用Python做全网搜索器的方法包括使用爬虫技术、解析网页内容、存储和检索数据、实现搜索算法等。 在这之中,爬虫技术是最基础和关键的一步。接下来,我将详细描述如何实现一个全网搜索器。
一、爬虫技术
Python中的爬虫技术主要通过库如requests
和BeautifulSoup
来实现。requests
库用来发送网络请求,而BeautifulSoup
库用来解析HTML和XML文档。
- 发送网络请求:
使用
requests
库可以方便地发送HTTP请求,获取网页内容。
import requests
url = "http://example.com"
response = requests.get(url)
html_content = response.text
- 解析网页内容:
使用
BeautifulSoup
库可以解析HTML内容,提取有用的信息。
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
titles = soup.find_all('h1')
for title in titles:
print(title.get_text())
二、解析网页内容
在抓取网页内容之后,需要对其进行解析,提取出有用的数据。解析网页时,可以使用BeautifulSoup
、lxml
等库来处理HTML内容。
- 使用BeautifulSoup解析内容:
BeautifulSoup是一个强大的HTML解析库,可以轻松地解析HTML文档并提取数据。
from bs4 import BeautifulSoup
html_doc = """
<html><head><title>The Dormouse's story</title></head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
print(soup.prettify())
- 提取特定数据:
通过BeautifulSoup的
find_all
等方法,可以提取特定标签内的数据。
links = soup.find_all('a')
for link in links:
print(link.get('href'))
三、存储和检索数据
在获取到有用的数据之后,需要将其存储起来,以便后续检索。可以使用关系型数据库(如MySQL、PostgreSQL)或NoSQL数据库(如MongoDB)来存储数据。
- 使用SQLite存储数据:
SQLite是一个轻量级的关系型数据库,适合小型应用程序使用。
import sqlite3
conn = sqlite3.connect('example.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS search_data
(url TEXT, title TEXT, content TEXT)''')
c.execute("INSERT INTO search_data VALUES ('http://example.com', 'Example Title', 'Example Content')")
conn.commit()
conn.close()
- 使用MongoDB存储数据:
MongoDB是一种NoSQL数据库,适合存储大量的非结构化数据。
from pymongo import MongoClient
client = MongoClient('localhost', 27017)
db = client['search_db']
collection = db['search_data']
data = {'url': 'http://example.com', 'title': 'Example Title', 'content': 'Example Content'}
collection.insert_one(data)
四、实现搜索算法
在数据存储完成后,需要实现搜索算法来检索数据。可以使用简单的字符串匹配算法,或使用更复杂的全文检索技术(如ElasticSearch)。
- 字符串匹配算法:
简单的字符串匹配算法可以直接在数据库中进行查询。
import sqlite3
conn = sqlite3.connect('example.db')
c = conn.cursor()
keyword = 'Example'
c.execute("SELECT * FROM search_data WHERE content LIKE ?", ('%' + keyword + '%',))
results = c.fetchall()
for result in results:
print(result)
conn.close()
- 使用ElasticSearch进行全文检索:
ElasticSearch是一个分布式搜索引擎,适合处理大规模的全文检索需求。
from elasticsearch import Elasticsearch
es = Elasticsearch()
创建索引
es.indices.create(index='search_index', ignore=400)
插入数据
doc = {'url': 'http://example.com', 'title': 'Example Title', 'content': 'Example Content'}
es.index(index='search_index', id=1, body=doc)
搜索数据
results = es.search(index='search_index', body={'query': {'match': {'content': 'Example'}}})
for result in results['hits']['hits']:
print(result['_source'])
五、优化和扩展
随着数据量的增加和应用需求的变化,可以对全网搜索器进行优化和扩展。
- 分布式爬虫:
为了提高爬取效率,可以使用分布式爬虫框架(如Scrapy)来实现多线程或多进程的爬取。
from scrapy import Spider
class ExampleSpider(Spider):
name = 'example'
start_urls = ['http://example.com']
def parse(self, response):
for title in response.css('h1::text'):
yield {'title': title.get()}
运行爬虫
from scrapy.crawler import CrawlerProcess
process = CrawlerProcess()
process.crawl(ExampleSpider)
process.start()
- 数据清洗和预处理:
在数据存储之前,可以对爬取的数据进行清洗和预处理,以提高数据质量。
def clean_data(data):
# 去除HTML标签
cleaned_data = BeautifulSoup(data, 'html.parser').get_text()
# 去除多余的空白字符
cleaned_data = ' '.join(cleaned_data.split())
return cleaned_data
- 提升搜索算法:
可以使用更高级的自然语言处理技术(如TF-IDF、Word2Vec)来提升搜索算法的准确性。
from sklearn.feature_extraction.text import TfidfVectorizer
documents = ['Example Content', 'Another Example Content']
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(documents)
print(tfidf_matrix)
通过以上步骤,可以使用Python实现一个基本的全网搜索器。随着需求的增加和技术的发展,可以不断对其进行优化和扩展,以满足更多的应用场景。
相关问答FAQs:
如何开始使用Python构建全网搜索器?
要开始构建全网搜索器,首先需要了解Python的基本语法和相关库。你可以使用如Beautiful Soup和Scrapy进行网页抓取,利用requests库进行HTTP请求。为了处理和存储数据,SQLite或MongoDB等数据库可以帮助你管理抓取到的信息。此外,学习如何使用正则表达式来提取特定数据也是非常有帮助的。
构建全网搜索器需要哪些工具和库?
构建全网搜索器通常需要一些关键的工具和库。常用的Python库包括Beautiful Soup(用于解析HTML和XML文档)、Scrapy(一个强大的网页抓取框架)、requests(用于发送HTTP请求)以及pandas(用于数据处理)。此外,使用Elasticsearch可以帮助你实现高效的搜索功能,建议对其进行了解和学习。
如何处理抓取到的数据以提高搜索效果?
抓取到的数据往往需要进行清洗和整理,以提高搜索效果。可以使用pandas库来处理数据,去除重复项、空值和不必要的信息。对文本内容进行分词和去停用词处理可以帮助提高搜索的相关性。此外,建立索引和使用自然语言处理技术(如TF-IDF)有助于改善搜索结果的质量和速度。
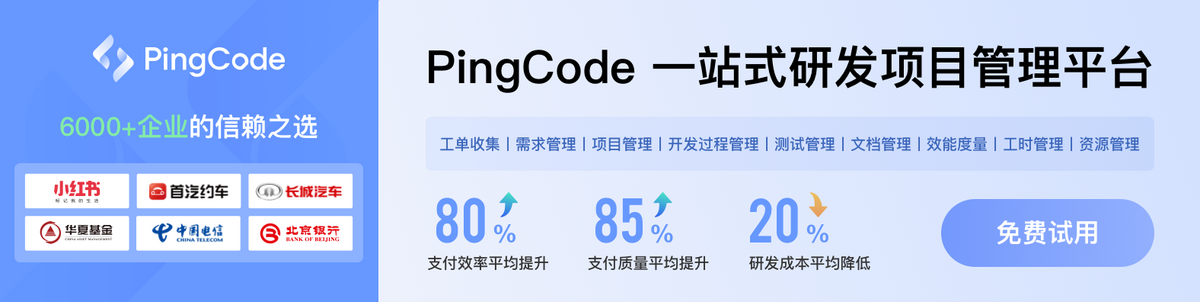