Python 爬虫如何模拟网页请求超时
在进行网页爬虫时,模拟网页请求超时、提高爬虫的稳定性、处理异常情况是非常重要的。其中,模拟网页请求超时可以帮助我们测试爬虫在面对不稳定网络或服务器响应缓慢时的表现。具体实现可以通过设置请求超时、捕捉异常并处理等方式进行。设置请求超时是最常用的方法,下面我们将详细讲解如何在 Python 中实现这些操作。
一、设置请求超时
使用 Python 中的 requests 库进行网络请求时,可以通过设置超时时间来模拟请求超时。超时时间可以通过 timeout
参数来指定,这个参数可以是一个浮点数(以秒为单位),也可以是一个元组,分别指定连接和读取的超时时间。
import requests
try:
response = requests.get('https://example.com', timeout=5)
response.raise_for_status()
print(response.text)
except requests.exceptions.Timeout:
print('The request timed out')
except requests.exceptions.RequestException as e:
print(f'An error occurred: {e}')
在上述代码中,我们通过 timeout=5
参数将请求的超时时间设置为5秒。如果超过这个时间服务器仍未响应,请求将会抛出 requests.exceptions.Timeout
异常,我们可以捕捉并处理这个异常。
二、处理异常情况
在进行网络请求时,除了请求超时外,还可能会遇到其他异常情况,如连接错误、HTTP 错误等。我们可以通过捕捉这些异常来提高爬虫的稳定性。
import requests
def fetch_url(url, timeout=5):
try:
response = requests.get(url, timeout=timeout)
response.raise_for_status()
return response.text
except requests.exceptions.Timeout:
print(f'The request to {url} timed out')
except requests.exceptions.HTTPError as err:
print(f'HTTP error occurred: {err}')
except requests.exceptions.RequestException as err:
print(f'An error occurred: {err}')
return None
content = fetch_url('https://example.com')
if content:
print(content)
在上述代码中,我们定义了一个 fetch_url
函数,该函数会捕捉并处理不同类型的异常,并返回网页内容或 None
。
三、使用重试机制
为了提高爬虫的稳定性,我们还可以使用重试机制,在请求失败时进行多次尝试。可以使用 requests
库的 Session
对象和 requests.adapters.HTTPAdapter
来实现重试机制。
import requests
from requests.adapters import HTTPAdapter
from requests.packages.urllib3.util.retry import Retry
def fetch_url_with_retry(url, retries=3, backoff_factor=0.3):
session = requests.Session()
retry = Retry(
total=retries,
read=retries,
connect=retries,
backoff_factor=backoff_factor,
status_forcelist=[500, 502, 503, 504]
)
adapter = HTTPAdapter(max_retries=retry)
session.mount('http://', adapter)
session.mount('https://', adapter)
try:
response = session.get(url)
response.raise_for_status()
return response.text
except requests.exceptions.RequestException as err:
print(f'An error occurred: {err}')
return None
content = fetch_url_with_retry('https://example.com')
if content:
print(content)
在上述代码中,我们使用 requests.Session
对象和 HTTPAdapter
来配置重试机制。Retry
类允许我们设置重试次数、退避因子以及需要重试的 HTTP 状态码。当请求失败时,程序会按照设定的重试策略进行多次尝试。
四、设置代理和用户代理
在进行网页爬虫时,使用代理和用户代理可以帮助我们避免被目标网站封禁。以下是如何设置代理和用户代理的示例:
import requests
proxies = {
'http': 'http://10.10.10.10:8000',
'https': 'http://10.10.10.10:8000',
}
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
try:
