在Python中,可以通过多种方法来转换字符串格式,这些方法包括使用内置字符串方法、格式化函数和正则表达式等。以下是几种常用的字符串转换方法:字符串方法、格式化函数、正则表达式。使用内置字符串方法可以高效地实现常见的字符串转换任务,如大小写转换、去除空白字符等。本文将详细介绍这些方法,并提供相应的代码示例。
一、使用字符串方法
Python提供了一系列内置的字符串方法,这些方法可以方便地对字符串进行处理和转换。
1.1 大小写转换
大小写转换是最常见的字符串转换任务之一,Python提供了多种方法来实现这一功能。
# 全部转换为大写
s = "hello world"
print(s.upper()) # 输出:HELLO WORLD
全部转换为小写
s = "HELLO WORLD"
print(s.lower()) # 输出:hello world
首字母大写
s = "hello world"
print(s.capitalize()) # 输出:Hello world
每个单词的首字母大写
s = "hello world"
print(s.title()) # 输出:Hello World
交换大小写
s = "Hello World"
print(s.swapcase()) # 输出:hELLO wORLD
1.2 去除空白字符
在处理字符串时,去除前后的空白字符是非常常见的需求,Python提供了strip、lstrip和rstrip方法来实现这一功能。
s = " hello world "
print(s.strip()) # 输出:"hello world"
print(s.lstrip()) # 输出:"hello world "
print(s.rstrip()) # 输出:" hello world"
1.3 替换子字符串
替换子字符串是字符串处理中的另一常见任务,Python的replace方法可以实现这一功能。
s = "hello world"
print(s.replace("world", "Python")) # 输出:hello Python
1.4 分割和连接字符串
分割和连接字符串是处理字符串时常用的操作,Python提供了split和join方法来实现这一功能。
# 分割字符串
s = "hello world"
words = s.split()
print(words) # 输出:['hello', 'world']
连接字符串
s = "-".join(words)
print(s) # 输出:hello-world
二、使用格式化函数
Python还提供了多种字符串格式化函数,如format、f-string(在Python 3.6及以上版本中引入)和%操作符等,这些函数可以用于灵活地格式化字符串。
2.1 使用format方法
format方法可以通过占位符来插入变量,并指定格式。
name = "Alice"
age = 30
s = "My name is {} and I am {} years old".format(name, age)
print(s) # 输出:My name is Alice and I am 30 years old
2.2 使用f-string
f-string是Python 3.6及以上版本提供的字符串格式化方法,它可以通过在字符串前加上字母f,并在字符串中使用{}来插入变量。
name = "Alice"
age = 30
s = f"My name is {name} and I am {age} years old"
print(s) # 输出:My name is Alice and I am 30 years old
2.3 使用%操作符
%操作符是Python 2.x中的字符串格式化方法,它可以通过%来插入变量。
name = "Alice"
age = 30
s = "My name is %s and I am %d years old" % (name, age)
print(s) # 输出:My name is Alice and I am 30 years old
三、使用正则表达式
正则表达式是一种强大的字符串处理工具,可以用于复杂的字符串匹配和替换任务。Python的re模块提供了对正则表达式的支持。
3.1 匹配字符串
通过re模块的match和search方法可以实现字符串的匹配。
import re
s = "hello world"
pattern = r"hello"
match = re.match(pattern, s)
if match:
print("Match found:", match.group()) # 输出:Match found: hello
search = re.search(pattern, s)
if search:
print("Search found:", search.group()) # 输出:Search found: hello
3.2 替换字符串
通过re模块的sub方法可以实现字符串的替换。
import re
s = "hello world"
pattern = r"world"
replacement = "Python"
result = re.sub(pattern, replacement, s)
print(result) # 输出:hello Python
3.3 分割字符串
通过re模块的split方法可以实现字符串的分割。
import re
s = "hello world"
pattern = r"\s"
words = re.split(pattern, s)
print(words) # 输出:['hello', 'world']
3.4 查找所有匹配
通过re模块的findall方法可以查找字符串中所有匹配的子字符串。
import re
s = "hello world hello"
pattern = r"hello"
matches = re.findall(pattern, s)
