Python可以通过多种方式输入一个空间坐标:手动输入、使用内置函数、从文件读取、从数据库读取。 在本文中,我们将详细介绍这四种方式,并展示如何在实际应用中使用它们。
一、手动输入
手动输入是最简单、最直接的方式。我们可以通过Python的input()
函数来获取用户输入的坐标值。
示例代码:
def manual_input():
x = float(input("Enter the x coordinate: "))
y = float(input("Enter the y coordinate: "))
z = float(input("Enter the z coordinate: "))
return (x, y, z)
coordinates = manual_input()
print(f"The coordinates are: {coordinates}")
在这个示例中,我们使用input()
函数接收用户输入的x、y、z坐标,并将其转换为浮点数。最后,将这些坐标值以元组的形式返回。
二、使用内置函数
Python内置了一些有用的库和函数,可以简化空间坐标的输入和处理。例如,numpy
库提供了强大的数组处理功能,可以方便地处理坐标数据。
示例代码:
import numpy as np
def numpy_input():
x = float(input("Enter the x coordinate: "))
y = float(input("Enter the y coordinate: "))
z = float(input("Enter the z coordinate: "))
coordinates = np.array([x, y, z])
return coordinates
coordinates = numpy_input()
print(f"The coordinates are: {coordinates}")
在这个示例中,我们使用numpy
库将用户输入的x、y、z坐标存储在一个数组中。这种方式不仅简化了代码,还提高了代码的可读性和效率。
三、从文件读取
在实际应用中,空间坐标通常存储在文件中。我们可以使用Python的open()
函数读取文件中的坐标数据。
示例代码:
def read_coordinates_from_file(file_path):
coordinates = []
with open(file_path, 'r') as file:
for line in file:
x, y, z = map(float, line.strip().split(','))
coordinates.append((x, y, z))
return coordinates
file_path = 'coordinates.txt'
coordinates = read_coordinates_from_file(file_path)
print(f"The coordinates are: {coordinates}")
在这个示例中,我们读取一个名为coordinates.txt
的文件,并将每行的坐标数据存储在一个列表中。每行的坐标值用逗号分隔,通过map()
函数将其转换为浮点数并存储在元组中。
四、从数据库读取
在大型应用中,空间坐标通常存储在数据库中。我们可以使用Python的数据库连接库,如sqlite3
、pymysql
等,从数据库中读取坐标数据。
示例代码(使用SQLite数据库):
import sqlite3
def read_coordinates_from_db(db_path):
coordinates = []
conn = sqlite3.connect(db_path)
cursor = conn.cursor()
cursor.execute("SELECT x, y, z FROM coordinates")
rows = cursor.fetchall()
for row in rows:
x, y, z = map(float, row)
coordinates.append((x, y, z))
conn.close()
return coordinates
db_path = 'coordinates.db'
coordinates = read_coordinates_from_db(db_path)
print(f"The coordinates are: {coordinates}")
在这个示例中,我们连接到一个SQLite数据库,并执行一个SQL查询以获取坐标数据。然后,我们将查询结果存储在一个列表中。
五、总结
通过以上四种方式,我们可以方便地在Python中输入空间坐标:手动输入、使用内置函数、从文件读取、从数据库读取。每种方式都有其优缺点,选择适合自己应用的方式非常重要。
- 手动输入:适用于简单的测试和小规模数据输入,代码实现简单,但不适合大规模数据处理。
- 使用内置函数:适用于需要大量坐标处理的场景,例如科学计算和数据分析。
numpy
库提供了强大的数组处理功能,可以简化代码并提高效率。 - 从文件读取:适用于需要从外部文件中读取坐标数据的应用场景。例如,读取传感器数据或地理信息系统(GIS)数据。
- 从数据库读取:适用于需要从数据库中读取大规模坐标数据的应用场景。例如,读取地理信息系统(GIS)数据或大型数据仓库中的坐标数据。
无论选择哪种方式,都需要根据具体应用场景和需求进行选择。希望本文对你在Python中输入空间坐标有所帮助。
相关问答FAQs:
如何在Python中输入三维空间坐标?
在Python中,可以使用input()
函数结合字符串处理将用户输入的空间坐标转换为三维坐标。用户可以输入坐标,例如"1, 2, 3",然后通过split()
方法将其分割,并转换为浮点数或整数。示例代码如下:
coordinates = input("请输入空间坐标 (格式: x, y, z): ")
x, y, z = map(float, coordinates.split(','))
print(f"您输入的坐标为: x={x}, y={y}, z={z}")
如何验证输入的坐标格式是否正确?
为了确保用户输入的坐标格式正确,可以使用try-except
语句进行异常处理。通过捕获ValueError
,可以判断输入是否符合预期格式。以下是一个示例:
try:
coordinates = input("请输入空间坐标 (格式: x, y, z): ")
x, y, z = map(float, coordinates.split(','))
print(f"您输入的坐标为: x={x}, y={y}, z={z}")
except ValueError:
print("输入格式错误,请确保格式为 x, y, z,且为数字。")
如何将输入的空间坐标存储为一个数据结构?
在Python中,可以将空间坐标存储为元组、列表或字典等数据结构。使用元组可以保持坐标的不可变性,而列表则允许对坐标进行修改。示例代码如下:
coordinates = input("请输入空间坐标 (格式: x, y, z): ")
x, y, z = map(float, coordinates.split(','))
coordinate_tuple = (x, y, z)
coordinate_list = [x, y, z]
coordinate_dict = {'x': x, 'y': y, 'z': z}
print(f"元组表示的坐标: {coordinate_tuple}")
print(f"列表表示的坐标: {coordinate_list}")
print(f"字典表示的坐标: {coordinate_dict}")
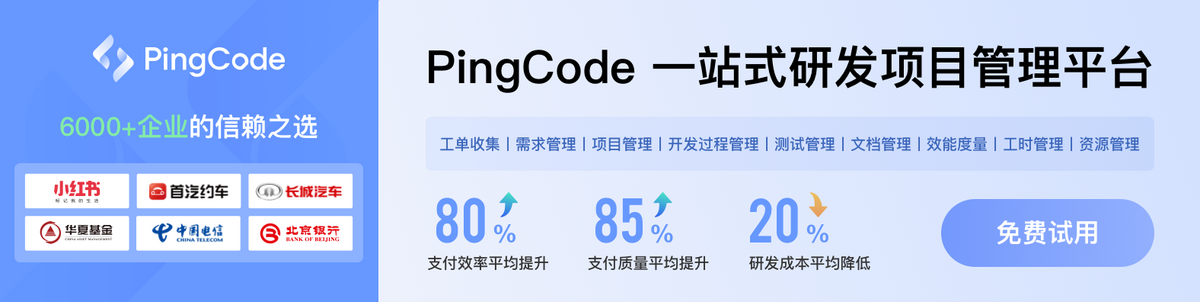