Python3写逻辑与的方法包括使用布尔运算符and
、结合if
语句、使用all()
函数。 使用布尔运算符and
是最常见的方法之一,它可以检查多个条件是否同时为真。结合if
语句,可以实现条件判断和逻辑控制。all()
函数则用于检查一个可迭代对象中的所有元素是否都为真。下面将详细介绍这三种方法。
一、布尔运算符and
布尔运算符and
是Python中用于实现逻辑与操作的基本工具。它可以用来连接两个或多个条件,并在所有条件都满足的情况下返回True
,否则返回False
。
示例代码:
x = 5
y = 10
if x > 0 and y > 0:
print("Both numbers are positive.")
else:
print("At least one number is not positive.")
在这个例子中,条件x > 0
和y > 0
都必须为真,整个表达式才会返回True
,并打印出“Both numbers are positive.”。否则,将打印出“At least one number is not positive.”。
二、结合if
语句
结合if
语句使用and
运算符,可以实现更复杂的逻辑判断。例如,判断一个数是否在某个范围内,并且是否为偶数。
示例代码:
number = 8
if number >= 1 and number <= 10 and number % 2 == 0:
print("The number is between 1 and 10 and is even.")
else:
print("The number does not meet the criteria.")
在这个例子中,条件number >= 1
、number <= 10
和number % 2 == 0
都必须为真,才会打印出“The number is between 1 and 10 and is even.
”。否则,将打印出“The number does not meet the criteria.
”。
三、使用all()
函数
all()
函数是Python内置的一个函数,用于检查一个可迭代对象(如列表、元组等)中的所有元素是否都为真。如果所有元素都为真,all()
函数返回True
,否则返回False
。这在需要检查多个条件时特别有用。
示例代码:
conditions = [x > 0, y > 0, x + y > 10]
if all(conditions):
print("All conditions are met.")
else:
print("Not all conditions are met.")
在这个例子中,conditions
是一个包含三个布尔表达式的列表。all(conditions)
检查列表中的所有条件是否都为真。如果是,则打印出“All conditions are met.
”,否则打印出“Not all conditions are met.
”。
总结
综上所述,Python3中实现逻辑与的方法主要包括使用布尔运算符and
、结合if
语句、使用all()
函数。布尔运算符and
是最常见的方法,可以方便地连接多个条件并进行判断。结合if
语句则可以实现更复杂的逻辑控制。all()
函数则提供了一种简洁的方式来检查一个可迭代对象中的所有条件是否都为真。根据具体需求,选择合适的方法,可以有效地实现逻辑与操作。
四、实际应用场景
1、数据验证
在数据验证过程中,经常需要检查多个条件是否同时满足。例如,验证用户输入的表单数据是否符合要求。
def validate_form(data):
if data['age'] > 18 and data['email'].endswith('@example.com') and data['name']:
return True
return False
form_data = {'age': 25, 'email': 'user@example.com', 'name': 'John Doe'}
if validate_form(form_data):
print("Form is valid.")
else:
print("Form is invalid.")
在这个例子中,validate_form
函数检查用户输入的年龄是否大于18、邮箱是否以@example.com
结尾、姓名是否非空。如果所有条件都满足,函数返回True
,否则返回False
。
2、复杂条件判断
在一些复杂的业务逻辑中,可能需要同时检查多个条件。例如,判断一个用户是否有权限访问某个资源,可能需要检查用户的角色、状态以及其他相关条件。
def has_access(user):
if user['role'] == 'admin' and user['active'] and not user['banned']:
return True
return False
user_info = {'role': 'admin', 'active': True, 'banned': False}
if has_access(user_info):
print("User has access.")
else:
print("User does not have access.")
在这个例子中,has_access
函数检查用户的角色是否为admin
、用户是否活跃以及用户是否未被禁止。如果所有条件都满足,函数返回True
,否则返回False
。
五、使用生成器表达式
生成器表达式可以与all()
函数结合使用,以实现更高效的逻辑与操作。生成器表达式在需要时才会生成元素,因此在处理大量数据时可以提高性能。
示例代码:
numbers = [2, 4, 6, 8, 10]
if all(n % 2 == 0 for n in numbers):
print("All numbers are even.")
else:
print("Not all numbers are even.")
在这个例子中,生成器表达式(n % 2 == 0 for n in numbers)
逐个检查列表中的每个数字是否为偶数。如果所有数字都是偶数,all()
函数返回True
,并打印出“All numbers are even.
”。否则,打印出“Not all numbers are even.
”。
六、结合逻辑或运算符
有时需要结合逻辑或运算符or
和逻辑与运算符and
来实现更复杂的条件判断。例如,判断一个用户是否为管理员或超级用户,并且用户是否活跃。
示例代码:
def has_privileges(user):
if (user['role'] == 'admin' or user['role'] == 'superuser') and user['active']:
return True
return False
user_info = {'role': 'superuser', 'active': True}
if has_privileges(user_info):
print("User has privileges.")
else:
print("User does not have privileges.")
