在Python中,有多种方法可以获取模块内的所有函数。你可以使用dir()
函数、inspect
模块、pkgutil
模块等方法。其中,最常用和高效的方法之一是使用inspect
模块,因为它提供了更丰富的反射能力,能够获取更多关于模块内对象的信息。下面将详细介绍其中一种方法并展开描述。
使用inspect
模块能够更方便地获取模块内的所有函数。inspect
模块提供了许多有用的函数来获取对象的详细信息,包括模块内的类、函数、方法等。通过inspect.getmembers()
函数,可以获取模块内的所有成员,并可以通过指定类型过滤出函数。
import inspect
import mymodule
获取模块内的所有函数
functions_list = inspect.getmembers(mymodule, inspect.isfunction)
for name, func in functions_list:
print(f"Function name: {name}, Function object: {func}")
这种方法不仅能获取函数名,还能获取函数对象,便于进一步操作。
一、使用dir()
函数
dir()
函数可以返回一个列表,包含指定模块内的所有名称。你可以通过遍历这个列表,并使用callable()
函数来检查每个名称是否为函数。
import mymodule
获取模块内的所有名称
names = dir(mymodule)
筛选出所有函数
functions = [name for name in names if callable(getattr(mymodule, name))]
print(functions)
这种方法虽然简单,但无法区分函数和其他可调用对象(如类、方法等),可能会包含一些非函数对象。
二、使用inspect
模块
inspect
模块提供了更强大的反射能力,可以更准确地获取模块内的函数。inspect.getmembers()
函数可以获取模块内的所有成员,并通过指定类型过滤出函数。
import inspect
import mymodule
获取模块内的所有函数
functions_list = inspect.getmembers(mymodule, inspect.isfunction)
for name, func in functions_list:
print(f"Function name: {name}, Function object: {func}")
这种方法不仅能获取函数名,还能获取函数对象,便于进一步操作。
三、使用pkgutil
模块
pkgutil
模块可以用于遍历包内的所有模块,并获取模块内的所有成员。结合inspect
模块,可以更方便地获取包内所有模块的函数。
import pkgutil
import inspect
import mypackage
获取包内所有模块
modules = [name for _, name, _ in pkgutil.iter_modules(mypackage.__path__)]
遍历所有模块,获取函数
for module_name in modules:
module = __import__(f"{mypackage.__name__}.{module_name}", fromlist=[module_name])
functions_list = inspect.getmembers(module, inspect.isfunction)
for name, func in functions_list:
print(f"Function name: {name}, Function object: {func}")
这种方法适用于获取包内所有模块的函数,适用范围更广。
四、使用globals()
和locals()
函数
在特定情况下,可以使用globals()
和locals()
函数获取当前作用域内的所有名称,并筛选出函数。这种方法适用于获取当前模块内的函数。
# 获取当前模块内的所有名称
names = globals()
筛选出所有函数
functions = {name: obj for name, obj in names.items() if callable(obj)}
print(functions)
这种方法简单高效,但仅适用于当前模块内的函数。
五、结合正则表达式筛选函数
在某些情况下,可以结合正则表达式筛选函数名,进一步提高筛选的精确度。例如,筛选出以特定前缀命名的函数。
import inspect
import re
import mymodule
获取模块内的所有函数
functions_list = inspect.getmembers(mymodule, inspect.isfunction)
筛选出以特定前缀命名的函数
prefix = "myfunc_"
filtered_functions = [func for name, func in functions_list if re.match(f"^{prefix}", name)]
