使用类的基本原则、定义构造函数、继承、使用方法实现功能
在Python中定义一个shape类涉及到几个重要的步骤和概念:使用类的基本原则、定义构造函数、继承、使用方法实现功能。我们将详细讨论这些步骤,并通过实际例子展示如何实现这些概念。
一、定义Shape类的基础
在Python中,类通过关键字class定义。一个基本的shape类可以包括一些通用的属性和方法,例如名称、颜色、计算面积和计算周长的方法。我们将从一个简单的类开始,并逐步增加复杂性。
class Shape:
def __init__(self, name, color):
self.name = name
self.color = color
def area(self):
pass
def perimeter(self):
pass
在上述代码中,我们定义了一个名为Shape的类,并使用__init__
构造函数初始化类的属性name和color。我们还定义了两个方法area和perimeter,这些方法在基本的Shape类中没有具体实现,可以在子类中进行重写。
二、继承Shape类
继承是面向对象编程的一个重要特性,可以让我们创建一个类,并继承另一个类的属性和方法。我们可以创建子类来表示具体的形状(例如Circle、Rectangle等),并重写父类的方法。
class Circle(Shape):
def __init__(self, name, color, radius):
super().__init__(name, color)
self.radius = radius
def area(self):
return 3.14159 * (self.radius 2)
def perimeter(self):
return 2 * 3.14159 * self.radius
在上述代码中,我们创建了一个名为Circle的子类,并继承了Shape类。我们使用super()
函数调用父类的构造函数来初始化name和color属性,并添加了一个新的属性radius。然后,我们重写了area和perimeter方法,以计算圆的面积和周长。
三、实现多个子类
我们可以继续创建其他子类,以表示不同的形状。每个子类都可以有自己的属性和方法。
class Rectangle(Shape):
def __init__(self, name, color, width, height):
super().__init__(name, color)
self.width = width
self.height = height
def area(self):
return self.width * self.height
def perimeter(self):
return 2 * (self.width + self.height)
class Triangle(Shape):
def __init__(self, name, color, base, height):
super().__init__(name, color)
self.base = base
self.height = height
def area(self):
return 0.5 * self.base * self.height
def perimeter(self):
# Assuming an equilateral triangle for simplicity
return 3 * self.base
在上述代码中,我们创建了两个子类Rectangle和Triangle,并重写了area和perimeter方法,以适应各自的形状。
四、使用Shape类和子类
我们可以创建Shape类和其子类的实例,并调用其方法来计算面积和周长。
# Creating instances of different shapes
circle = Circle("Circle", "Red", 5)
rectangle = Rectangle("Rectangle", "Blue", 4, 6)
triangle = Triangle("Triangle", "Green", 3, 4)
Printing the details and calculations
print(f"{circle.name} of color {circle.color} has area: {circle.area()} and perimeter: {circle.perimeter()}")
print(f"{rectangle.name} of color {rectangle.color} has area: {rectangle.area()} and perimeter: {rectangle.perimeter()}")
print(f"{triangle.name} of color {triangle.color} has area: {triangle.area()} and perimeter: {triangle.perimeter()}")
在上述代码中,我们创建了Circle、Rectangle和Triangle的实例,并调用它们的area和perimeter方法来计算和打印结果。
五、扩展Shape类
我们可以进一步扩展Shape类,以包含更多的属性和方法。例如,我们可以添加一个方法来更改形状的颜色,或添加一个方法来比较两个形状的面积。
class Shape:
def __init__(self, name, color):
self.name = name
self.color = color
def area(self):
pass
def perimeter(self):
pass
def change_color(self, new_color):
self.color = new_color
def compare_area(self, other_shape):
return self.area() > other_shape.area()
在上述代码中,我们添加了两个新方法:change_color和compare_area。change_color方法用于更改形状的颜色,compare_area方法用于比较两个形状的面积。
通过上述步骤,我们不仅定义了一个基本的Shape类,还展示了如何继承、扩展和使用它。通过这些概念和示例代码,您可以创建更复杂和多样化的形状类,以满足不同的需求。
相关问答FAQs:
如何在Python中创建一个基本的Shape类?
要创建一个基本的Shape类,可以使用Python的类定义功能。Shape类可以包含一些基本属性,比如形状的名字和面积。您可以定义一个基类Shape,然后根据需要创建其他子类(如Circle、Square等),以实现多态性和代码复用。示例代码如下:
class Shape:
def __init__(self, name):
self.name = name
def area(self):
raise NotImplementedError("Subclasses must implement this method")
如何在Shape类中添加计算面积的方法?
在Shape类中,您可以为每个子类实现特定的计算面积方法。通过在Shape类中定义一个抽象方法area,确保所有子类都实现该方法。例如,Circle类可以计算其面积使用公式πr²,Square类则使用边长的平方。示例代码如下:
import math
class Circle(Shape):
def __init__(self, radius):
super().__init__("Circle")
self.radius = radius
def area(self):
return math.pi * (self.radius ** 2)
class Square(Shape):
def __init__(self, side_length):
super().__init__("Square")
self.side_length = side_length
def area(self):
return self.side_length ** 2
如何实例化Shape类及其子类?
实例化Shape类及其子类非常简单。您只需调用构造函数并传入必要的参数。例如,创建一个Circle对象和一个Square对象,并调用它们的area方法来获取面积。示例代码如下:
circle = Circle(5)
print(f"The area of the {circle.name} is {circle.area()}")
square = Square(4)
print(f"The area of the {square.name} is {square.area()}")
这些示例展示了如何定义一个Shape类及其子类的基本方法和实例化过程,让您在Python中更加灵活地处理不同的形状。
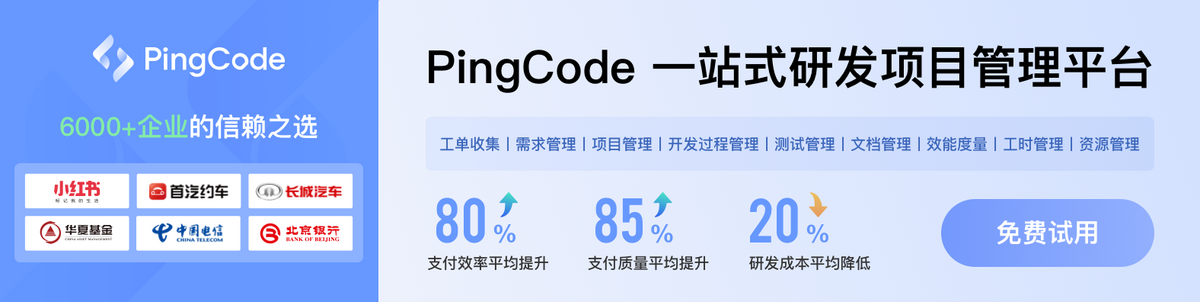