要让Python定时器持续运行,可以使用threading
库、while
循环、schedule
库等多种方式。下面将具体介绍如何通过这些方法实现Python定时器的持续运行。
一、使用threading
库
Python的threading
库提供了一个Timer
类,可以用来创建定时器。定时器可以在指定的时间间隔后执行一个函数。要让定时器持续运行,可以在定时器函数中重新启动定时器。
import threading
def repeat_function():
print("This function runs every 5 seconds")
# 重新启动定时器
threading.Timer(5, repeat_function).start()
启动定时器
repeat_function()
在这个例子中,repeat_function
函数将在每次执行时重新启动定时器,从而实现持续运行。
二、使用while
循环和time.sleep
另一种方法是使用while
循环和time.sleep
函数。这种方法的好处是简单易懂,但需要注意不要阻塞主线程。
import time
def repeat_function():
print("This function runs every 5 seconds")
while True:
repeat_function()
time.sleep(5)
在这个例子中,while
循环将一直运行,并在每次迭代时调用repeat_function
函数,然后休眠5秒。
三、使用schedule
库
schedule
库是一个轻量级的任务调度库,非常适合定时任务。它允许你以更人性化的方式定义任务调度。
import schedule
import time
def repeat_function():
print("This function runs every 5 seconds")
每5秒运行一次函数
schedule.every(5).seconds.do(repeat_function)
while True:
schedule.run_pending()
time.sleep(1)
在这个例子中,使用schedule
库定义任务调度,并在while
循环中运行所有待执行的任务。
四、使用asyncio
库
asyncio
库是Python的异步编程库,适用于需要并发执行的任务。可以通过asyncio
库实现异步定时器。
import asyncio
async def repeat_function():
while True:
print("This function runs every 5 seconds")
await asyncio.sleep(5)
创建事件循环
loop = asyncio.get_event_loop()
运行任务
loop.run_until_complete(repeat_function())
在这个例子中,使用asyncio
库创建一个异步任务,该任务将在每次执行后休眠5秒。
五、使用APScheduler
库
APScheduler
(Advanced Python Scheduler)是一个功能强大的任务调度库,支持基于时间间隔、日期、cron表达式等多种调度方式。
from apscheduler.schedulers.background import BackgroundScheduler
import time
def repeat_function():
print("This function runs every 5 seconds")
scheduler = BackgroundScheduler()
scheduler.add_job(repeat_function, 'interval', seconds=5)
scheduler.start()
try:
while True:
time.sleep(1)
except (KeyboardInterrupt, SystemExit):
scheduler.shutdown()
在这个例子中,使用APScheduler
库创建一个后台任务调度器,并将任务添加到调度器中。
六、使用Twisted
库
Twisted
是一个事件驱动的网络编程框架,可以用来实现定时器。它适用于对性能要求较高的场景。
from twisted.internet import reactor
def repeat_function():
print("This function runs every 5 seconds")
reactor.callLater(5, repeat_function)
启动定时器
reactor.callLater(5, repeat_function)
reactor.run()
在这个例子中,使用Twisted
库的reactor
对象创建一个定时器,并在每次执行后重新启动定时器。
七、使用Tkinter
库
如果你正在开发一个GUI应用程序,可以使用Tkinter
库的after
方法实现定时器。
import tkinter as tk
def repeat_function():
print("This function runs every 5 seconds")
# 重新启动定时器
root.after(5000, repeat_function)
root = tk.Tk()
启动定时器
root.after(5000, repeat_function)
root.mainloop()
在这个例子中,使用Tkinter
库的after
方法创建一个定时器,并在每次执行后重新启动定时器。
八、使用multiprocessing
库
multiprocessing
库允许你创建独立的进程,可以用来实现定时器。这样做的好处是不会阻塞主线程。
from multiprocessing import Process
import time
def repeat_function():
while True:
print("This function runs every 5 seconds")
time.sleep(5)
if __name__ == "__main__":
p = Process(target=repeat_function)
p.start()
p.join()
在这个例子中,使用multiprocessing
库创建一个独立的进程,该进程将在每次执行后休眠5秒。
九、使用concurrent.futures
库
concurrent.futures
库提供了一个高级的接口来并发执行任务。可以使用ThreadPoolExecutor
或ProcessPoolExecutor
来实现定时器。
import concurrent.futures
import time
def repeat_function():
while True:
print("This function runs every 5 seconds")
time.sleep(5)
if __name__ == "__main__":
with concurrent.futures.ThreadPoolExecutor() as executor:
executor.submit(repeat_function)
在这个例子中,使用concurrent.futures
库的ThreadPoolExecutor
创建一个线程池,并提交一个定时任务。
通过上述多种方法,你可以根据具体需求选择合适的方式来实现Python定时器的持续运行。每种方法都有其优缺点,选择时需要综合考虑代码的可读性、性能、扩展性等因素。
相关问答FAQs:
如何在Python中创建一个持续运行的定时器?