response = requests.get('https://example.com', proxies=proxies, headers=headers, timeout=5)
response.raise_for_status()
print(response.text)
except requests.exceptions.RequestException as e:
print(f'An error occurred: {e}')
在上述代码中,我们通过 proxies
参数设置了 HTTP 和 HTTPS 代理,通过 headers
参数设置了用户代理。在进行网络请求时,这些设置将帮助我们伪装成正常用户,减少被封禁的风险。
五、解析网页内容
在获取网页内容后,我们通常需要对其进行解析。可以使用 BeautifulSoup 库进行 HTML 解析。以下是一个简单的示例:
import requests
from bs4 import BeautifulSoup
def fetch_and_parse(url, timeout=5):
try:
response = requests.get(url, timeout=timeout)
response.raise_for_status()
soup = BeautifulSoup(response.text, 'html.parser')
return soup
except requests.exceptions.RequestException as e:
print(f'An error occurred: {e}')
return None
soup = fetch_and_parse('https://example.com')
if soup:
print(soup.prettify())
在上述代码中,我们使用 BeautifulSoup
将网页内容解析为 HTML 树,并使用 prettify
方法打印出格式化的 HTML 内容。
六、并发请求
为了提高爬虫的效率,我们可以使用并发请求。可以使用 concurrent.futures
模块进行并发请求。以下是一个简单的示例:
import requests
from concurrent.futures import ThreadPoolExecutor, as_completed
def fetch_url(url, timeout=5):
try:
response = requests.get(url, timeout=timeout)
response.raise_for_status()
return response.text
except requests.exceptions.RequestException as e:
print(f'An error occurred: {e}')
return None
urls = ['https://example.com', 'https://example.org', 'https://example.net']
with ThreadPoolExecutor(max_workers=5) as executor:
future_to_url = {executor.submit(fetch_url, url): url for url in urls}
for future in as_completed(future_to_url):
url = future_to_url[future]
try:
data = future.result()
if data:
print(f'Content from {url} retrieved successfully')
except Exception as e:
print(f'An error occurred when fetching {url}: {e}')
在上述代码中,我们使用 ThreadPoolExecutor
创建了一个线程池,并提交了多个并发请求。通过 as_completed
方法,我们可以按完成顺序处理每个请求的结果。
七、总结
在进行 Python 爬虫时,模拟网页请求超时、处理异常情况、使用重试机制、设置代理和用户代理、解析网页内容以及进行并发请求是提高爬虫稳定性和效率的关键。通过合理设置和处理这些方面,我们可以构建一个高效、稳定的爬虫系统。在实际应用中,我们还需要根据具体需求进行优化和调整,以应对不同的网络环境和目标网站的特殊情况。
相关问答FAQs:
如何在Python爬虫中设置请求超时的时间?
在Python爬虫中,可以使用requests
库中的timeout
参数来设置请求超时的时间。例如,当调用requests.get()
或requests.post()
时,可以传入timeout
参数,以秒为单位指定超时时间。如果请求超过指定的时间,程序将抛出requests.exceptions.Timeout
异常。
遇到请求超时后该如何处理?
当请求超时时,可以通过异常处理机制捕获requests.exceptions.Timeout
异常,并进行适当的处理。例如,可以重试请求、记录日志或返回默认值等。使用try...except
结构可以有效地管理超时情况,确保程序稳定运行。
使用Python爬虫时,如何提高请求的成功率?
提高请求成功率的方式有很多。可以使用代理服务器来分散请求,避免被目标网站封锁。设置合理的请求间隔时间,以防止过于频繁的请求导致被限制。此外,使用重试机制(例如,retrying
库或requests
的Session
对象)可以在请求失败时自动重试,增加成功的机会。
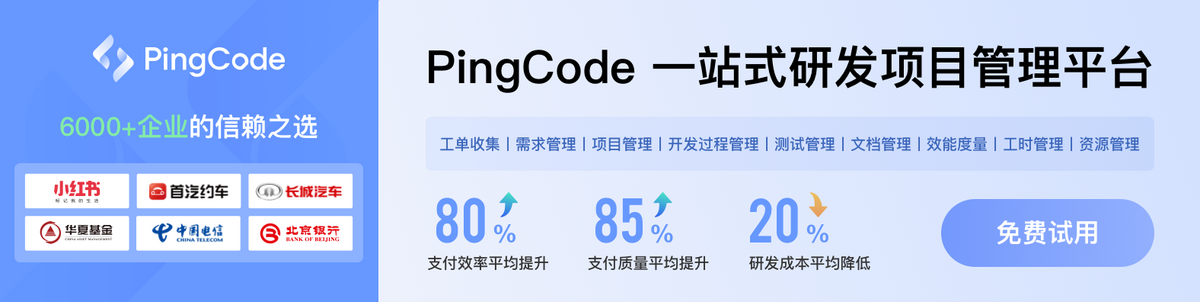