Python判断字符串为数字的方法有多种,分别是:使用字符串方法isdigit()
, isnumeric()
, isdecimal()
, 使用try-except
块进行类型转换、使用正则表达式。 其中,isdigit()
方法是最常用的一种,它能够判断字符串是否全部由数字字符组成。
最常用的isdigit()
方法适用于大多数基本场景。它会检查字符串中的每个字符是否都是数字字符,如果是,则返回True
,否则返回False
。例如:
s = "12345"
print(s.isdigit()) # 输出: True
下面将详细介绍每种方法的使用场景及其优缺点。
一、字符串方法:isdigit()
, isnumeric()
, isdecimal()
1、isdigit()
isdigit()
方法是最常用的方法之一。它检查字符串中的每个字符是否都是数字字符,包括0-9的数字字符以及其他Unicode数字字符。
s1 = "12345"
s2 = "1234a"
print(s1.isdigit()) # 输出: True
print(s2.isdigit()) # 输出: False
2、isnumeric()
isnumeric()
方法检查字符串中的每个字符是否都是数字字符或者是其他数字字符(如罗马数字、汉字数字等)。
s1 = "12345"
s2 = "一二三四五"
print(s1.isnumeric()) # 输出: True
print(s2.isnumeric()) # 输出: True
3、isdecimal()
isdecimal()
方法检查字符串中的每个字符是否都是十进制数字字符。它比isdigit()
和isnumeric()
更严格,只能判断0-9的数字字符。
s1 = "12345"
s2 = "12.34"
print(s1.isdecimal()) # 输出: True
print(s2.isdecimal()) # 输出: False
二、使用try-except
块进行类型转换
这是一种通用的解决方案,适用于判断字符串是否可以转换为整数或浮点数。通过尝试将字符串转换为int
或float
类型,如果成功则说明是数字,否则不是。
def is_number(s):
try:
float(s)
return True
except ValueError:
return False
s1 = "12345"
s2 = "12.34"
s3 = "1234a"
print(is_number(s1)) # 输出: True
print(is_number(s2)) # 输出: True
print(is_number(s3)) # 输出: False
三、使用正则表达式
正则表达式是一种强大且灵活的字符串匹配工具。可以使用正则表达式来判断字符串是否为数字。
import re
def is_number(s):
return bool(re.match(r'^\d+(\.\d+)?$', s))
s1 = "12345"
s2 = "12.34"
s3 = "1234a"
print(is_number(s1)) # 输出: True
print(is_number(s2)) # 输出: True
print(is_number(s3)) # 输出: False
四、使用str.replace()
方法处理小数点
有时候需要判断一个字符串是否为浮点数,可以先移除小数点再使用isdigit()
方法。
def is_float(s):
if s.count('.') == 1:
s = s.replace('.', '', 1)
return s.isdigit()
s1 = "12345"
s2 = "12.34"
s3 = "12.34.56"
print(is_float(s1)) # 输出: True
print(is_float(s2)) # 输出: True
print(is_float(s3)) # 输出: False
五、综合使用多种方法
根据实际需求,可以组合多种方法来判断字符串是否为数字,以达到最佳效果。
import re
def is_number(s):
if re.match(r'^\d+(\.\d+)?$', s):
return True
try:
float(s)
return True
except ValueError:
return False
s1 = "12345"
s2 = "12.34"
s3 = "1234a"
s4 = "一二三四五"
print(is_number(s1)) # 输出: True
print(is_number(s2)) # 输出: True
print(is_number(s3)) # 输出: False
print(is_number(s4)) # 输出: False
总结
判断字符串是否为数字有多种方法,最常用的方法是isdigit()
,适用于基本的数字判断场景。如果需要更全面的判断,可以使用isnumeric()
、isdecimal()
方法,或者使用try-except
块进行类型转换。此外,正则表达式和字符串处理方法也可以用于更复杂的场景。根据实际需求选择合适的方法,可以帮助我们在Python编程中更高效地处理字符串判断问题。
相关问答FAQs:
如何在Python中检查一个字符串是否只包含数字?
在Python中,可以使用str.isdigit()
方法来判断字符串是否只包含数字字符。该方法会返回一个布尔值,表示字符串中的所有字符是否都是数字。如果字符串包含任何非数字字符(例如字母或空格),该方法将返回False
。
Python中是否有其他方法来判断字符串是否为数字?
除了使用isdigit()
方法外,str.isnumeric()
和str.isdecimal()
也是可行的选择。isnumeric()
可以识别所有数字字符,包括分数和罗马数字,而isdecimal()
则仅识别十进制数字。根据具体的需求,可以选择最适合的方法。
如何处理包含小数点或负号的数字字符串?
对于包含小数点或负号的字符串,可以使用try-except
语句结合float()
函数进行转换。如果字符串可以被转换为浮点数,则说明它是一个有效的数字字符串。示例代码如下:
def is_number(s):
try:
float(s)
return True
except ValueError:
return False
这个方法可以有效处理整数、小数和负数的情况。
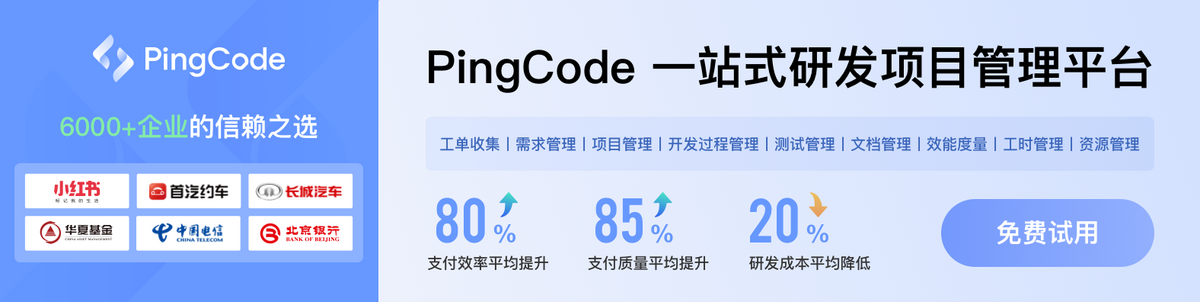