要将数据传到云端,可以使用云存储服务(例如AWS S3、Google Cloud Storage、Azure Blob Storage)、REST API、数据库服务(例如Google Firestore、AWS RDS)、使用Python库(例如boto3、google-cloud-storage、pyodbc)。下面详细介绍如何使用AWS S3和Google Cloud Storage存储数据。
一、AWS S3
AWS S3(Amazon Simple Storage Service)是一个广泛使用的云存储服务。以下是使用Python将数据上传到AWS S3的步骤。
1. 安装boto3库
boto3是AWS SDK for Python,提供与AWS服务集成的接口。
pip install boto3
2. 配置AWS凭证
在你的系统上设置AWS凭证。AWS凭证可以通过AWS管理控制台创建。
aws configure
3. 编写Python代码
以下是一个示例代码,将本地文件上传到S3桶中。
import boto3
from botocore.exceptions import NoCredentialsError
def upload_to_aws(local_file, bucket, s3_file):
s3 = boto3.client('s3')
try:
s3.upload_file(local_file, bucket, s3_file)
print("Upload Successful")
return True
except FileNotFoundError:
print("The file was not found")
return False
except NoCredentialsError:
print("Credentials not available")
return False
uploaded = upload_to_aws('local_file.txt', 'my_bucket', 's3_file.txt')
详细描述:
在这段代码中,我们使用了boto3库来创建一个S3客户端。然后,我们使用upload_file
方法将本地文件上传到指定的S3桶中。如果文件上传成功,返回True,否则捕获异常并返回False。
二、Google Cloud Storage
Google Cloud Storage是Google Cloud Platform提供的对象存储服务。以下是使用Python将数据上传到Google Cloud Storage的步骤。
1. 安装google-cloud-storage库
pip install google-cloud-storage
2. 配置Google Cloud身份验证
在Google Cloud控制台中创建服务账号,并下载JSON格式的密钥文件。设置GOOGLE_APPLICATION_CREDENTIALS
环境变量以指向密钥文件。
export GOOGLE_APPLICATION_CREDENTIALS="/path/to/keyfile.json"
3. 编写Python代码
以下是一个示例代码,将本地文件上传到Google Cloud Storage桶中。
from google.cloud import storage
def upload_to_gcs(bucket_name, source_file_name, destination_blob_name):
storage_client = storage.Client()
bucket = storage_client.bucket(bucket_name)
blob = bucket.blob(destination_blob_name)
blob.upload_from_filename(source_file_name)
print(f"File {source_file_name} uploaded to {destination_blob_name}.")
upload_to_gcs('my_bucket', 'local_file.txt', 'gcs_file.txt')
详细描述:
在这段代码中,我们使用了google-cloud-storage库来创建一个存储客户端。然后,我们获取指定的桶,并创建一个Blob对象,使用upload_from_filename
方法将本地文件上传到指定的桶中。
三、使用REST API
许多云服务提供REST API接口,可以通过HTTP请求上传数据。以下是如何使用Python的requests库进行POST请求上传数据的示例。
1. 安装requests库
pip install requests
2. 编写Python代码
以下是一个示例代码,使用POST请求将数据上传到示例REST API。
import requests
url = 'https://example.com/upload'
files = {'file': open('local_file.txt', 'rb')}
response = requests.post(url, files=files)
if response.status_code == 200:
print("Upload Successful")
else:
print("Upload Failed")
详细描述:
在这段代码中,我们使用了requests库发送一个POST请求。我们将本地文件作为二进制文件打开,并将其包含在请求的files参数中。如果服务器响应状态码为200,表示上传成功。
四、使用数据库服务
可以使用Python连接到云数据库,并将数据插入数据库表中。以下是如何使用pyodbc将数据插入到Azure SQL数据库的示例。
1. 安装pyodbc库
pip install pyodbc
2. 配置数据库连接
你需要Azure SQL数据库的连接字符串。
3. 编写Python代码
以下是一个示例代码,使用pyodbc将数据插入到Azure SQL数据库表中。
import pyodbc
def insert_to_azure_sql(server, database, username, password, table, data):
connection_string = f'DRIVER={{ODBC Driver 17 for SQL Server}};SERVER={server};DATABASE={database};UID={username};PWD={password}'
connection = pyodbc.connect(connection_string)
cursor = connection.cursor()
insert_query = f'INSERT INTO {table} (column1, column2) VALUES (?, ?)'
cursor.execute(insert_query, data[0], data[1])
connection.commit()
cursor.close()
connection.close()
print("Data inserted successfully")
server = 'your_server.database.windows.net'
database = 'your_database'
username = 'your_username'
password = 'your_password'
table = 'your_table'
data = ('value1', 'value2')
insert_to_azure_sql(server, database, username, password, table, data)
详细描述:
在这段代码中,我们使用pyodbc库连接到Azure SQL数据库。我们创建一个连接对象和一个游标对象,然后执行插入语句将数据插入到指定的表中,最后提交事务并关闭连接。
通过这些方法,可以方便地将数据上传到云端。根据具体需求选择适合的云存储服务和方法,确保数据的安全性和可靠性。
相关问答FAQs:
如何选择合适的云服务提供商进行数据传输?
在选择云服务提供商时,需要考虑多个因素,如数据安全性、传输速度、存储成本和可扩展性。常见的云服务提供商包括AWS、Google Cloud和Microsoft Azure。建议根据项目的具体需求进行评估,查看其提供的API文档和数据传输工具。
在Python中如何使用API将数据上传到云端?
使用Python上传数据到云端,通常可以通过调用云服务的API实现。可以使用库如requests
来发送HTTP请求,或使用专用的SDK(例如boto3
用于AWS)来简化操作。在上传数据前,需要进行身份验证,并根据API文档设置相应的请求头和数据格式。
如何保证数据在传输过程中的安全性?
为了确保数据在传输过程中的安全性,可以采用SSL/TLS加密技术,这样数据在传输时会被加密。此外,使用JWT(JSON Web Token)或OAuth进行身份验证可以增加安全性。还可以考虑使用数据加密库(如cryptography
)在传输前对数据进行加密。
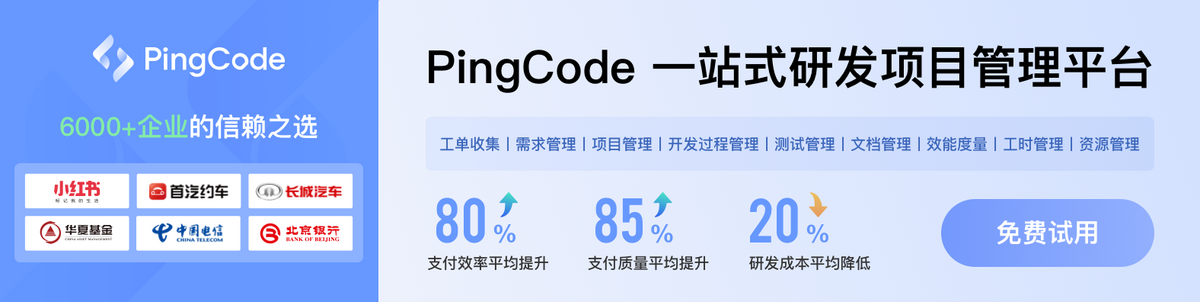