判断网页是否存在的核心方法包括:使用requests库发送HTTP请求、检查响应状态码、处理异常情况。 其中,使用requests库发送HTTP请求是最常用的方法,通过发送GET请求并检查响应状态码来判断网页是否存在。例如,状态码200表示网页存在,而404表示网页不存在。下面将详细讲解如何使用这些方法来判断网页的存在性。
一、使用requests库判断网页是否存在
使用requests库发送HTTP请求是判断网页是否存在的常用方法之一。以下是具体步骤:
-
安装requests库:
pip install requests
-
发送GET请求并检查响应状态码:
import requests
def check_url(url):
try:
response = requests.get(url)
if response.status_code == 200:
print(f"URL '{url}' exists.")
else:
print(f"URL '{url}' does not exist. Status code: {response.status_code}")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
url = "https://www.example.com"
check_url(url)
详细描述:
在上述代码中,我们首先导入requests库,然后定义一个函数check_url,该函数接受一个URL作为参数。我们使用requests.get方法发送GET请求,并通过response.status_code获取响应状态码。如果状态码是200,表示网页存在;否则,网页不存在。我们还捕获了请求异常,以处理可能的网络错误。
二、使用HEAD请求提高效率
有时我们只需要检查网页是否存在,而不需要获取网页内容。这时可以使用HEAD请求,它只请求响应头部信息,提高效率。
import requests
def check_url_head(url):
try:
response = requests.head(url)
if response.status_code == 200:
print(f"URL '{url}' exists.")
else:
print(f"URL '{url}' does not exist. Status code: {response.status_code}")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
url = "https://www.example.com"
check_url_head(url)
在上述代码中,requests.head方法只请求响应头部信息,相比GET请求效率更高。
三、处理重定向情况
有些网页可能会重定向到其他URL。我们需要处理这种情况,确保判断网页是否存在时考虑重定向。
import requests
def check_url_with_redirect(url):
try:
response = requests.get(url, allow_redirects=True)
if response.status_code == 200:
print(f"URL '{response.url}' exists.")
else:
print(f"URL '{response.url}' does not exist. Status code: {response.status_code}")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
url = "http://example.com"
check_url_with_redirect(url)
在上述代码中,我们将allow_redirects参数设置为True,允许处理重定向。最终判断重定向后的URL是否存在。
四、检测URL有效性
有时输入的URL可能无效,例如格式错误。我们可以使用正则表达式检查URL格式是否正确。
import re
import requests
def is_valid_url(url):
regex = re.compile(
r'^(?:http|ftp)s?://' # http:// or https://
r'(?:(?:[A-Z0-9](?:[A-Z0-9-]{0,61}[A-Z0-9])?\.)+(?:[A-Z]{2,6}\.?|[A-Z0-9-]{2,}\.?)|' # domain...
r'localhost|' # localhost...
r'\d{1,3}\.\d{1,3}\.\d{1,3}\.\d{1,3}|' # ...or ipv4
r'\[?[A-F0-9]*:[A-F0-9:]+\]?)' # ...or ipv6
r'(?::\d+)?' # optional port
r'(?:/?|[/?]\S+)$', re.IGNORECASE)
return re.match(regex, url) is not None
def check_url(url):
if is_valid_url(url):
try:
response = requests.get(url)
if response.status_code == 200:
print(f"URL '{url}' exists.")
else:
print(f"URL '{url}' does not exist. Status code: {response.status_code}")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
else:
print(f"Invalid URL: {url}")
url = "https://www.example.com"
check_url(url)
在上述代码中,我们使用正则表达式检查URL格式是否正确,确保输入的URL有效。
五、处理HTTPS和HTTP协议
有些网页可能只支持HTTP或HTTPS协议。我们可以尝试使用两种协议检查网页是否存在。
import requests
def check_url_with_protocols(url):
try:
response = requests.get(url)
if response.status_code == 200:
print(f"URL '{url}' exists.")
else:
print(f"URL '{url}' does not exist. Status code: {response.status_code}")
except requests.exceptions.RequestException:
# Try with HTTP if HTTPS fails
if url.startswith('https://'):
url = url.replace('https://', 'http://')
try:
response = requests.get(url)
if response.status_code == 200:
print(f"URL '{url}' exists.")