print(matches) # 输出:['hello', 'hello']
四、综合应用
在实际应用中,字符串的转换通常需要综合运用多种方法来实现。以下是一些综合应用的示例。
4.1 转换日期格式
假设我们有一个日期字符串,格式为"YYYY-MM-DD",我们需要将其转换为"MM/DD/YYYY"格式。
import re
date_str = "2023-10-01"
pattern = r"(\d{4})-(\d{2})-(\d{2})"
replacement = r"\2/\3/\1"
formatted_date = re.sub(pattern, replacement, date_str)
print(formatted_date) # 输出:10/01/2023
4.2 转换文件路径格式
假设我们有一个文件路径字符串,格式为"folder1\folder2\file.txt",我们需要将其转换为"folder1/folder2/file.txt"格式。
import re
path_str = "folder1\\folder2\\file.txt"
formatted_path = path_str.replace("\\", "/")
print(formatted_path) # 输出:folder1/folder2/file.txt
4.3 提取电子邮件地址
假设我们有一段文本,其中包含多个电子邮件地址,我们需要提取所有的电子邮件地址。
import re
text = "Contact us at support@example.com or sales@example.com"
pattern = r"\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b"
emails = re.findall(pattern, text)
print(emails) # 输出:['support@example.com', 'sales@example.com']
4.4 转换驼峰命名为下划线命名
假设我们有一个驼峰命名的字符串,格式为"camelCaseString",我们需要将其转换为下划线命名的格式,即"camel_case_string"。
import re
camel_case_str = "camelCaseString"
pattern = r"(?<!^)(?=[A-Z])"
snake_case_str = re.sub(pattern, "_", camel_case_str).lower()
print(snake_case_str) # 输出:camel_case_string
4.5 转换下划线命名为驼峰命名
假设我们有一个下划线命名的字符串,格式为"snake_case_string",我们需要将其转换为驼峰命名的格式,即"snakeCaseString"。
import re
snake_case_str = "snake_case_string"
components = snake_case_str.split('_')
camel_case_str = components[0] + ''.join(x.title() for x in components[1:])
print(camel_case_str) # 输出:snakeCaseString
五、总结
通过本文的介绍,我们详细探讨了Python中转换字符串格式的多种方法,包括使用字符串方法、格式化函数和正则表达式等。这些方法各有优缺点,适用于不同的应用场景。希望本文对您在处理字符串时有所帮助。无论是简单的大小写转换,还是复杂的正则表达式匹配和替换,Python都提供了丰富的工具和方法来满足您的需求。
相关问答FAQs:
如何在Python中将字符串转换为日期格式?
在Python中,可以使用datetime
模块来将字符串转换为日期格式。使用strptime
方法可以指定字符串的格式。例如,若要将字符串"2023-10-01"转换为日期对象,可以使用以下代码:
from datetime import datetime
date_string = "2023-10-01"
date_object = datetime.strptime(date_string, "%Y-%m-%d")
print(date_object)
这种方法可以处理多种日期格式,确保在转换时格式字符串与输入一致。
Python中如何将字符串转换为数字类型?
使用Python内置的int()
和float()
函数可以轻松将字符串转换为整数或浮点数。例如,若有字符串"123",可以通过以下方式将其转换为整数:
number_string = "123"
number = int(number_string)
print(number)
对于浮点数,使用float()
函数类似:
float_string = "123.45"
float_number = float(float_string)
print(float_number)
注意确保字符串中只包含数字或小数点,以避免转换错误。
如何在Python中修改字符串的格式?
在Python中,可以通过字符串的内置方法来修改字符串格式。例如,使用upper()
或lower()
方法可以将字符串转换为大写或小写:
original_string = "Hello World"
upper_string = original_string.upper()
lower_string = original_string.lower()
此外,使用replace()
方法可以替换字符串中的特定字符或子字符串,例如:
modified_string = original_string.replace("World", "Python")
这些方法使得在处理和格式化字符串时更加灵活和方便。
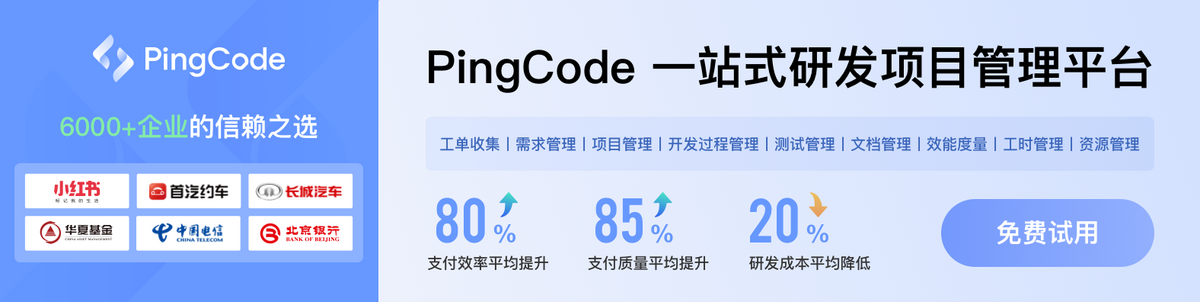