在Python中,输出文件路径的方式有很多种,主要取决于你具体需要做什么。os.path模块、Pathlib模块、os.getcwd()函数、__file__变量是最常用的四种方法。下面我将详细介绍其中的os.path模块,并在后续部分介绍其他方法及其用法。
一、os.path模块
os.path模块是Python标准库的一部分,用于处理文件和目录路径。以下是一些常用的功能:
1. os.path.abspath()
os.path.abspath()
函数返回指定路径的绝对路径。绝对路径是指从根目录开始的完整路径。
import os
relative_path = "example.txt"
absolute_path = os.path.abspath(relative_path)
print(absolute_path)
在这个例子中,os.path.abspath()
将相对路径example.txt
转换为绝对路径并输出。
2. os.path.join()
os.path.join()
函数将多个路径组件组合成一个路径。它会根据操作系统使用正确的路径分隔符。
import os
directory = "/home/user"
filename = "example.txt"
full_path = os.path.join(directory, filename)
print(full_path)
在这个例子中,os.path.join()
将目录路径和文件名组合成一个完整的文件路径。
二、Pathlib模块
Pathlib模块是Python 3.4引入的一个新模块,提供面向对象的文件系统路径操作。它更加简洁和易于使用。
1. Pathlib的Path类
Pathlib
模块中的Path
类可以方便地处理文件路径。
from pathlib import Path
创建Path对象
file_path = Path("example.txt")
获取绝对路径
absolute_path = file_path.resolve()
print(absolute_path)
在这个例子中,resolve()
方法将相对路径转换为绝对路径。
2. 使用Path进行路径组合
Pathlib
模块的Path
类还可以方便地组合路径。
from pathlib import Path
directory = Path("/home/user")
filename = "example.txt"
full_path = directory / filename
print(full_path)
在这个例子中,我们使用/
操作符来组合路径组件。
三、os.getcwd()函数
os.getcwd()
函数返回当前工作目录的路径。它可以用来获取当前脚本所在的目录。
import os
current_directory = os.getcwd()
print(current_directory)
在这个例子中,os.getcwd()
返回当前工作目录的绝对路径。
四、__file__变量
__file__
变量包含当前脚本的文件路径。可以通过os.path
或Pathlib
模块进一步处理它。
import os
current_script = __file__
script_directory = os.path.dirname(current_script)
print(script_directory)
在这个例子中,__file__
变量提供当前脚本的路径,os.path.dirname()
函数获取其目录部分。
综合实例
以下是一个综合实例,展示如何使用上述方法处理文件路径:
import os
from pathlib import Path
使用os.path模块
relative_path = "example.txt"
absolute_path = os.path.abspath(relative_path)
print(f"Absolute path (os.path): {absolute_path}")
使用Pathlib模块
file_path = Path("example.txt")
absolute_path = file_path.resolve()
print(f"Absolute path (Pathlib): {absolute_path}")
获取当前工作目录
current_directory = os.getcwd()
print(f"Current working directory: {current_directory}")
获取当前脚本的目录
current_script = __file__
script_directory = os.path.dirname(current_script)
print(f"Script directory: {script_directory}")
使用Pathlib组合路径
directory = Path("/home/user")
filename = "example.txt"
full_path = directory / filename
print(f"Full path (Pathlib): {full_path}")
结论
通过以上方法,你可以在Python中灵活地处理文件路径。os.path模块、Pathlib模块、os.getcwd()函数、__file__变量是处理文件路径的常用方法。根据具体需求选择合适的方法,可以使你的代码更加简洁和易于维护。
相关问答FAQs:
如何在Python中获取当前工作目录的文件路径?
在Python中,可以使用os
模块中的getcwd()
函数来获取当前工作目录的文件路径。示例代码如下:
import os
current_directory = os.getcwd()
print(current_directory)
运行这段代码后,将会输出当前工作目录的完整路径。
在Python中如何构建跨平台的文件路径?
为了构建跨平台的文件路径,推荐使用os.path
模块中的join()
函数。这个函数能根据操作系统自动选择合适的路径分隔符。示例代码如下:
import os
file_path = os.path.join("folder", "subfolder", "file.txt")
print(file_path)
这样可以确保在不同操作系统上(如Windows和Linux)都能正确生成文件路径。
如何在Python中检查文件路径是否存在?
可以使用os.path
模块中的exists()
函数来检查指定的文件路径是否存在。以下是一个示例代码:
import os
file_path = 'path/to/your/file.txt'
if os.path.exists(file_path):
print("文件存在")
else:
print("文件不存在")
这段代码会根据文件路径的存在性输出相应的信息。
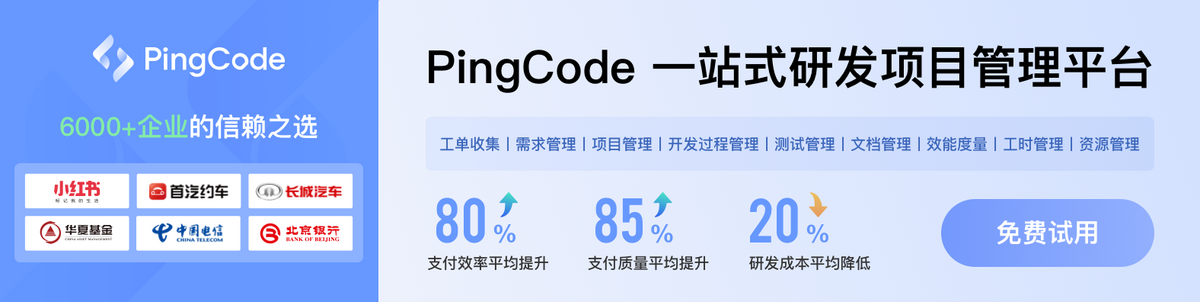