在Python中实现渐变背景色的方法包括使用图形库、生成渐变色数组、创建渐变色背景图、使用渐变色背景图进行绘制。 其中,使用图形库如Tkinter、PIL(Pillow)或Pygame生成渐变色背景图是一个详细的实现方法。以下将详细介绍如何使用这些库来实现渐变背景色。
一、使用Tkinter库
Tkinter是Python的标准GUI库,非常适合用于创建简单的图形用户界面。我们可以使用Tkinter来创建一个具有渐变背景色的窗口。
1. 安装Tkinter
Tkinter通常随Python一起安装。如果没有安装,可以使用以下命令进行安装:
pip install tk
2. 创建渐变背景
import tkinter as tk
def create_gradient(canvas, width, height, color1, color2):
gradient = []
for i in range(height):
ratio = i / height
r = int((1 - ratio) * color1[0] + ratio * color2[0])
g = int((1 - ratio) * color1[1] + ratio * color2[1])
b = int((1 - ratio) * color1[2] + ratio * color2[2])
color = f'#{r:02x}{g:02x}{b:02x}'
gradient.append(color)
canvas.create_line(0, i, width, i, fill=color)
return gradient
root = tk.Tk()
canvas = tk.Canvas(root, width=500, height=500)
canvas.pack()
color1 = (255, 0, 0) # Red
color2 = (0, 0, 255) # Blue
create_gradient(canvas, 500, 500, color1, color2)
root.mainloop()
二、使用PIL(Pillow)库
PIL是Python Imaging Library的缩写,Pillow是PIL的一个分支。它提供了多种图像处理功能。
1. 安装Pillow
pip install pillow
2. 创建渐变背景图像
from PIL import Image
def create_gradient_image(width, height, color1, color2, filename):
image = Image.new('RGB', (width, height))
for y in range(height):
ratio = y / height
r = int((1 - ratio) * color1[0] + ratio * color2[0])
g = int((1 - ratio) * color1[1] + ratio * color2[1])
b = int((1 - ratio) * color1[2] + ratio * color2[2])
for x in range(width):
image.putpixel((x, y), (r, g, b))
image.save(filename)
color1 = (255, 0, 0) # Red
color2 = (0, 0, 255) # Blue
create_gradient_image(500, 500, color1, color2, 'gradient.png')
三、使用Pygame库
Pygame是一个跨平台的Python模块,用于编写视频游戏。它包括计算机图形和声音库。
1. 安装Pygame
pip install pygame
2. 创建渐变背景
import pygame
def create_gradient(surface, width, height, color1, color2):
for y in range(height):
ratio = y / height
r = int((1 - ratio) * color1[0] + ratio * color2[0])
g = int((1 - ratio) * color1[1] + ratio * color2[1])
b = int((1 - ratio) * color1[2] + ratio * color2[2])
pygame.draw.line(surface, (r, g, b), (0, y), (width, y))
pygame.init()
screen = pygame.display.set_mode((500, 500))
color1 = (255, 0, 0) # Red
color2 = (0, 0, 255) # Blue
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
create_gradient(screen, 500, 500, color1, color2)