else:
print(f"URL '{url}' does not exist. Status code: {response.status_code}")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
url = "https://www.example.com"
check_url_with_protocols(url)
在上述代码中,如果HTTPS请求失败,我们尝试使用HTTP协议检查网页是否存在。
六、处理不同的HTTP状态码
除了200和404状态码外,还有其他状态码需要处理。例如,403表示禁止访问,500表示服务器内部错误。我们可以根据不同状态码提供具体的提示信息。
import requests
def check_url_with_status(url):
try:
response = requests.get(url)
if response.status_code == 200:
print(f"URL '{url}' exists.")
elif response.status_code == 403:
print(f"URL '{url}' is forbidden.")
elif response.status_code == 404:
print(f"URL '{url}' does not exist.")
elif response.status_code == 500:
print(f"URL '{url}' has internal server error.")
else:
print(f"URL '{url}' returned status code: {response.status_code}")
except requests.exceptions.RequestException as e:
print(f"An error occurred: {e}")
url = "https://www.example.com"
check_url_with_status(url)
在上述代码中,我们根据不同的状态码提供具体的提示信息,帮助用户更好地了解网页状态。
七、使用异步请求提高效率
当需要检查多个URL时,使用异步请求可以提高效率。我们可以使用aiohttp库实现异步请求。
-
安装aiohttp库:
pip install aiohttp
-
使用异步请求检查多个URL:
import aiohttp
import asyncio
async def fetch(session, url):
try:
async with session.get(url) as response:
if response.status == 200:
print(f"URL '{url}' exists.")
else:
print(f"URL '{url}' does not exist. Status code: {response.status}")
except aiohttp.ClientError as e:
print(f"An error occurred: {e}")
async def check_urls(urls):
async with aiohttp.ClientSession() as session:
tasks = [fetch(session, url) for url in urls]
await asyncio.gather(*tasks)
urls = ["https://www.example.com", "https://www.nonexistenturl.com"]
asyncio.run(check_urls(urls))
在上述代码中,我们使用aiohttp库实现异步请求,检查多个URL的存在性。这样可以显著提高效率,尤其是在需要检查大量URL时。
八、总结
通过上述方法,我们可以有效地判断网页是否存在。这些方法包括使用requests库发送HTTP请求、使用HEAD请求提高效率、处理重定向情况、检测URL有效性、处理HTTPS和HTTP协议、处理不同的HTTP状态码以及使用异步请求提高效率。希望这些方法对您有所帮助,在实际应用中能够更好地判断网页的存在性。
相关问答FAQs:
如何使用Python3检查网页是否有效?
使用Python3可以通过发送HTTP请求来检查网页是否有效。常用的库有requests
。你可以使用requests.get()
方法向目标网址发送请求,并根据返回的状态码判断网页是否存在。状态码200表示网页正常存在,404则表示网页不存在。
在Python3中如何处理网页请求的异常?
在进行网页请求时,可能会遇到各种异常,例如网络问题或无效的URL。可以使用try-except
结构捕获这些异常,以确保程序的稳定性。例如,如果使用requests.get()
方法时发生异常,可以捕获requests.exceptions.RequestException
,并进行适当的处理,比如记录日志或返回友好的提示信息。
使用Python3检查多个网页是否存在的最佳方法是什么?
如果需要检查多个网页的存在性,可以将所有网址存储在一个列表中,并使用循环遍历每个网址。结合requests
库,你可以为每个网址发送请求,并记录下它们的状态。为了提高效率,可以考虑使用异步请求库如aiohttp
,这样可以同时处理多个请求,从而加快检查速度。
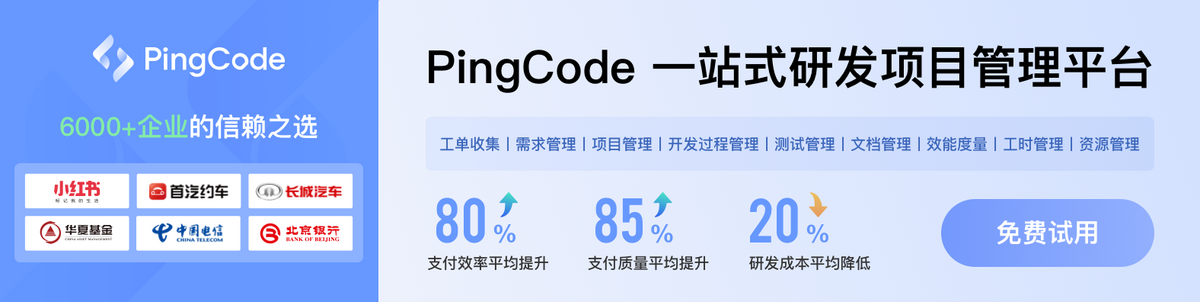