要用Python备份文件格式,可以使用shutil模块、os模块、pathlib模块、或者第三方库如boto3、dropbox等进行本地或者云端备份。 其中,使用shutil模块来复制文件是最简单直接的方法。shutil模块提供了高层次的文件操作功能,比如复制、移动、压缩文件和目录等。接下来,我们详细描述如何使用shutil模块来备份文件。
一、SHUTIL模块备份文件
shutil模块提供了许多高层次的文件操作功能,包括复制文件、移动文件、删除文件等。要备份文件,可以使用shutil.copy()或shutil.copy2()。
1、复制单个文件
shutil.copy()函数用于复制单个文件。它接受两个参数:源文件路径和目标文件路径。
import shutil
def backup_file(source, destination):
try:
shutil.copy(source, destination)
print(f"Backup of {source} to {destination} completed successfully.")
except Exception as e:
print(f"Error occurred while backing up file: {e}")
source_file = 'path/to/source/file.txt'
destination_file = 'path/to/destination/file_backup.txt'
backup_file(source_file, destination_file)
注意: shutil.copy2()
类似于shutil.copy()
,但它会尽量保留原文件的元数据(如时间戳)。
2、复制整个目录
shutil.copytree()函数可以用来复制整个目录。它接受两个参数:源目录路径和目标目录路径。
import shutil
def backup_directory(source_dir, destination_dir):
try:
shutil.copytree(source_dir, destination_dir)
print(f"Backup of {source_dir} to {destination_dir} completed successfully.")
except Exception as e:
print(f"Error occurred while backing up directory: {e}")
source_directory = 'path/to/source/directory'
destination_directory = 'path/to/destination/directory_backup'
backup_directory(source_directory, destination_directory)
二、使用OS模块进行文件备份
os模块提供了与操作系统进行交互的功能。尽管它的文件操作功能没有shutil模块丰富,但它可以与shutil模块结合使用。
1、检查文件是否存在
在备份文件之前,检查源文件是否存在是一个好习惯。
import os
import shutil
def backup_file(source, destination):
if os.path.isfile(source):
try:
shutil.copy(source, destination)
print(f"Backup of {source} to {destination} completed successfully.")
except Exception as e:
print(f"Error occurred while backing up file: {e}")
else:
print(f"Source file {source} does not exist.")
source_file = 'path/to/source/file.txt'
destination_file = 'path/to/destination/file_backup.txt'
backup_file(source_file, destination_file)
2、创建目标目录
在备份目录之前,确保目标目录存在。如果目标目录不存在,可以使用os.makedirs()函数来创建。
import os
import shutil
def backup_directory(source_dir, destination_dir):
if os.path.isdir(source_dir):
if not os.path.exists(destination_dir):
os.makedirs(destination_dir)
try:
shutil.copytree(source_dir, destination_dir)
print(f"Backup of {source_dir} to {destination_dir} completed successfully.")
except Exception as e:
print(f"Error occurred while backing up directory: {e}")
else:
print(f"Source directory {source_dir} does not exist.")
source_directory = 'path/to/source/directory'
destination_directory = 'path/to/destination/directory_backup'
backup_directory(source_directory, destination_directory)
三、使用Pathlib模块进行文件备份
pathlib模块提供了一种面向对象的方式来处理文件和目录路径。它使得代码更加简洁和易读。
1、使用Pathlib复制单个文件
from pathlib import Path
import shutil
def backup_file(source, destination):
source_path = Path(source)
destination_path = Path(destination)
if source_path.is_file():
try:
shutil.copy(source_path, destination_path)
print(f"Backup of {source} to {destination} completed successfully.")
except Exception as e:
print(f"Error occurred while backing up file: {e}")
else:
print(f"Source file {source} does not exist.")
source_file = 'path/to/source/file.txt'
destination_file = 'path/to/destination/file_backup.txt'
backup_file(source_file, destination_file)
2、使用Pathlib复制整个目录
from pathlib import Path
import shutil
def backup_directory(source_dir, destination_dir):
source_path = Path(source_dir)
destination_path = Path(destination_dir)
if source_path.is_dir():
if not destination_path.exists():
destination_path.mkdir(parents=True)
try:
shutil.copytree(source_path, destination_path)
print(f"Backup of {source_dir} to {destination_dir} completed successfully.")
except Exception as e:
print(f"Error occurred while backing up directory: {e}")
else:
print(f"Source directory {source_dir} does not exist.")
source_directory = 'path/to/source/directory'
destination_directory = 'path/to/destination/directory_backup'
backup_directory(source_directory, destination_directory)
四、使用第三方库进行云端备份
如果需要将文件备份到云端,可以使用第三方库,如boto3(用于AWS S3)或dropbox(用于Dropbox)。
1、使用boto3备份到AWS S3
安装boto3库:
pip install boto3
使用boto3将文件上传到S3:
import boto3
from botocore.exceptions import NoCredentialsError
def backup_to_s3(source, bucket_name, destination):
s3 = boto3.client('s3')
try:
s3.upload_file(source, bucket_name, destination)
print(f"Backup of {source} to s3://{bucket_name}/{destination} completed successfully.")