for func in filtered_functions:
print(f"Filtered function: {func}")
这种方法适用于需要进一步筛选特定命名规则的函数。
六、获取函数的详细信息
除了获取函数名和对象外,还可以使用inspect
模块获取函数的详细信息,如函数的参数、默认值、注释等。
import inspect
import mymodule
获取模块内的所有函数
functions_list = inspect.getmembers(mymodule, inspect.isfunction)
for name, func in functions_list:
# 获取函数的详细信息
signature = inspect.signature(func)
docstring = inspect.getdoc(func)
print(f"Function name: {name}")
print(f"Signature: {signature}")
print(f"Docstring: {docstring}")
print("-" * 40)
这种方法适用于需要获取函数的详细信息,以便进行更深入的分析和操作。
七、获取类内的所有方法
除了获取模块内的函数外,还可以获取模块内类的所有方法。使用inspect
模块可以方便地获取类的成员,并筛选出方法。
import inspect
import mymodule
获取模块内的所有类
classes_list = inspect.getmembers(mymodule, inspect.isclass)
for name, cls in classes_list:
# 获取类的所有方法
methods_list = inspect.getmembers(cls, inspect.isfunction)
for method_name, method in methods_list:
print(f"Class: {name}, Method name: {method_name}, Method object: {method}")
这种方法适用于需要获取类内所有方法的场景。
八、使用第三方库pydoc
pydoc
库是Python自带的文档生成工具,支持生成模块的文档信息。可以结合pydoc
获取模块内的所有函数。
import pydoc
import mymodule
生成模块的文档信息
doc = pydoc.render_doc(mymodule)
解析文档信息,获取函数列表
functions = [line.split()[1] for line in doc.splitlines() if line.startswith("def ")]
print(functions)
这种方法适用于需要生成模块文档并获取函数列表的场景。
通过以上多种方法,你可以根据具体需求选择合适的方式获取模块内的所有函数。每种方法各有优劣,适用于不同的场景和需求。
相关问答FAQs:
如何在Python中列出一个模块中的所有函数?
可以使用内置的dir()
函数来获取模块中定义的所有名称,包括函数。结合inspect
模块的isfunction()
方法,可以轻松筛选出函数。例如,导入模块后,使用以下代码可以获取所有函数的列表:
import my_module # 替换为你的模块名
import inspect
functions_list = [func for func in dir(my_module) if inspect.isfunction(getattr(my_module, func))]
print(functions_list)
在Python中获取模块内函数的详细信息,有哪些方法?
除了简单列出函数名,你还可以使用inspect.getmembers()
来获取函数的详细信息。此方法不仅返回函数名,还可以提供其文档字符串和其他属性。示例如下:
import my_module
import inspect
functions_info = inspect.getmembers(my_module, inspect.isfunction)
for name, func in functions_info:
print(f"Function Name: {name}, Doc: {func.__doc__}")
如何过滤出特定条件下的函数?
如果需要根据特定条件过滤函数,例如只获取以特定前缀命名的函数,可以在获取函数列表后添加条件判断。示例代码如下:
import my_module
import inspect
filtered_functions = [func for func in dir(my_module) if inspect.isfunction(getattr(my_module, func)) and func.startswith('prefix_')]
print(filtered_functions)
通过这些方法,你可以灵活地获取模块内的函数,并根据需要进行筛选和展示。
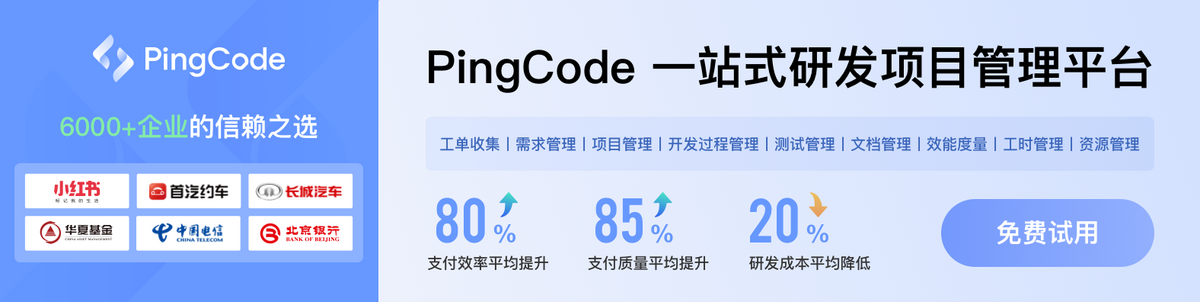