使用Python替换文件中的某一行时,可以通过读取文件内容到内存中,修改目标行,然后将修改后的内容写回文件。步骤包括读取文件、处理数据、写回文件。下面将详细描述其中一个步骤。
具体操作步骤如下:
- 读取文件内容并存储到列表中。
- 找到并替换目标行。
- 将修改后的内容写回文件。
这三步操作能够确保文件内容得以正确的修改并保存。下面将详细介绍每一个步骤。
一、读取文件内容并存储到列表中
首先,我们需要将文件内容读取到内存中。这样我们可以对内容进行处理。可以使用 readlines()
方法将文件内容读入到一个列表中,每一行作为列表中的一个元素。
with open('filename.txt', 'r') as file:
lines = file.readlines()
二、找到并替换目标行
在读取完文件内容后,我们需要遍历列表找到需要替换的目标行,并用新的内容替换它。假设我们要替换第三行,可以直接通过列表索引来进行替换。
target_line_index = 2 # 索引从0开始,第三行的索引是2
new_content = "This is the new content for the third line.\n"
lines[target_line_index] = new_content
如果需要根据某些条件来替换行,比如包含特定字符串的行,则可以使用循环和条件判断来实现。
search_string = "old content"
new_content = "This is the new content.\n"
for i, line in enumerate(lines):
if search_string in line:
lines[i] = new_content
break
三、将修改后的内容写回文件
最后一步是将修改后的内容写回文件。可以使用 writelines()
方法将列表内容写入文件。这会覆盖原文件内容。
with open('filename.txt', 'w') as file:
file.writelines(lines)
示例代码
将上述步骤整合起来,形成一个完整的示例代码:
def replace_line_in_file(filename, target_line_index, new_content):
# 读取文件内容
with open(filename, 'r') as file:
lines = file.readlines()
# 替换目标行
if 0 <= target_line_index < len(lines):
lines[target_line_index] = new_content + '\n'
# 将修改后的内容写回文件
with open(filename, 'w') as file:
file.writelines(lines)
示例调用
replace_line_in_file('example.txt', 2, "This is the new content for the third line.")
四、根据条件替换行
有时,替换操作需要根据某些条件来执行,比如替换包含特定字符串的行。下面是一个根据条件替换行的示例:
def replace_line_in_file_by_condition(filename, search_string, new_content):
# 读取文件内容
with open(filename, 'r') as file:
lines = file.readlines()
# 查找并替换符合条件的行
for i, line in enumerate(lines):
if search_string in line:
lines[i] = new_content + '\n'
break
# 将修改后的内容写回文件
with open(filename, 'w') as file:
file.writelines(lines)
示例调用
replace_line_in_file_by_condition('example.txt', "old content", "This is the new content.")
这种方法可以更灵活地处理文件内容的替换操作。
五、处理大文件
如果文件非常大,读取整个文件到内存中可能会导致内存不足。此时可以采用逐行读取和写入的方式。在这种情况下,可以使用临时文件来存储修改后的内容,然后再替换原文件。
import os
def replace_line_in_large_file(filename, search_string, new_content):
temp_filename = filename + '.tmp'
with open(filename, 'r') as file, open(temp_filename, 'w') as temp_file:
for line in file:
if search_string in line:
temp_file.write(new_content + '\n')
else:
temp_file.write(line)
os.replace(temp_filename, filename)
示例调用
replace_line_in_large_file('large_example.txt', "old content", "This is the new content.")
这种方法更适合处理大文件,因为它避免了将整个文件内容加载到内存中。
总结
通过以上步骤,我们可以使用Python替换文件中的某一行。总结起来,主要步骤包括读取文件内容、找到并替换目标行、将修改后的内容写回文件。对于大文件,可以采用临时文件来避免内存不足的问题。通过这些方法,可以方便地实现文件内容的修改操作。
相关问答FAQs:
如何使用Python替换文件中的特定行?
要替换文件中的特定行,您可以使用Python的文件读写功能。首先,读取文件的所有行并将其存储在列表中,然后修改您想替换的行,最后将所有行写回到文件中。例如,您可以使用open()
函数打开文件并使用readlines()
方法读取所有行。在进行修改后,使用writelines()
方法将更改写入文件。
在Python中替换文件行时需要注意哪些事项?
在替换文件行时,确保您有适当的权限来读取和写入文件。此外,处理文件时要注意文件的编码格式,确保读取和写入时使用相同的编码,以避免出现乱码或数据丢失。建议在进行文件操作之前备份原始文件,以防在修改过程中发生意外问题。
使用Python替换文件行的最佳实践是什么?
最佳实践包括使用with
语句来打开文件,以确保文件在操作完成后自动关闭,这可以避免文件泄露或其他资源问题。同时,建议在进行行替换时,使用异常处理机制来捕获潜在的错误,例如文件未找到或权限错误。这将提高代码的健壮性和可维护性。
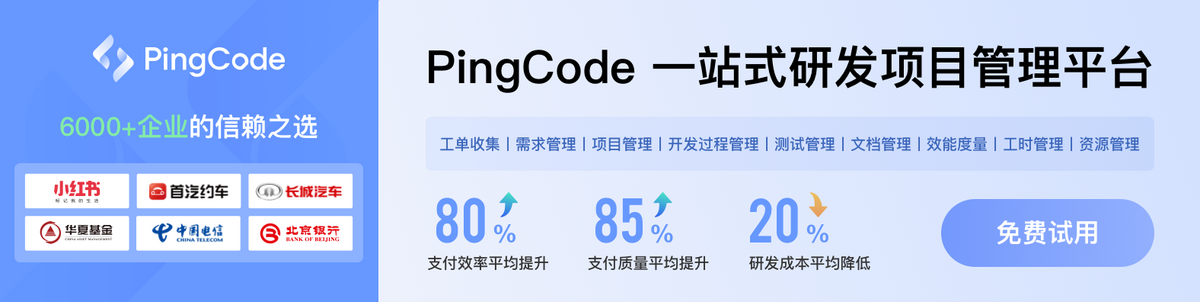