如何用Python抓取百度文档这一问题,可以通过以下几点来实现:使用爬虫库如BeautifulSoup、模拟浏览器行为、处理验证码、解析和提取文档内容。其中,使用爬虫库如BeautifulSoup是最基础且常用的方法。使用BeautifulSoup,能够快速解析HTML页面结构,从中提取需要的信息。接下来,我将详细介绍如何通过使用BeautifulSoup库来实现从百度文档抓取数据。
一、使用BeautifulSoup库解析HTML页面
BeautifulSoup是Python中最常用的HTML解析库之一,它能够将复杂的HTML页面解析成一个可以方便操作的树形结构。首先,你需要安装BeautifulSoup和请求库requests,用于发送HTTP请求并获取页面内容。以下是安装命令:
pip install beautifulsoup4 requests
安装完成后,我们可以开始编写抓取百度文档的代码。
import requests
from bs4 import BeautifulSoup
发送HTTP请求,获取页面内容
url = 'https://wenku.baidu.com/view/your_document_id.html'
response = requests.get(url)
response.encoding = 'utf-8'
解析HTML页面
soup = BeautifulSoup(response.text, 'html.parser')
提取文档内容
content = soup.find_all('div', class_='reader-word-layer')
for section in content:
print(section.get_text())
二、模拟浏览器行为
百度文档通常会对爬虫进行一些反爬虫措施,比如动态加载内容、需要登录等。为了应对这些措施,可以使用Selenium库模拟浏览器行为。Selenium可以自动化地操作浏览器,加载动态内容并处理登录等操作。以下是安装命令:
pip install selenium
同时,你还需要下载对应的浏览器驱动程序(例如ChromeDriver)。
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
设置Chrome浏览器选项
chrome_options = Options()
chrome_options.add_argument('--headless')
chrome_options.add_argument('--disable-gpu')
创建Chrome浏览器对象
service = Service('path/to/chromedriver')
driver = webdriver.Chrome(service=service, options=chrome_options)
访问百度文档页面
url = 'https://wenku.baidu.com/view/your_document_id.html'
driver.get(url)
等待页面加载完成
driver.implicitly_wait(10)
提取文档内容
content = driver.find_elements(By.CLASS_NAME, 'reader-word-layer')
for section in content:
print(section.text)
关闭浏览器
driver.quit()
三、处理验证码
在某些情况下,百度文档可能会要求输入验证码。为了处理验证码,可以使用第三方验证码识别服务,比如打码平台,或者通过人工输入验证码。在这里,以使用打码平台为例:
import requests
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.options import Options
def recognize_captcha(image_path):
# 调用打码平台API进行验证码识别
api_url = 'https://your_captcha_api_url'
api_key = 'your_api_key'
with open(image_path, 'rb') as image_file:
response = requests.post(api_url, files={'image': image_file}, data={'key': api_key})
return response.json()['code']
设置Chrome浏览器选项
chrome_options = Options()
chrome_options.add_argument('--headless')
chrome_options.add_argument('--disable-gpu')
创建Chrome浏览器对象
service = Service('path/to/chromedriver')
driver = webdriver.Chrome(service=service, options=chrome_options)
访问百度文档页面
url = 'https://wenku.baidu.com/view/your_document_id.html'
driver.get(url)
等待页面加载完成
driver.implicitly_wait(10)
检查是否出现验证码
captcha_image = driver.find_element(By.ID, 'captcha_image_id')
if captcha_image:
captcha_image_path = 'path/to/captcha_image.png'
captcha_image.screenshot(captcha_image_path)
captcha_code = recognize_captcha(captcha_image_path)
captcha_input = driver.find_element(By.ID, 'captcha_input_id')
captcha_input.send_keys(captcha_code)
submit_button = driver.find_element(By.ID, 'captcha_submit_button_id')
submit_button.click()
driver.implicitly_wait(10)
提取文档内容
content = driver.find_elements(By.CLASS_NAME, 'reader-word-layer')
for section in content:
print(section.text)
关闭浏览器
driver.quit()
四、解析和提取文档内容
在成功获取到百度文档页面内容后,下一步是解析和提取文档中的具体内容。根据百度文档的HTML结构,可以使用BeautifulSoup或Selenium定位到具体的文档内容元素,并提取其中的文本。
import requests
from bs4 import BeautifulSoup
发送HTTP请求,获取页面内容
url = 'https://wenku.baidu.com/view/your_document_id.html'
response = requests.get(url)
response.encoding = 'utf-8'
解析HTML页面
soup = BeautifulSoup(response.text, 'html.parser')
提取文档内容
content = soup.find_all('div', class_='reader-word-layer')
document_text = ''
for section in content:
document_text += section.get_text() + '\n'
保存文档内容到文件
with open('document.txt', 'w', encoding='utf-8') as file:
file.write(document_text)
总结:
通过使用BeautifulSoup库解析HTML页面、模拟浏览器行为、处理验证码等技术,可以实现从百度文档中抓取数据。需要注意的是,抓取百度文档属于网络爬虫行为,请遵守相关法律法规和百度文档的使用协议,不要进行恶意抓取或滥用。
相关问答FAQs:
如何使用Python抓取百度文档的基本步骤是什么?
抓取百度文档通常需要使用Python的网络请求库,如requests以及数据解析库,如BeautifulSoup。首先,您需要发送一个HTTP请求以获取文档的HTML内容,然后使用BeautifulSoup解析HTML,提取所需的数据。建议在抓取前了解百度文档的网页结构,以便更好地定位需要抓取的信息。
抓取百度文档时需要注意哪些法律和道德问题?
在抓取百度文档时,务必遵循网站的使用条款和条件。确保您抓取的数据不侵犯他人的版权或隐私权。某些情况下,您可能需要获取文档作者的许可,尤其是在使用数据进行商业用途时。此外,建议设置合理的请求间隔,以避免对网站造成过大的负担。
是否有可用的Python库专门用于抓取百度文档?
尽管没有专门针对百度文档的库,但可以使用一些通用的网络抓取库来实现这个目的。比如,Scrapy是一个强大的框架,适合于大规模抓取任务。同时,Pandas可以帮助您整理和分析抓取到的数据。结合这些工具,您可以有效地抓取并处理百度文档中的信息。
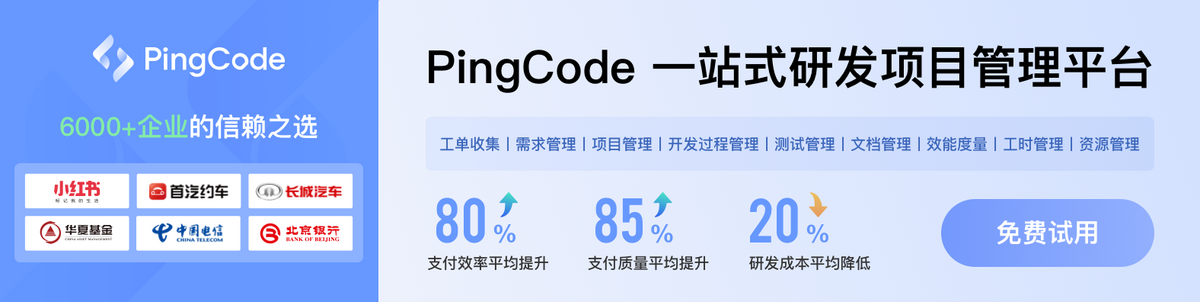