Python自动录入学生成绩的方法主要有:使用CSV文件、数据库和GUI应用程序。在本文中,我们将详细介绍这些方法,并提供相关代码示例和解释,以帮助你更好地理解和实现自动录入学生成绩的功能。
一、使用CSV文件
使用CSV文件是一种简单且常见的方法来自动录入学生成绩。CSV文件格式易于理解和操作,Python内置的CSV模块可以方便地读取和写入CSV文件。
读取和写入CSV文件
要读取CSV文件,可以使用以下代码:
import csv
def read_csv(file_path):
students = []
with open(file_path, mode='r', newline='') as file:
reader = csv.reader(file)
header = next(reader) # 读取表头
for row in reader:
students.append(row)
return students
file_path = 'students.csv'
students = read_csv(file_path)
print(students)
要写入CSV文件,可以使用以下代码:
import csv
def write_csv(file_path, data):
with open(file_path, mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Score']) # 写入表头
writer.writerows(data)
students = [['Alice', 85], ['Bob', 90], ['Charlie', 78]]
file_path = 'students.csv'
write_csv(file_path, students)
更新CSV文件中的成绩
以下是一个更新CSV文件中学生成绩的示例代码:
def update_student_score(file_path, student_name, new_score):
students = read_csv(file_path)
for student in students:
if student[0] == student_name:
student[1] = new_score
break
write_csv(file_path, students)
file_path = 'students.csv'
update_student_score(file_path, 'Alice', 95)
二、使用数据库
使用数据库是一种更为高效和可靠的方法来管理和录入学生成绩。常见的数据库包括SQLite、MySQL和PostgreSQL。这里,我们以SQLite为例,介绍如何使用Python中的sqlite3
模块来操作数据库。
创建数据库和表
首先,创建一个数据库并创建一个表来存储学生成绩:
import sqlite3
def create_database(db_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('''
CREATE TABLE IF NOT EXISTS students (
id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT NOT NULL,
score INTEGER NOT NULL
)
''')
conn.commit()
conn.close()
db_name = 'students.db'
create_database(db_name)
插入和更新学生成绩
插入新的学生成绩:
def insert_student(db_name, student_name, student_score):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('''
INSERT INTO students (name, score)
VALUES (?, ?)
''', (student_name, student_score))
conn.commit()
conn.close()
insert_student(db_name, 'Alice', 85)
insert_student(db_name, 'Bob', 90)
更新学生成绩:
def update_student_score(db_name, student_name, new_score):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('''
UPDATE students
SET score = ?
WHERE name = ?
''', (new_score, student_name))
conn.commit()
conn.close()
update_student_score(db_name, 'Alice', 95)
查询学生成绩
查询所有学生成绩:
def query_all_students(db_name):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('SELECT * FROM students')
students = cursor.fetchall()
conn.close()
return students
students = query_all_students(db_name)
print(students)
三、使用GUI应用程序
使用GUI应用程序可以提供更友好的用户界面来录入和管理学生成绩。这里,我们使用Python的tkinter
库来创建一个简单的GUI应用程序。
创建基本的GUI界面
以下是创建基本GUI界面的代码:
import tkinter as tk
from tkinter import messagebox
import sqlite3
db_name = 'students.db'
def create_database():
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('''
CREATE TABLE IF NOT EXISTS students (
id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT NOT NULL,
score INTEGER NOT NULL
)
''')
conn.commit()
conn.close()
def add_student(name, score):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('''
INSERT INTO students (name, score)
VALUES (?, ?)
''', (name, score))
conn.commit()
conn.close()
def update_student(name, score):
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('''
UPDATE students
SET score = ?
WHERE name = ?