pygame.display.flip()
pygame.quit()
四、使用Matplotlib库
Matplotlib是一个绘图库,适用于Python编程语言及其数值数学扩展包NumPy。它提供了一个面向对象的API,可嵌入到各种应用程序中。
1. 安装Matplotlib
pip install matplotlib
2. 创建渐变背景
import matplotlib.pyplot as plt
import numpy as np
def create_gradient_image(width, height, color1, color2):
gradient = np.zeros((height, width, 3), dtype=np.uint8)
for y in range(height):
ratio = y / height
r = int((1 - ratio) * color1[0] + ratio * color2[0])
g = int((1 - ratio) * color1[1] + ratio * color2[1])
b = int((1 - ratio) * color1[2] + ratio * color2[2])
gradient[y, :, :] = (r, g, b)
return gradient
color1 = (255, 0, 0) # Red
color2 = (0, 0, 255) # Blue
gradient = create_gradient_image(500, 500, color1, color2)
plt.imshow(gradient)
plt.axis('off')
plt.show()
五、使用OpenCV库
OpenCV是一个开源的计算机视觉和机器学习软件库。它提供了大量的图像处理功能。
1. 安装OpenCV
pip install opencv-python
2. 创建渐变背景
import cv2
import numpy as np
def create_gradient_image(width, height, color1, color2):
gradient = np.zeros((height, width, 3), dtype=np.uint8)
for y in range(height):
ratio = y / height
r = int((1 - ratio) * color1[0] + ratio * color2[0])
g = int((1 - ratio) * color1[1] + ratio * color2[1])
b = int((1 - ratio) * color1[2] + ratio * color2[2])
gradient[y, :, :] = (r, g, b)
return gradient
color1 = (255, 0, 0) # Red
color2 = (0, 0, 255) # Blue
gradient = create_gradient_image(500, 500, color1, color2)
cv2.imshow('Gradient', gradient)
cv2.waitKey(0)
cv2.destroyAllWindows()
六、总结
在Python中实现渐变背景色的方法有很多,主要依赖于不同的图形库。Tkinter适用于简单的GUI应用,PIL(Pillow)适用于图像处理,Pygame适用于游戏开发,Matplotlib适用于数据可视化,OpenCV适用于计算机视觉和图像处理。根据具体需求选择合适的库,可以高效地实现渐变背景色效果。
通过上述不同方法的介绍,大家可以选择最适合自己项目需求的方式来实现渐变背景色。希望这篇文章能帮助你更好地理解和应用Python中的渐变背景色技术。
相关问答FAQs:
如何在Python中实现渐变背景色的效果?
在Python中,可以使用多种图形库来创建渐变背景色。常见的库如Tkinter、Pygame或Matplotlib都支持渐变效果。以Tkinter为例,您可以通过绘制多个矩形来模拟渐变,或者使用Canvas组件自定义背景颜色。此外,Pygame提供了填充渐变的功能,通过循环设置每个像素的颜色实现。
渐变背景色在Python项目中有哪些应用场景?
渐变背景色可以提升用户界面的视觉效果,常用于游戏开发、数据可视化和网页设计。在游戏中,渐变背景可以为场景提供层次感;在数据可视化中,渐变色可以帮助区分数据集的不同部分;而在网页设计中,渐变背景能够使整体风格更加现代和吸引用户注意。
使用Python制作渐变背景色时需要注意哪些事项?
在制作渐变背景时,需考虑渐变的颜色搭配和过渡效果,以确保视觉上的和谐。过于鲜艳的颜色可能会使界面显得杂乱,因此选择柔和的色调更为合适。此外,性能也是一个关键因素,尤其是在需要实时渲染的应用中,优化绘制的方式以提高效率是十分重要的。
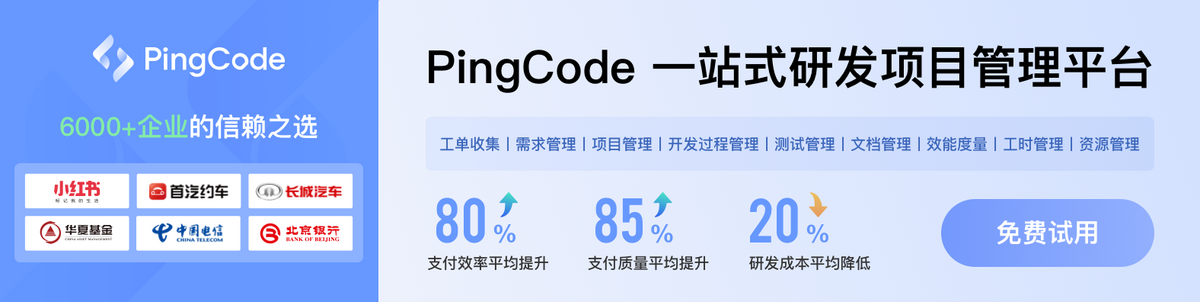