使用Python统计每个单词的个数的方法包括:使用字典、使用collections.Counter、使用正则表达式。最常用的方法是使用字典。 通过字典统计每个单词的个数时,可以通过遍历文本中的每个单词,并将其存储在字典中,同时增加其出现的次数。以下是详细描述:
使用字典统计单词个数的步骤包括:读取文本、分割单词、遍历单词并存储在字典中、输出结果。具体示例如下:
def count_words(text):
word_count = {}
words = text.split()
for word in words:
word = word.lower().strip(".,!?:;()[]{}\"'")
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
text = "Hello world! This is a test. Hello again, world!"
result = count_words(text)
print(result)
一、读取文本
首先,需要读取文本内容,可以从文件中读取,也可以从字符串中读取。读取文本的方式有很多种,取决于具体应用场景。以下是从文件中读取文本的示例:
with open('sample.txt', 'r') as file:
text = file.read()
如果文本内容是字符串,则直接将其赋值给变量:
text = "Hello world! This is a test. Hello again, world!"
二、分割单词
将读取到的文本内容进行分割,得到单词列表。可以使用字符串的 split()
方法,默认以空白字符(空格、换行、制表符等)分割:
words = text.split()
三、遍历单词并存储在字典中
创建一个空字典,然后遍历分割后的单词列表,将单词存储在字典中,并记录每个单词出现的次数。对于每个单词,将其转换为小写,并去除标点符号,以确保统计结果准确:
word_count = {}
for word in words:
word = word.lower().strip(".,!?:;()[]{}\"'")
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
四、输出结果
遍历字典,输出每个单词及其对应的出现次数:
for word, count in word_count.items():
print(f"{word}: {count}")
五、使用collections.Counter
Python的 collections
模块提供了 Counter
类,可以方便地统计单词出现的次数。以下是使用 Counter
进行单词统计的示例:
from collections import Counter
def count_words(text):
words = text.lower().split()
words = [word.strip(".,!?:;()[]{}\"'") for word in words]
return Counter(words)
text = "Hello world! This is a test. Hello again, world!"
result = count_words(text)
print(result)
六、使用正则表达式
通过正则表达式可以更灵活地分割单词,处理各种复杂的文本内容。以下是使用正则表达式进行单词统计的示例:
import re
from collections import Counter
def count_words(text):
words = re.findall(r'\b\w+\b', text.lower())
return Counter(words)
text = "Hello world! This is a test. Hello again, world!"
result = count_words(text)
print(result)
七、处理大文件
对于大文件,逐行读取文件可以有效减少内存使用。以下是逐行读取文件并统计单词个数的示例:
from collections import Counter
import re
def count_words_in_file(file_path):
word_count = Counter()
with open(file_path, 'r') as file:
for line in file:
words = re.findall(r'\b\w+\b', line.lower())
word_count.update(words)
return word_count
file_path = 'large_sample.txt'
result = count_words_in_file(file_path)
print(result)
八、多线程和多进程处理
对于极其庞大的文件,可以使用多线程或多进程进行并行处理,以提高效率。以下是使用 concurrent.futures
模块实现多进程单词统计的示例:
from concurrent.futures import ProcessPoolExecutor
from collections import Counter
import re
def count_words_in_chunk(text_chunk):
words = re.findall(r'\b\w+\b', text_chunk.lower())
return Counter(words)
def count_words_in_file(file_path):
word_count = Counter()
with open(file_path, 'r') as file:
text_chunks = file.read().split('\n')
with ProcessPoolExecutor() as executor:
results = executor.map(count_words_in_chunk, text_chunks)
for result in results:
word_count.update(result)
return word_count
file_path = 'large_sample.txt'
result = count_words_in_file(file_path)
print(result)
通过以上方法,可以有效地统计文本中每个单词的出现次数。选择合适的方法取决于具体的应用场景和文本内容大小。使用字典、collections.Counter和正则表达式是最常用和高效的方法。在处理大文件时,可以通过逐行读取文件和使用多线程或多进程来提高处理效率。此外,还可以根据具体需求进行进一步优化和调整,以获得最佳的统计结果。
相关问答FAQs:
如何使用Python统计文本中的单词频率?
在Python中,可以利用collections
模块中的Counter
类来方便地统计文本中每个单词的频率。首先,读取文本内容并进行分词,然后使用Counter来计算每个单词出现的次数。示例代码如下:
from collections import Counter
text = "这是一个示例文本,其中包含一些重复的单词。单词的统计是个有趣的任务。"
words = text.split() # 根据空格分词
word_count = Counter(words)
print(word_count)
在统计单词时,如何处理大小写和标点符号?
为了确保统计的准确性,可以在分词之前将文本转换为统一的小写形式,并去除标点符号。例如,可以使用str.lower()
方法来转换大小写,并使用正则表达式来去除标点。以下是处理大小写和标点的示例:
import re
from collections import Counter
text = "这是一个示例文本,其中包含一些重复的单词。单词的统计是个有趣的任务。"
text = text.lower() # 转换为小写
text = re.sub(r'[^\w\s]', '', text) # 去除标点符号
words = text.split()
word_count = Counter(words)
print(word_count)
如何将统计结果保存到文件中?
统计结果可以轻松保存到文本文件中,以便后续分析。可以使用Python的内置文件操作功能来实现。以下是将单词统计结果写入文件的示例代码:
with open('word_count.txt', 'w', encoding='utf-8') as f:
for word, count in word_count.items():
f.write(f"{word}: {count}\n")
通过这些方法,用户能够有效地利用Python进行单词统计,处理文本中的各种情况,并将结果保存以供进一步分析。
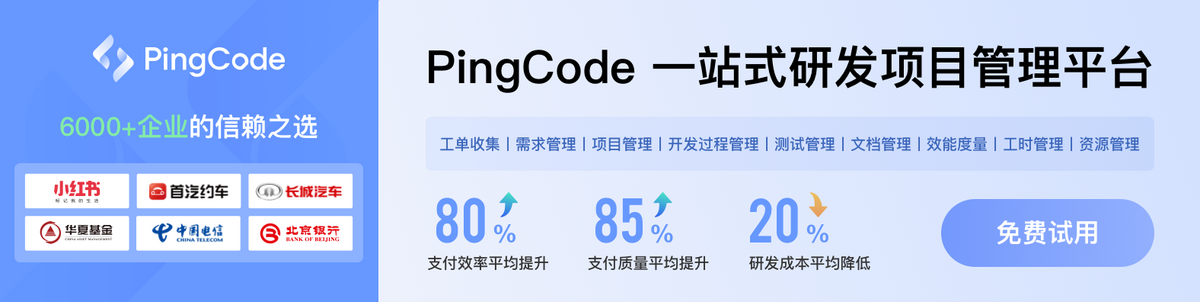