要创建一个持续运行的定时器,您可以使用threading
模块中的Timer
类。通过设置一个循环,您可以在指定的时间间隔内重复执行某个函数。示例代码如下:
import threading
def my_function():
print("定时器触发了!")
threading.Timer(5, my_function).start() # 每5秒调用一次my_function
my_function() # 启动定时器
这段代码会每5秒打印一次“定时器触发了!”。您可以根据需要调整时间间隔。
如何在Python中停止一个正在运行的定时器?
要停止一个正在运行的定时器,您需要保持对定时器实例的引用,并调用其cancel()
方法。示例代码如下:
import threading
timer = None
def start_timer():
global timer
timer = threading.Timer(5, my_function)
timer.start()
def stop_timer():
global timer
if timer is not None:
timer.cancel()
timer = None
print("定时器已停止。")
# 启动定时器
start_timer()
# 停止定时器
stop_timer()
这样可以有效地控制定时器的启动和停止。
在Python中使用定时器时,如何确保线程安全?
在多线程环境中使用定时器时,确保线程安全是非常重要的。可以使用threading.Lock()
来防止多个线程同时访问共享资源。以下是一个使用锁的示例:
import threading
lock = threading.Lock()
timer = None
def my_function():
with lock:
print("定时器触发了!")
def start_timer():
global timer
with lock:
timer = threading.Timer(5, my_function)
timer.start()
def stop_timer():
global timer
with lock:
if timer is not None:
timer.cancel()
timer = None
print("定时器已停止。")
通过使用锁,可以确保在定时器触发时,其他线程不会同时执行与定时器相关的操作,从而避免潜在的竞争条件。
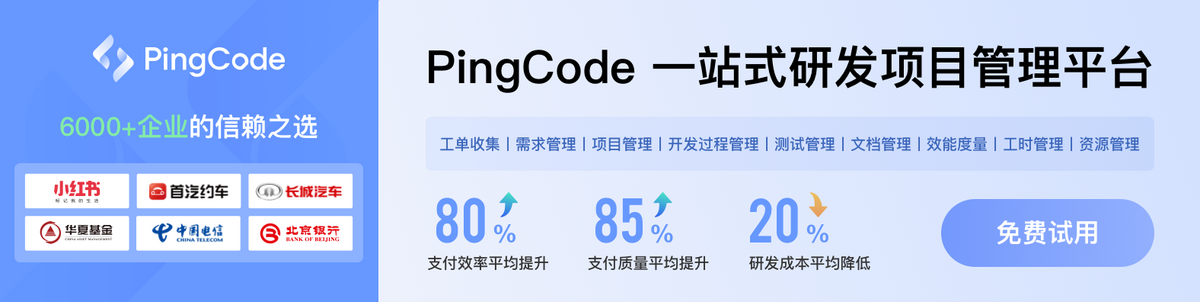