Python修改txt文件中的内容可以通过读取文件内容、对内容进行修改、然后将修改后的内容写回文件中实现。常用的方法包括逐行读取和写入、直接读取整个文件内容到内存中进行操作、使用正则表达式进行复杂的文本替换。具体实现方法可能因需求不同而有所差异。下面将详细介绍几种常见的实现方法。
一、逐行读取和写入
逐行读取和写入文件是最基本的方法之一。这种方法适用于文件内容较大且修改内容相对较少的情况。其基本步骤包括打开源文件和目标文件、逐行读取源文件内容并进行修改、将修改后的内容写入目标文件。
def modify_file_line_by_line(input_file, output_file, target_str, replacement_str):
with open(input_file, 'r') as file:
lines = file.readlines()
with open(output_file, 'w') as file:
for line in lines:
modified_line = line.replace(target_str, replacement_str)
file.write(modified_line)
Usage
modify_file_line_by_line('input.txt', 'output.txt', 'old_text', 'new_text')
在上述代码中,modify_file_line_by_line
函数通过readlines()
方法逐行读取文件内容,并在每行中使用replace
方法将目标字符串替换为新的字符串,最后将修改后的内容写入目标文件。
二、直接读取整个文件内容到内存中进行操作
这种方法适用于文件较小且修改内容较多的情况。其基本步骤包括读取整个文件内容到内存中、进行修改、将修改后的内容写回文件。
def modify_file_in_memory(file_path, target_str, replacement_str):
with open(file_path, 'r') as file:
content = file.read()
modified_content = content.replace(target_str, replacement_str)
with open(file_path, 'w') as file:
file.write(modified_content)
Usage
modify_file_in_memory('example.txt', 'old_text', 'new_text')
在上述代码中,modify_file_in_memory
函数通过read
方法读取整个文件内容,并在内存中使用replace
方法进行字符串替换,最后将修改后的内容写回文件。
三、使用正则表达式进行复杂的文本替换
对于复杂的文本替换操作,可以使用Python的re
模块。正则表达式可以处理多种复杂的字符串匹配和替换需求。
import re
def modify_file_with_regex(file_path, pattern, replacement):
with open(file_path, 'r') as file:
content = file.read()
modified_content = re.sub(pattern, replacement, content)
with open(file_path, 'w') as file:
file.write(modified_content)
Usage
modify_file_with_regex('example.txt', r'\bold_text\b', 'new_text')
在上述代码中,modify_file_with_regex
函数通过re.sub
方法使用正则表达式进行字符串匹配和替换,适用于需要复杂匹配规则的情况。
四、处理大文件的修改
对于特别大的文件,逐行读取和写入的方法更为合适,因为可以节省内存消耗。在这种情况下,可以使用生成器或迭代器来逐行处理文件内容。
def modify_large_file(input_file, output_file, target_str, replacement_str):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
modified_line = line.replace(target_str, replacement_str)
outfile.write(modified_line)
Usage
modify_large_file('large_input.txt', 'large_output.txt', 'old_text', 'new_text')
在上述代码中,modify_large_file
函数使用文件对象作为上下文管理器,逐行读取输入文件内容并进行修改,然后将修改后的内容写入输出文件。
五、示例:将多种方法结合应用
在实际应用中,可能需要将多种方法结合使用。例如,先使用正则表达式进行复杂的文本替换,然后逐行写回文件。
import re
def complex_modify_file(input_file, output_file, patterns_replacements):
with open(input_file, 'r') as infile, open(output_file, 'w') as outfile:
for line in infile:
modified_line = line
for pattern, replacement in patterns_replacements:
modified_line = re.sub(pattern, replacement, modified_line)
outfile.write(modified_line)
Usage
patterns_replacements = [
(r'\bold_text1\b', 'new_text1'),
(r'\bold_text2\b', 'new_text2'),
(r'\bpattern\b', 'replacement')
]
complex_modify_file('complex_input.txt', 'complex_output.txt', patterns_replacements)
在上述代码中,complex_modify_file
函数接受一个包含多个模式和替换字符串的列表,逐行读取和修改文件内容,并将修改后的内容写回文件。这种方法适用于需要进行多种复杂替换的情况。
通过上述几种方法,可以实现对txt文件内容的多种修改需求。选择合适的方法取决于具体应用场景和文件大小。在实际应用中,可以根据需要灵活组合使用这些方法,以实现最佳效果。
相关问答FAQs:
如何在Python中打开和读取txt文件的内容?
在Python中,可以使用内置的open()
函数来打开txt文件。使用'r'
模式可以读取文件内容,使用read()
方法读取整个文件或使用readlines()
方法读取每一行。示例如下:
with open('example.txt', 'r') as file:
content = file.read() # 读取整个文件
# 或者
lines = file.readlines() # 逐行读取文件
这种方法能够让你轻松访问文件中的信息,以便后续进行修改。
在Python中如何写入或更新txt文件的内容?
为了写入或更新txt文件,可以使用open()
函数的'w'
或'a'
模式。'w'
模式会覆盖原有内容,而'a'
模式会在文件末尾添加内容。以下是示例代码:
with open('example.txt', 'w') as file:
file.write('新的内容') # 使用'w'模式覆盖文件内容
with open('example.txt', 'a') as file:
file.write('\n附加内容') # 使用'a'模式添加内容
这种方式使得用户可以根据需求选择是覆盖还是追加内容。
如何在Python中处理文件内容的特定修改?
如果需要在文件中进行特定的内容修改,首先需要读取文件内容,进行字符串操作后再写入文件。可以使用replace()
方法替换指定内容。示例代码如下:
with open('example.txt', 'r') as file:
content = file.read()
content = content.replace('旧内容', '新内容') # 替换指定字符串
with open('example.txt', 'w') as file:
file.write(content) # 将修改后的内容写回文件
这种方式灵活适应各种文本内容的修改需求。
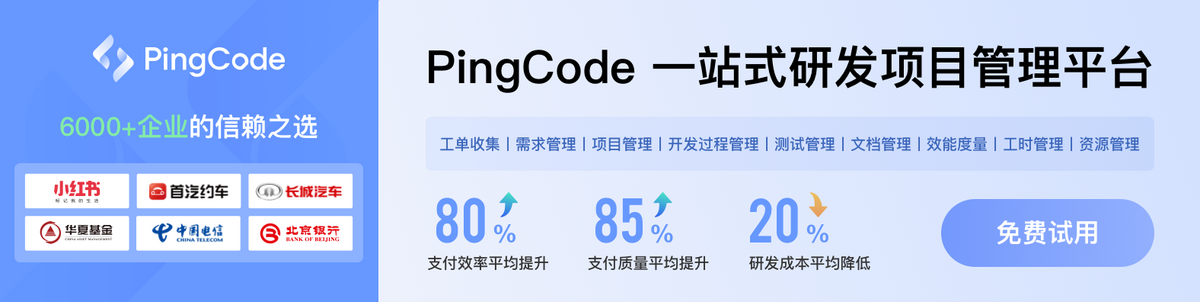