''', (score, name))
conn.commit()
conn.close()
def show_students():
conn = sqlite3.connect(db_name)
cursor = conn.cursor()
cursor.execute('SELECT * FROM students')
students = cursor.fetchall()
conn.close()
return students
def add_student_callback():
name = name_entry.get()
score = score_entry.get()
if name and score:
try:
score = int(score)
add_student(name, score)
messagebox.showinfo("Success", "Student added successfully")
except ValueError:
messagebox.showerror("Error", "Score must be an integer")
else:
messagebox.showerror("Error", "Name and score are required")
def update_student_callback():
name = name_entry.get()
score = score_entry.get()
if name and score:
try:
score = int(score)
update_student(name, score)
messagebox.showinfo("Success", "Student updated successfully")
except ValueError:
messagebox.showerror("Error", "Score must be an integer")
else:
messagebox.showerror("Error", "Name and score are required")
def show_students_callback():
students = show_students()
students_str = "\n".join([f"{student[1]}: {student[2]}" for student in students])
messagebox.showinfo("Students", students_str)
create_database()
root = tk.Tk()
root.title("Student Management")
tk.Label(root, text="Name").grid(row=0)
tk.Label(root, text="Score").grid(row=1)
name_entry = tk.Entry(root)
score_entry = tk.Entry(root)
name_entry.grid(row=0, column=1)
score_entry.grid(row=1, column=1)
tk.Button(root, text="Add Student", command=add_student_callback).grid(row=2, column=0)
tk.Button(root, text="Update Student", command=update_student_callback).grid(row=2, column=1)
tk.Button(root, text="Show Students", command=show_students_callback).grid(row=3, column=0, columnspan=2)
root.mainloop()
这个简单的GUI应用程序允许用户添加、更新和查看学生成绩。通过tkinter库,你可以创建更复杂和丰富的用户界面,以满足更复杂的需求。
总结
本文介绍了三种Python自动录入学生成绩的方法:使用CSV文件、数据库和GUI应用程序。每种方法都有其优点和适用场景,具体选择哪种方法取决于你的实际需求和应用场景。通过本文的介绍和示例代码,希望你能够更好地理解和实现学生成绩的自动录入功能。
相关问答FAQs:
如何使用Python自动录入学生成绩?
使用Python进行自动录入学生成绩,可以通过编写脚本来读取电子表格或数据库中的数据,并将其录入到指定的系统中。常用的库包括Pandas用于处理数据,OpenPyXL用于操作Excel文件,以及SQLAlchemy用于与数据库交互。通过这些工具,可以实现批量导入、数据清洗以及格式转换等功能,提高效率。
在录入学生成绩时,如何处理数据的准确性和完整性?
确保数据的准确性和完整性是自动录入过程中的关键。可以在录入之前进行数据验证,比如检查每个成绩是否在合理范围内(如0到100),以及是否有缺失值。使用Python中的异常处理机制,可以捕获并记录任何错误,以便后续修正。此外,定期与源数据进行比对,确保系统内的数据始终保持最新和准确。
如何将录入的学生成绩可视化?
使用Python的可视化库,如Matplotlib和Seaborn,可以将录入的学生成绩以图表形式展示。通过生成柱状图、折线图或饼图,能够清晰地呈现成绩分布、平均分以及各科成绩之间的比较。这不仅帮助教师分析学生的学习情况,也能为学校的教学决策提供数据支持。可视化结果可以直接嵌入报告或仪表盘,便于分享和交流。
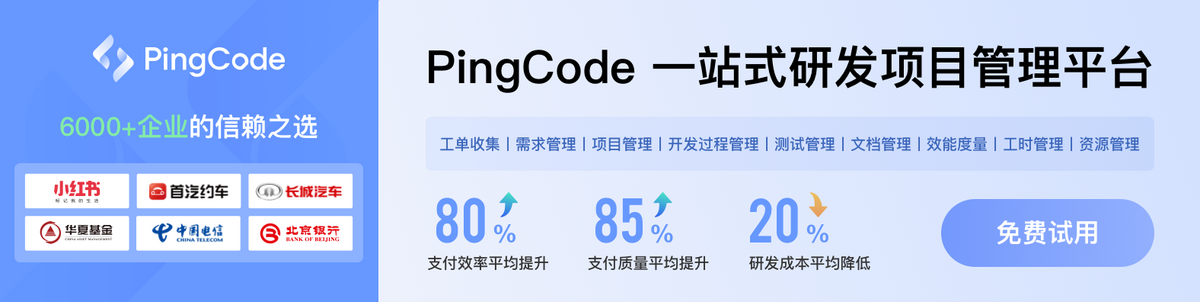