Python限制函数执行时间的方法有多种,包括使用信号处理、线程、装饰器等方式。其中一个常见且有效的方法是使用线程和信号处理来实现。下面将详细讲解其中一种方法,并提供代码示例。
一、使用信号处理限制函数执行时间
在Unix-like系统中,可以使用signal
模块来设置一个定时器,如果函数在规定时间内没有完成执行,可以发出一个信号来终止该函数。这种方法简单高效,但只能在Unix-like系统上使用。
1.1 定义超时信号处理器
首先,我们需要定义一个超时信号处理器。当我们的定时器到期时,这个处理器将被调用。
import signal
class TimeoutException(Exception):
pass
def timeout_handler(signum, frame):
raise TimeoutException("Function execution timed out")
1.2 设置定时器
接下来,我们可以设置一个定时器,并在函数执行前启动它。
def limited_time_function(timeout):
def decorator(func):
def wrapper(*args, kwargs):
# 设置信号处理器
signal.signal(signal.SIGALRM, timeout_handler)
# 设置定时器
signal.alarm(timeout)
try:
result = func(*args, kwargs)
finally:
# 取消定时器
signal.alarm(0)
return result
return wrapper
return decorator
1.3 使用装饰器限制函数执行时间
现在,我们可以使用这个装饰器来限制任意函数的执行时间。
@limited_time_function(5)
def long_running_function():
import time
time.sleep(10)
return "Finished"
try:
result = long_running_function()
print(result)
except TimeoutException as e:
print(e)
在上述代码中,如果long_running_function
在5秒内没有完成,它将被终止,并抛出TimeoutException
异常。
二、使用线程限制函数执行时间
在跨平台的情况下,可以使用线程来限制函数的执行时间。虽然这种方法稍微复杂一些,但它可以在Windows和Unix-like系统上运行。
2.1 定义超时装饰器
首先,我们定义一个超时装饰器,它将在新的线程中运行目标函数,并监控它的执行时间。
import threading
import functools
class ThreadTimeoutException(Exception):
pass
def thread_timeout(timeout):
def decorator(func):
@functools.wraps(func)
def wrapper(*args, kwargs):
result = [ThreadTimeoutException("Function execution timed out")]
def target():
try:
result[0] = func(*args, kwargs)
except Exception as e:
result[0] = e
thread = threading.Thread(target=target)
thread.start()
thread.join(timeout)
if isinstance(result[0], Exception):
raise result[0]
return result[0]
return wrapper
return decorator
2.2 使用线程超时装饰器
现在,我们可以使用这个线程超时装饰器来限制函数的执行时间。
@thread_timeout(5)
def long_running_function():
import time
time.sleep(10)
return "Finished"
try:
result = long_running_function()
print(result)
except ThreadTimeoutException as e:
print(e)
在上述代码中,如果long_running_function
在5秒内没有完成,它将被终止,并抛出ThreadTimeoutException
异常。
三、使用concurrent.futures模块限制函数执行时间
Python的concurrent.futures
模块提供了一个高层次的接口来管理线程和进程池。我们可以使用它来实现函数执行时间的限制。
3.1 使用ThreadPoolExecutor
ThreadPoolExecutor
是一个线程池管理器,可以用来并发执行函数,并通过timeout
参数来限制函数执行时间。
from concurrent.futures import ThreadPoolExecutor, TimeoutError
def limited_time_function(func, timeout, *args, kwargs):
with ThreadPoolExecutor(max_workers=1) as executor:
future = executor.submit(func, *args, kwargs)
try:
result = future.result(timeout=timeout)
except TimeoutError:
raise TimeoutException("Function execution timed out")
return result
3.2 使用ThreadPoolExecutor限制函数执行时间
现在,我们可以使用ThreadPoolExecutor
来限制函数的执行时间。
def long_running_function():
import time
time.sleep(10)
return "Finished"
try:
result = limited_time_function(long_running_function, 5)
print(result)
except TimeoutException as e:
print(e)
在上述代码中,如果long_running_function
在5秒内没有完成,它将被终止,并抛出TimeoutException
异常。
四、总结
限制函数执行时间的方法有多种选择,包括使用信号处理、线程、以及concurrent.futures
模块。每种方法都有其优缺点,选择合适的方法取决于具体的应用场景和系统环境。
- 信号处理方法简单高效,但仅适用于Unix-like系统。
- 线程方法跨平台,但实现稍微复杂,可能会影响性能。
concurrent.futures
模块提供了高层次的接口,使用方便,适用于大多数情况。
希望通过本文的介绍,您能够找到适合自己的方法来限制函数的执行时间,从而提高程序的稳定性和可靠性。
相关问答FAQs:
如何在Python中设置函数的最大执行时间?
在Python中,可以使用signal
模块来限制函数的执行时间。通过设置一个定时器,您可以在指定时间内强制停止函数的执行。需要注意的是,这种方法在Windows系统上可能不兼容。此外,使用threading
模块也可以实现类似的功能,您可以在一个单独的线程中运行函数,并在主线程中监控其执行时间。
如何处理超时函数引发的异常?
当您限制函数的执行时间时,可能会遇到超时引发的异常。为了有效地处理这种情况,可以使用try...except
语句来捕获超时异常,并在异常处理块中执行清理操作或返回默认值。这种方式能够确保程序不会因为未处理的异常而崩溃,增加了代码的健壮性。
是否有现成的库可以帮助限制函数执行时间?
是的,有一些第三方库可以帮助您更轻松地限制函数的执行时间。例如,timeout_decorator
库提供了一个简单的装饰器,可以直接应用于函数上,从而指定最大执行时间。如果函数超时,这个装饰器会抛出TimeoutError
异常。使用这样的库可以减少代码的复杂性,让您的实现更加简洁明了。
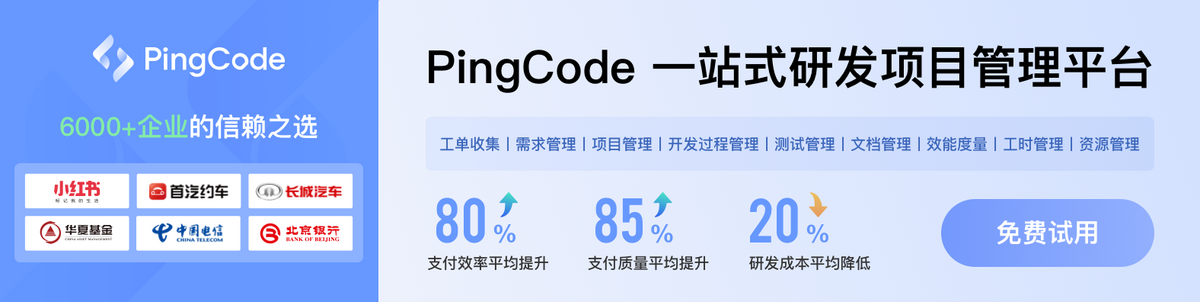