在这个例子中,has_privileges
函数检查用户的角色是否为admin
或superuser
,并且用户是否活跃。如果条件都满足,函数返回True
,否则返回False
。
七、在列表推导式中使用逻辑与
列表推导式可以用于生成满足特定条件的列表项。在列表推导式中使用逻辑与,可以实现更复杂的条件过滤。
示例代码:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
filtered_numbers = [n for n in numbers if n > 3 and n % 2 == 0]
print(filtered_numbers) # 输出: [4, 6, 8, 10]
在这个例子中,列表推导式生成一个新列表,其中包含所有大于3且为偶数的数字。逻辑与运算符and
用于同时检查两个条件。
八、错误处理中的逻辑与
在错误处理过程中,逻辑与运算符也可以用于检查多个条件。例如,检查文件是否存在并且文件是否可读。
示例代码:
import os
file_path = 'example.txt'
if os.path.exists(file_path) and os.access(file_path, os.R_OK):
with open(file_path, 'r') as file:
content = file.read()
print(content)
else:
print("File does not exist or is not readable.")
在这个例子中,os.path.exists(file_path)
检查文件是否存在,os.access(file_path, os.R_OK)
检查文件是否可读。如果两个条件都满足,文件将被打开并读取内容。否则,将打印出“File does not exist or is not readable.
”。
九、结合函数式编程
在函数式编程中,逻辑与运算符可以与高阶函数结合使用,以实现更简洁的代码。例如,使用filter()
函数过滤满足特定条件的列表项。
示例代码:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
def is_even_and_greater_than_three(n):
return n > 3 and n % 2 == 0
filtered_numbers = list(filter(is_even_and_greater_than_three, numbers))
print(filtered_numbers) # 输出: [4, 6, 8, 10]
在这个例子中,filter()
函数用于过滤满足is_even_and_greater_than_three
条件的列表项。逻辑与运算符and
用于同时检查两个条件。
十、结合逻辑非运算符
在某些情况下,逻辑与运算符可以与逻辑非运算符not
结合使用,以实现更复杂的条件判断。例如,判断一个用户是否既不是管理员也不是超级用户,并且用户是否活跃。
示例代码:
def lacks_privileges(user):
if (user['role'] != 'admin' and user['role'] != 'superuser') and user['active']:
return True
return False
user_info = {'role': 'guest', 'active': True}
if lacks_privileges(user_info):
print("User lacks privileges but is active.")
else:
print("User does not meet the criteria.")
在这个例子中,lacks_privileges
函数检查用户的角色是否既不是admin
也不是superuser
,并且用户是否活跃。如果条件都满足,函数返回True
,否则返回False
。
总结
通过上述详细介绍,Python3中实现逻辑与的方法主要包括使用布尔运算符and
、结合if
语句、使用all()
函数、生成器表达式、逻辑或运算符结合、列表推导式、错误处理、函数式编程以及逻辑非运算符结合等。根据具体需求,选择合适的方法,可以有效地实现逻辑与操作,并在不同的应用场景中发挥重要作用。合理使用这些方法,可以使代码更简洁、高效和易于维护。
相关问答FAQs:
逻辑与在Python3中是如何实现的?
在Python3中,逻辑与操作使用关键字and
进行实现。逻辑与运算符会检查两个条件是否同时为真,只有当两个条件都为真时,整个表达式的结果才为真。例如,a = True
和b = False
时,表达式a and b
的结果为False
。
在Python3中,逻辑与运算的常见应用场景有哪些?
逻辑与运算在条件判断中非常常见,尤其是在需要同时满足多个条件的情况下。例如,在处理用户输入时,可以使用逻辑与来确保用户输入的值符合特定的范围,或者在控制流中判断多个条件是否都成立,从而执行相应的代码块。
如何在Python3中使用逻辑与进行复杂条件判断?
在Python3中,逻辑与可以与其他逻辑运算符(如or
和not
)结合使用,从而形成复杂的条件判断。例如,可以使用逻辑与将多个条件组合在一起,如if (age > 18 and age < 65) and (income > 30000):
,这段代码将检查age
是否在18到65之间,并且income
是否大于30000。
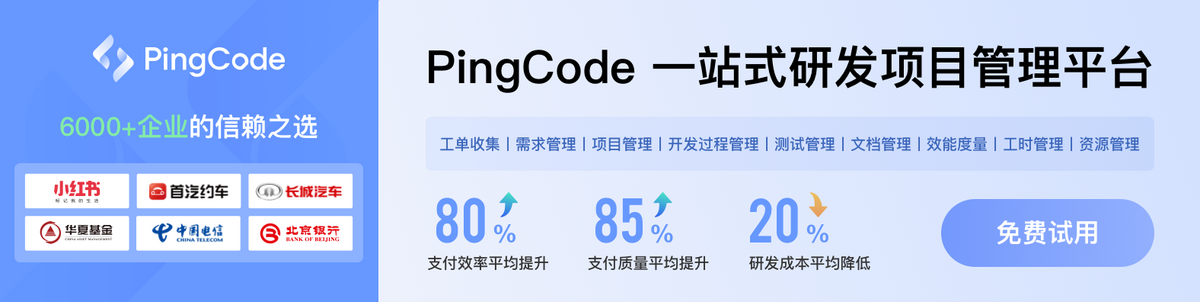