判断Python中文件指针是否指向末尾,可以通过以下几种方法:使用tell()和seek()方法、使用read()方法、使用os库中的stat()方法。其中,最常用且简单的方法是使用tell()和seek()方法。通过tell()方法获取当前文件指针的位置,然后将文件指针移动到文件末尾,通过对比两者的位置来判断文件指针是否已经指向末尾。
一、使用tell()和seek()方法
在Python中,文件对象提供了tell()方法和seek()方法,利用这两个方法可以很方便地判断文件指针是否指向文件末尾。下面具体介绍这两个方法的使用:
1.1 tell()方法
tell()方法用于获取文件指针当前位置的偏移量。返回值为从文件开始到当前文件指针所在位置的字节数。
with open('example.txt', 'r') as file:
position = file.tell()
print(f'Current file pointer position: {position}')
1.2 seek()方法
seek()方法用于移动文件指针到指定位置。它有两个参数:第一个参数是偏移量,第二个参数是可选的,表示从哪里开始移动(0表示从文件开头,1表示从当前位置,2表示从文件末尾)。
with open('example.txt', 'r') as file:
file.seek(0, 2) # Move the file pointer to the end of the file
end_position = file.tell()
print(f'End file pointer position: {end_position}')
1.3 综合使用tell()和seek()方法
通过将文件指针移动到文件末尾,然后比较当前位置和文件末尾位置来判断文件指针是否已经指向末尾。
with open('example.txt', 'r') as file:
current_position = file.tell()
file.seek(0, 2) # Move the file pointer to the end of the file
end_position = file.tell()
if current_position == end_position:
print('The file pointer is at the end of the file.')
else:
print('The file pointer is not at the end of the file.')
二、使用read()方法
使用read()方法也可以判断文件指针是否指向文件末尾。read()方法在文件指针已经到达文件末尾时会返回一个空字符串。
2.1 直接使用read()方法
通过调用read()方法读取文件内容,如果返回空字符串,则说明文件指针已经到达文件末尾。
with open('example.txt', 'r') as file:
if file.read() == '':
print('The file pointer is at the end of the file.')
else:
print('The file pointer is not at the end of the file.')
2.2 结合seek()和read()方法
我们可以先记录当前文件指针的位置,然后使用read()方法读取文件内容,再恢复文件指针的位置。
with open('example.txt', 'r') as file:
current_position = file.tell()
if file.read() == '':
print('The file pointer is at the end of the file.')
else:
print('The file pointer is not at the end of the file.')
file.seek(current_position) # Restore the file pointer position
三、使用os库中的stat()方法
os库中的stat()方法可以获取文件的相关信息,包括文件大小。通过对比文件指针当前位置和文件大小,可以判断文件指针是否已经指向文件末尾。
3.1 使用os.stat()方法获取文件大小
首先,需要导入os模块,然后使用os.stat()方法获取文件的大小信息。
import os
file_path = 'example.txt'
file_size = os.stat(file_path).st_size
print(f'File size: {file_size} bytes')
3.2 结合tell()方法和os.stat()方法
通过对比文件指针当前位置和文件大小来判断文件指针是否已经指向文件末尾。
import os
file_path = 'example.txt'
with open(file_path, 'r') as file:
current_position = file.tell()
file_size = os.stat(file_path).st_size
if current_position == file_size:
print('The file pointer is at the end of the file.')
else:
print('The file pointer is not at the end of the file.')
四、综合使用以上方法
在实际应用中,可以根据具体需求选择合适的方法来判断文件指针是否指向文件末尾。以下是一个综合使用以上方法的示例:
import os
def is_file_pointer_at_end(file):
current_position = file.tell()
# Method 1: Using tell() and seek() methods
file.seek(0, 2) # Move the file pointer to the end of the file
end_position = file.tell()
file.seek(current_position) # Restore the file pointer position
if current_position == end_position:
return True
# Method 2: Using read() method
file.seek(current_position) # Ensure the file pointer is at the original position
if file.read() == '':
file.seek(current_position) # Restore the file pointer position
return True
# Method 3: Using os.stat() method
file_size = os.stat(file.name).st_size
if current_position == file_size:
return True
return False
file_path = 'example.txt'
with open(file_path, 'r') as file:
if is_file_pointer_at_end(file):
print('The file pointer is at the end of the file.')
else:
print('The file pointer is not at the end of the file.')
综上所述,判断Python中文件指针是否指向末尾的方法有多种,包括使用tell()和seek()方法、使用read()方法、使用os库中的stat()方法。根据具体需求选择合适的方法可以有效地判断文件指针是否已经到达文件末尾。
相关问答FAQs:
如何在Python中检查文件指针的位置?
在Python中,您可以使用file.tell()
方法来获取当前文件指针的位置。该方法返回一个整数,表示文件中当前指针所处的字节位置。如果返回的值等于文件的总字节数,则表示文件指针指向末尾。
如果文件指针在末尾,我该如何处理?
当文件指针指向末尾时,您可能会需要重新定位指针。可以使用file.seek(0)
方法将指针移动到文件的开头,或者使用file.seek(offset, whence)
方法根据需要重新定位指针。适当的处理方式取决于您的具体需求,比如是否需要读取文件的内容或进行追加操作。
在读取文件时,如何避免文件指针超出范围?
为防止文件指针超出范围,您可以在读取文件时先检查文件是否已经到达末尾。使用file.read(size)
方法时,您可以将size
参数设置为适当的值,或者在每次读取后使用file.tell()
来确认当前指针位置,确保不会试图读取超过文件内容的部分。使用file.readline()
方法时,文件指针会自动移动到下一行,从而避免超出范围的问题。
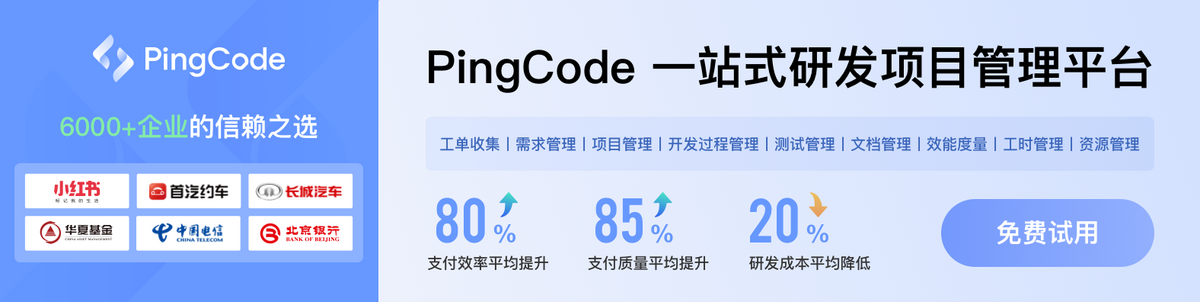