3] [4, 5, 6]
2. 使用列表解析
列表解析可以根据条件将列表拆分成多个子列表。
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = [x for x in numbers if x % 2 == 0]
odd_numbers = [x for x in numbers if x % 2 != 0]
print(even_numbers, odd_numbers)
输出:
[2, 4, 6] [1, 3, 5]
三、字典拆分
1. 按键拆分
可以根据字典的键将字典拆分成多个子字典。
data = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
keys_part1 = ['a', 'b']
keys_part2 = ['c', 'd']
dict_part1 = {k: data[k] for k in keys_part1}
dict_part2 = {k: data[k] for k in keys_part2}
print(dict_part1, dict_part2)
输出:
{'a': 1, 'b': 2} {'c': 3, 'd': 4}
2. 按值拆分
可以根据字典的值将字典拆分成多个子字典。
data = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
dict_part1 = {k: v for k, v in data.items() if v <= 2}
dict_part2 = {k: v for k, v in data.items() if v > 2}
print(dict_part1, dict_part2)
输出:
{'a': 1, 'b': 2} {'c': 3, 'd': 4}
四、元组拆分
1. 使用切片
元组切片可以根据索引位置将元组拆分成多个部分。
numbers = (1, 2, 3, 4, 5, 6)
part1 = numbers[:3]
part2 = numbers[3:]
print(part1, part2)
输出:
(1, 2, 3) (4, 5, 6)
2. 使用解包
解包可以将元组拆分成多个变量。
numbers = (1, 2, 3, 4)
a, b, c, d = numbers
print(a, b, c, d)
输出:
1 2 3 4
五、数据框拆分
在数据分析中,经常需要将一个数据框拆分成多个子数据框。可以使用pandas库进行数据框拆分。
import pandas as pd
data = {'A': [1, 2, 3, 4], 'B': [5, 6, 7, 8]}
df = pd.DataFrame(data)
按行拆分
df_part1 = df.iloc[:2]
df_part2 = df.iloc[2:]
按列拆分
df_part3 = df[['A']]
df_part4 = df[['B']]
print(df_part1)
print(df_part2)
print(df_part3)
print(df_part4)
输出:
A B
0 1 5
1 2 6
A B
2 3 7
3 4 8
A
0 1
1 2
2 3
3 4
B
0 5
1 6
2 7
3 8
六、函数拆分
有时需要将一个复杂的函数拆分成多个小函数,以提高代码的可读性和可维护性。
def complex_function(x):
result = step1(x)
result = step2(result)
result = step3(result)
return result
def step1(x):
return x + 1
def step2(x):
return x * 2
def step3(x):
return x - 3
print(complex_function(5))
输出:
9
七、文件拆分
在处理大文件时,可能需要将一个大文件拆分成多个小文件。可以使用Python内置的文件操作函数进行文件拆分。
input_file = 'large_file.txt'
output_file1 = 'part1.txt'
output_file2 = 'part2.txt'
with open(input_file, 'r') as infile:
lines = infile.readlines()
half = len(lines) // 2
with open(output_file1, 'w') as outfile1:
outfile1.writelines(lines[:half])
with open(output_file2, 'w') as outfile2:
outfile2.writelines(lines[half:])
八、图像拆分
在计算机视觉中,可能需要将一个大图像拆分成多个小图像。可以使用PIL库进行图像拆分。
from PIL import Image
image = Image.open('large_image.jpg')
width, height = image.size
part1 = image.crop((0, 0, width // 2, height))
part2 = image.crop((width // 2, 0, width, height))
part1.save('part1.jpg')
part2.save('part2.jpg')
九、数据集拆分
在机器学习中,经常需要将数据集拆分成训练集和测试集。可以使用scikit-learn库进行数据集拆分。
from sklearn.model_selection import train_test_split
X = [[1, 2], [3, 4], [5, 6], [7, 8]]
y = [0, 1, 0, 1]
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.25, random_state=42)
print(X_train, X_test)
print(y_train, y_test)
输出:
[[3, 4], [1, 2], [7, 8]] [[5, 6]]
[1, 0, 1] [0]
十、字符串格式化拆分
有时需要将一个格式化的字符串拆分成多个部分。可以使用字符串的format()方法进行格式化拆分。
text = "Hello, {name}. Welcome to {place}."
formatted_text = text.format(name="Alice", place="Wonderland")
print(formatted_text)
输出:
Hello, Alice. Welcome to Wonderland.
总结:在Python中,可以通过多种方式将一个变量拆开,包括字符串拆分、列表拆分、字典拆分、元组拆分、数据框拆分、函数拆分、文件拆分、图像拆分、数据集拆分和字符串格式化拆分。每种方法都有其适用的场景和优缺点,选择合适的方法可以提高代码的效率和可读性。
相关问答FAQs:
如何在Python中将一个变量的值拆分成多个部分?
在Python中,可以使用字符串的拆分功能,将一个变量的值拆分为多个部分。例如,使用split()
方法可以根据特定的分隔符将字符串拆分成列表。假设变量data
包含一个字符串"apple,banana,cherry",可以通过data.split(',')
将其拆分为['apple', 'banana', 'cherry']
。
在Python中,如何将列表中的元素单独提取为变量?
如果有一个列表,比如fruits = ['apple', 'banana', 'cherry']
,可以使用解包的方法将其元素提取到多个变量中。例如,使用语法a, b, c = fruits
,就能将fruits
列表中的每个元素分别赋值给变量a
、b
和c
。
Python可以如何处理字典变量以提取特定的键值对?
对于字典变量,比如my_dict = {'name': 'Alice', 'age': 30, 'city': 'New York'}
,可以通过键来直接访问特定的值。例如,使用my_dict['name']
可以获取值'Alice'
。如果需要将字典中的多个键值对拆分到不同的变量中,可以使用多重赋值,例如name, age = my_dict['name'], my_dict['age']
。
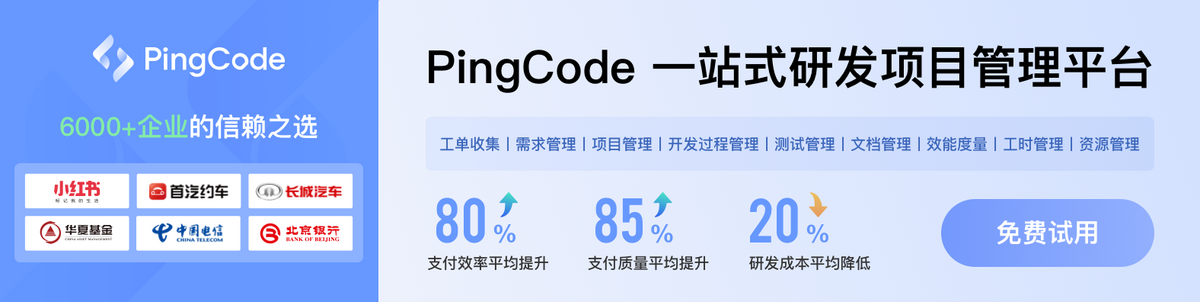