在Python中编写if嵌套语句时,可以通过使用if、elif和else语句在其他条件语句中进行嵌套。这样可以检查多个条件,并在不同条件下执行不同的代码块。、嵌套if语句可以提高代码的灵活性和精确性、便于处理复杂的逻辑、简化代码的编写和阅读。下面将详细描述如何在Python中编写if嵌套语句,并介绍一些常见的应用场景和注意事项。
一、嵌套if语句的基本概念
在Python中,if语句用于判断某个条件是否为真,如果为真则执行相应的代码块。嵌套if语句是指在一个if语句的代码块内,再次使用if、elif或else语句进行条件判断。这种嵌套结构可以用于处理多个相关联的条件,并根据不同的条件组合执行不同的操作。
例如:
age = 20
if age >= 18:
print("You are an adult.")
if age >= 21:
print("You can also drink alcohol.")
else:
print("You cannot drink alcohol yet.")
else:
print("You are a minor.")
二、嵌套if语句的应用场景
1、处理多级分类
嵌套if语句常用于处理多级分类问题。例如,根据用户的年龄进行分类,并进一步判断其他条件:
age = 25
membership_status = "premium"
if age >= 18:
if membership_status == "premium":
print("You are an adult with premium membership.")
elif membership_status == "standard":
print("You are an adult with standard membership.")
else:
print("You are an adult with no membership.")
else:
print("You are a minor.")
2、处理复杂的业务逻辑
在实际项目中,嵌套if语句可以用于处理复杂的业务逻辑。例如,根据用户的购买金额和会员等级计算折扣:
purchase_amount = 150
membership_level = "gold"
if purchase_amount >= 100:
if membership_level == "gold":
discount = 0.2
elif membership_level == "silver":
discount = 0.1
else:
discount = 0.05
else:
discount = 0
final_amount = purchase_amount * (1 - discount)
print(f"The final amount to be paid is: {final_amount}")
三、嵌套if语句的注意事项
1、避免过深的嵌套
虽然嵌套if语句可以处理复杂的逻辑,但过深的嵌套会导致代码难以阅读和维护。建议尽量避免超过三层的嵌套,通过拆分函数或使用其他控制结构(如循环、字典映射等)来简化代码。
2、保持代码的可读性
在编写嵌套if语句时,保持代码的可读性非常重要。可以通过合理的缩进、注释和命名来提高代码的可读性。例如:
age = 30
is_student = False
if age >= 18:
if is_student:
print("You are an adult student.")
else:
print("You are an adult non-student.")
else:
print("You are a minor.")
四、嵌套if语句的替代方案
在某些情况下,可以使用其他控制结构来替代嵌套if语句,从而简化代码。
1、使用逻辑运算符
逻辑运算符(如and、or、not)可以用于简化多个条件的判断。例如:
age = 25
membership_status = "premium"
if age >= 18 and membership_status == "premium":
print("You are an adult with premium membership.")
elif age >= 18 and membership_status == "standard":
print("You are an adult with standard membership.")
elif age >= 18:
print("You are an adult with no membership.")
else:
print("You are a minor.")
2、使用字典映射
字典映射可以用于替代多重嵌套的条件判断。例如,根据用户的购买金额和会员等级计算折扣:
purchase_amount = 150
membership_level = "gold"
discounts = {
"gold": 0.2,
"silver": 0.1,
"none": 0.05
}
if purchase_amount >= 100:
discount = discounts.get(membership_level, 0.05)
else:
discount = 0
final_amount = purchase_amount * (1 - discount)
print(f"The final amount to be paid is: {final_amount}")
五、嵌套if语句的调试技巧
在编写和调试嵌套if语句时,可以使用以下技巧来提高效率:
1、打印调试信息
在条件判断和代码块内添加打印语句,输出相关变量的值和状态,帮助定位问题。例如:
age = 25
membership_status = "premium"
if age >= 18:
print("Age check passed.")
if membership_status == "premium":
print("Membership status: premium")
print("You are an adult with premium membership.")
elif membership_status == "standard":
print("Membership status: standard")
print("You are an adult with standard membership.")
else:
print("Membership status: none")
print("You are an adult with no membership.")
else:
print("You are a minor.")
2、使用调试器
使用调试器(如Python自带的pdb模块或集成开发环境中的调试工具)逐步执行代码,检查变量的值和程序的执行流程,帮助定位和解决问题。
六、总结
在Python中,if嵌套语句是一种常用的控制结构,用于处理多个相关联的条件。通过合理使用嵌套if语句,可以提高代码的灵活性和精确性。然而,过深的嵌套会导致代码难以阅读和维护,因此需要注意保持代码的可读性,并尽量避免超过三层的嵌套。在某些情况下,可以使用逻辑运算符、字典映射等替代嵌套if语句,从而简化代码。此外,在编写和调试嵌套if语句时,可以通过打印调试信息和使用调试器来提高效率。希望本文对您在编写Python代码时处理if嵌套语句有所帮助。
相关问答FAQs:
什么是Python中的if嵌套语句?
在Python编程中,if嵌套语句是指在一个if语句内部再写一个或多个if语句。这种结构可以让程序根据多层条件进行判断,适用于需要更复杂决策的场景。例如,您可以先判断一个条件,如果该条件成立,再进行进一步的条件判断。
如何在Python中编写if嵌套语句的示例?
编写if嵌套语句时,可以使用如下结构:
if condition1:
# 执行某些操作
if condition2:
# 执行其他操作
在这个结构中,只有当condition1
为真时,condition2
才会被检查。这样的设计可以让您灵活处理复杂的逻辑。
使用if嵌套语句时需要注意哪些问题?
在使用if嵌套语句时,代码的可读性是一个重要的考虑因素。过多的嵌套可能会使代码变得复杂,难以维护。尽量保持嵌套层数在合理范围内,并考虑使用逻辑运算符(如and
、or
)来简化条件判断。同时,确保适当的缩进,以保持代码结构的清晰。
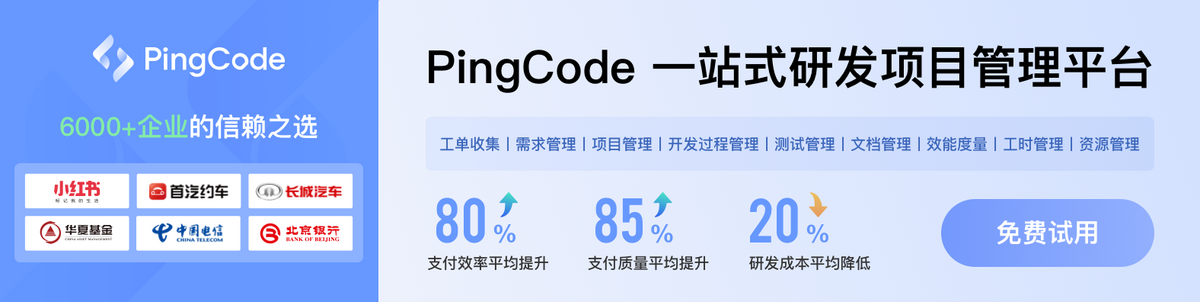