Python中可以使用函数获取数据类型的方法包括:使用type()
函数、使用isinstance()
函数、使用types
模块。 其中,最常用的就是type()
函数。下面就type()
函数进行详细描述。type()
函数可以返回对象的数据类型,使用方法简单直观。例如,对于整数、浮点数、字符串、列表等常见类型,type()
函数可以直接返回相应的类型名。
在Python中,确定数据类型是编程中非常重要的一部分,了解数据类型有助于编写更高效、更可靠的代码。下面将详细介绍获取数据类型的几种方法以及它们的应用。
一、使用type()
函数
type()
函数是Python内置的函数之一,用于返回对象的类型。其语法非常简单,直接传入一个对象即可。
# 示例代码
a = 10
b = 3.14
c = "Hello"
d = [1, 2, 3]
print(type(a)) # <class 'int'>
print(type(b)) # <class 'float'>
print(type(c)) # <class 'str'>
print(type(d)) # <class 'list'>
type()
函数的优势在于简单明了,可以快速查看变量的类型。在调试和开发过程中,这个功能非常有用。
二、使用isinstance()
函数
isinstance()
函数用于判断一个对象是否是一个已知的类型。这个函数对于需要进行类型检查和类型判断的场景非常有用。
# 示例代码
a = 10
b = 3.14
c = "Hello"
d = [1, 2, 3]
print(isinstance(a, int)) # True
print(isinstance(b, float)) # True
print(isinstance(c, str)) # True
print(isinstance(d, list)) # True
与type()
函数相比,isinstance()
函数更适合用于类型检查,因为它可以检查是否是某种类型的子类。
三、使用types
模块
types
模块包含了Python中所有标准类型的定义。通过这个模块,我们可以对一些特殊类型进行检查。
import types
def func():
pass
print(type(func)) # <class 'function'>
print(isinstance(func, types.FunctionType)) # True
检查生成器
def generator():
yield 1
gen = generator()
print(type(gen)) # <class 'generator'>
print(isinstance(gen, types.GeneratorType)) # True
使用types
模块可以更精确地检查一些特殊类型,如函数、生成器等。
四、自定义类型检查函数
在实际开发中,我们可以根据需要自定义类型检查函数,以便更灵活地处理不同类型的数据。
def check_type(variable):
if isinstance(variable, int):
return "Integer"
elif isinstance(variable, float):
return "Float"
elif isinstance(variable, str):
return "String"
elif isinstance(variable, list):
return "List"
else:
return "Unknown type"
示例代码
a = 10
b = 3.14
c = "Hello"
d = [1, 2, 3]
print(check_type(a)) # Integer
print(check_type(b)) # Float
print(check_type(c)) # String
print(check_type(d)) # List
这种自定义函数可以根据实际需求进行扩展和修改,适用于更复杂的类型检查场景。
五、使用collections.abc
模块
对于更复杂的数据结构,如容器类型,可以使用collections.abc
模块中的抽象基类进行类型检查。
from collections.abc import Iterable, Iterator
def check_iterable(variable):
if isinstance(variable, Iterable):
return "Iterable"
else:
return "Not Iterable"
def check_iterator(variable):
if isinstance(variable, Iterator):
return "Iterator"
else:
return "Not Iterator"
示例代码
a = [1, 2, 3]
b = iter(a)
print(check_iterable(a)) # Iterable
print(check_iterable(b)) # Iterable
print(check_iterator(a)) # Not Iterator
print(check_iterator(b)) # Iterator
通过collections.abc
模块,可以对容器类型进行更精确的检查,适用于需要处理复杂数据结构的场景。
六、总结
在Python中,获取数据类型的方法有很多,每种方法都有其特定的应用场景。type()
函数简单直观,适合快速查看变量类型;isinstance()
函数适合用于类型检查,尤其是检查是否为某种类型的子类;types
模块用于检查一些特殊类型,如函数、生成器等;自定义类型检查函数可以根据实际需求进行扩展和修改;collections.abc
模块适用于检查复杂数据结构,如容器类型。在实际开发中,根据具体需求选择合适的方法,可以提高代码的可读性和可靠性。
相关问答FAQs:
如何在Python中使用内置函数检查变量的数据类型?
在Python中,可以使用内置的type()
函数来获取变量的数据类型。例如,如果你有一个变量x = 10
,你可以通过调用type(x)
来获取x
的类型,返回结果将是<class 'int'>
,表示x
是一个整数类型。
如何判断一个变量是否为特定的数据类型?
除了使用type()
函数外,Python还提供了isinstance()
函数,可以用来判断一个变量是否属于某个特定的数据类型。例如,isinstance(x, int)
将返回True
,如果x
是整数类型。这种方法更为灵活,适用于判断子类和父类关系。
在Python中如何查看自定义对象的类型?
对于自定义类的对象,使用type()
函数同样有效。例如,如果你有一个类Person
,创建一个对象p = Person()
,调用type(p)
将返回<class '__main__.Person'>
,这表明p
是Person
类的实例。通过这种方式,可以轻松查看任何自定义对象的类型。
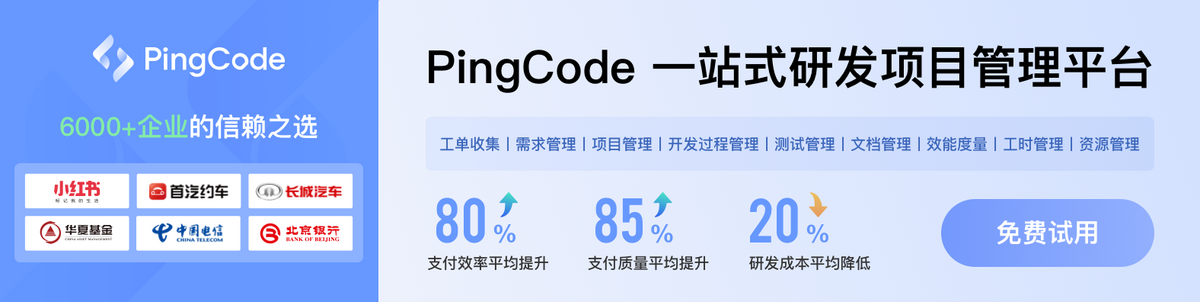