except FileNotFoundError:
print(f"Source file {source} does not exist.")
except NoCredentialsError:
print("Credentials not available.")
except Exception as e:
print(f"Error occurred while backing up file: {e}")
source_file = 'path/to/source/file.txt'
bucket_name = 'your-s3-bucket'
destination_file = 'file_backup.txt'
backup_to_s3(source_file, bucket_name, destination_file)
2、使用Dropbox API备份文件
安装dropbox库:
pip install dropbox
使用dropbox库将文件上传到Dropbox:
import dropbox
def backup_to_dropbox(source, access_token, destination):
dbx = dropbox.Dropbox(access_token)
try:
with open(source, 'rb') as f:
dbx.files_upload(f.read(), destination)
print(f"Backup of {source} to Dropbox at {destination} completed successfully.")
except FileNotFoundError:
print(f"Source file {source} does not exist.")
except dropbox.exceptions.ApiError as err:
print(f"API error: {err}")
except Exception as e:
print(f"Error occurred while backing up file: {e}")
source_file = 'path/to/source/file.txt'
access_token = 'your-dropbox-access-token'
destination_file = '/file_backup.txt'
backup_to_dropbox(source_file, access_token, destination_file)
五、定时备份
为了确保数据定期备份,可以使用调度库如schedule
或APScheduler
来定时执行备份任务。
1、使用schedule库定时备份
安装schedule库:
pip install schedule
import schedule
import time
import shutil
def backup_file():
source = 'path/to/source/file.txt'
destination = 'path/to/destination/file_backup.txt'
try:
shutil.copy(source, destination)
print(f"Backup of {source} to {destination} completed successfully.")
except Exception as e:
print(f"Error occurred while backing up file: {e}")
Schedule the backup to run every day at 2:00 AM
schedule.every().day.at("02:00").do(backup_file)
while True:
schedule.run_pending()
time.sleep(1)
2、使用APScheduler定时备份
安装APScheduler库:
pip install apscheduler
from apscheduler.schedulers.blocking import BlockingScheduler
import shutil
def backup_file():
source = 'path/to/source/file.txt'
destination = 'path/to/destination/file_backup.txt'
try:
shutil.copy(source, destination)
print(f"Backup of {source} to {destination} completed successfully.")
except Exception as e:
print(f"Error occurred while backing up file: {e}")
scheduler = BlockingScheduler()
Schedule the backup to run every day at 2:00 AM
scheduler.add_job(backup_file, 'cron', hour=2, minute=0)
try:
scheduler.start()
except (KeyboardInterrupt, SystemExit):
pass
总结
通过使用Python中的shutil、os、pathlib模块,我们可以轻松地进行文件和目录的本地备份。同时,借助第三方库如boto3、dropbox,我们还可以将文件备份到云端。此外,利用调度库如schedule和APScheduler,我们可以定时自动执行备份任务。选择适合自己需求的方式,能够确保数据安全、备份及时。
相关问答FAQs:
如何使用Python进行文件备份?
使用Python进行文件备份通常涉及到文件的复制。可以使用内置的shutil
模块,通过shutil.copy()
或shutil.copytree()
函数来实现文件或目录的备份。此外,还可以结合os
模块来处理文件路径和检查文件是否存在。备份的过程可以简单地编写为一个脚本,定时运行或手动执行。
在Python中备份文件时,应该考虑哪些因素?
在备份文件时,考虑以下因素是非常重要的:首先,确认备份的文件是否重要并且需要定期备份;其次,选择合适的存储位置,如外部硬盘或云存储,以确保数据的安全;最后,制定一个备份策略,比如定期自动备份,以防止数据丢失。
如何实现自动化备份,Python中有哪些工具可以使用?
自动化备份可以通过设置定时任务来实现。在Python中,可以使用schedule
库来创建定时任务,它允许你设定特定时间间隔进行备份。此外,结合cron
(Linux)或任务计划程序(Windows)可以实现更复杂的备份策略,确保备份操作在指定时间自动执行。
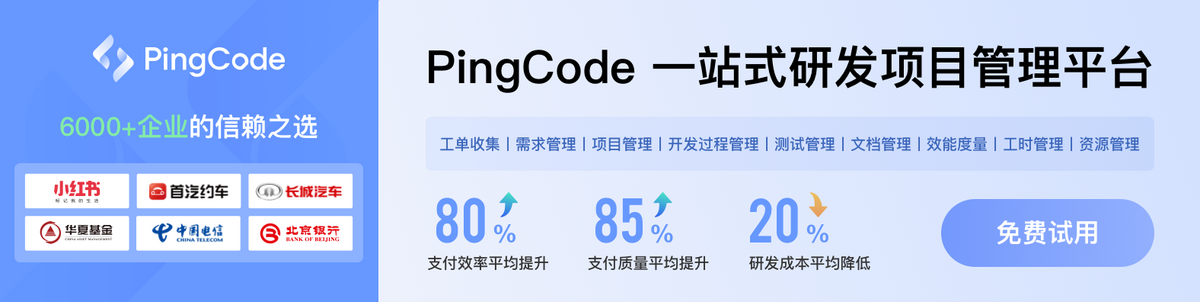