如何用Python画楼梯和围棋棋盘
在Python中,绘图可以通过多种方法实现。最常用的方法之一是使用图形库,如matplotlib、turtle等。使用matplotlib绘制棋盘、使用turtle绘制楼梯。下面将详细介绍如何使用这些库来绘制楼梯和围棋棋盘。
一、使用Turtle绘制楼梯
Turtle是Python中一个非常容易上手的图形绘制库,特别适合初学者。
1、安装和导入Turtle库
首先,确保你的Python环境中安装了turtle库。turtle库是Python标准库的一部分,一般无需额外安装。
import turtle
2、初始化Turtle
初始化turtle绘图环境,并设置画笔的速度和颜色。
t = turtle.Turtle()
t.speed(1)
t.color("black")
3、绘制楼梯
使用循环来绘制楼梯的每一级台阶。
def draw_stairs(steps, step_size):
for _ in range(steps):
t.forward(step_size)
t.left(90)
t.forward(step_size)
t.right(90)
draw_stairs(10, 20)
turtle.done()
在这个例子中,我们定义了一个函数draw_stairs
,它接受两个参数:楼梯的级数和每一级台阶的大小。通过循环,画笔前进并转向,从而形成楼梯的形状。
二、使用Matplotlib绘制围棋棋盘
Matplotlib是一个强大的Python绘图库,特别适合创建各种类型的图表和图形。
1、安装和导入Matplotlib库
如果你还没有安装matplotlib,可以使用pip进行安装:
pip install matplotlib
然后导入matplotlib库:
import matplotlib.pyplot as plt
import numpy as np
2、创建棋盘
通过绘制网格线来创建围棋棋盘。
def draw_go_board(size):
fig, ax = plt.subplots()
ax.set_xlim(0, size)
ax.set_ylim(0, size)
ax.set_aspect('equal')
# Draw grid lines
for i in range(size):
ax.plot([i, i], [0, size-1], color='black')
ax.plot([0, size-1], [i, i], color='black')
# Draw star points
star_points = [(3, 3), (3, size-4), (size-4, 3), (size-4, size-4),
(size//2, size//2), (size//2, 3), (size//2, size-4),
(3, size//2), (size-4, size//2)]
for point in star_points:
ax.plot(point[0], point[1], 'ko')
plt.show()
draw_go_board(19)
在这个例子中,我们定义了一个函数draw_go_board
,它接受一个参数:棋盘的大小(一般为19×19)。通过绘制网格线和星位点,创建一个标准的围棋棋盘。
三、总结
通过以上步骤,你可以分别使用Turtle库绘制楼梯和Matplotlib库绘制围棋棋盘。使用Turtle绘制楼梯步骤简单、使用Matplotlib绘制围棋棋盘更为专业。这两个库各有千秋,适用于不同的绘图需求。如果你是初学者,建议先从Turtle库入手,逐步掌握绘图技巧后,再尝试使用更为复杂的Matplotlib库。
四、进阶技巧
1、动态绘制楼梯
如果你希望看到动态的楼梯绘制过程,可以增加一些延时效果:
import time
def draw_stairs(steps, step_size):
for _ in range(steps):
t.forward(step_size)
t.left(90)
time.sleep(0.1) # 延时0.1秒
t.forward(step_size)
t.right(90)
time.sleep(0.1) # 延时0.1秒
draw_stairs(10, 20)
turtle.done()
2、绘制带有棋子的位置
在绘制围棋棋盘时,你还可以在棋盘上添加棋子的位置:
def draw_go_board_with_stones(size, black_stones, white_stones):
fig, ax = plt.subplots()
ax.set_xlim(0, size)
ax.set_ylim(0, size)
ax.set_aspect('equal')
# Draw grid lines
for i in range(size):
ax.plot([i, i], [0, size-1], color='black')
ax.plot([0, size-1], [i, i], color='black')
# Draw star points
star_points = [(3, 3), (3, size-4), (size-4, 3), (size-4, size-4),
(size//2, size//2), (size//2, 3), (size//2, size-4),
(3, size//2), (size-4, size//2)]
for point in star_points:
ax.plot(point[0], point[1], 'ko')
# Draw stones
for stone in black_stones:
ax.plot(stone[0], stone[1], 'ko', markersize=20)
for stone in white_stones:
ax.plot(stone[0], stone[1], 'wo', markersize=20, markeredgecolor='black')
plt.show()
black_stones = [(3, 3), (10, 10), (16, 16)]
white_stones = [(3, 16), (10, 3), (16, 3)]
draw_go_board_with_stones(19, black_stones, white_stones)
在这个例子中,draw_go_board_with_stones
函数不仅绘制了围棋棋盘,还在指定的位置添加了黑白棋子的位置。
五、进一步学习
通过以上示例,你可以初步掌握使用Python绘制楼梯和围棋棋盘的方法。深入学习图形库的各种功能、结合其他Python库扩展功能,是提升绘图技能的重要途径。
1、深入理解Turtle库
Turtle库不仅可以绘制简单的图形,还可以绘制复杂的图案和动画。你可以通过阅读Turtle库的官方文档和示例代码,深入了解其功能。
2、扩展Matplotlib的使用
Matplotlib是一个功能强大的绘图库,广泛应用于科学计算和数据可视化。你可以学习如何使用Matplotlib绘制各种类型的图表,如折线图、柱状图、散点图等,以及如何自定义图表的样式和布局。
3、结合其他Python库
除了Turtle和Matplotlib,你还可以结合其他Python库,如Pygame、Pillow等,进一步扩展你的绘图能力。例如,使用Pygame可以创建更加复杂的图形和动画,而使用Pillow可以进行图像处理和编辑。
通过不断学习和实践,你可以熟练掌握Python的绘图技巧,并应用于各种实际项目中。希望这篇文章对你有所帮助,祝你在Python绘图的学习之路上取得更大的进步。
相关问答FAQs:
如何用Python绘制楼梯图形?
可以使用Python的matplotlib
库来绘制楼梯图形。首先需要安装matplotlib
,然后使用plot
函数定义楼梯的坐标。通过设置坐标点的x和y值,可以实现楼梯的效果。例如,使用numpy
生成x轴的坐标,再通过循环设置y轴的高度,最后调用plt.plot()
绘制出楼梯形状。
使用Python绘制围棋棋盘的步骤是什么?
绘制围棋棋盘时,通常需要使用matplotlib
库。可以创建一个19×19的网格,设置合适的坐标轴范围。绘制时,可以使用plt.grid()
函数来生成网格线,并通过plt.scatter()
在特定位置添加棋子。棋盘的背景色也可以通过plt.gca().set_facecolor()
来调整,以增强视觉效果。
如何在Python中自定义棋盘的大小和样式?
在Python中绘制围棋棋盘时,可以通过调整网格的大小和样式来实现自定义。使用plt.xlim()
和plt.ylim()
可以设置棋盘的范围,plt.xticks()
和plt.yticks()
则可以修改坐标轴的刻度。为了更改棋盘的样式,例如线条颜色和粗细,可以在调用plt.grid()
时设置参数,如color
和linewidth
,以便使棋盘更具个性化。
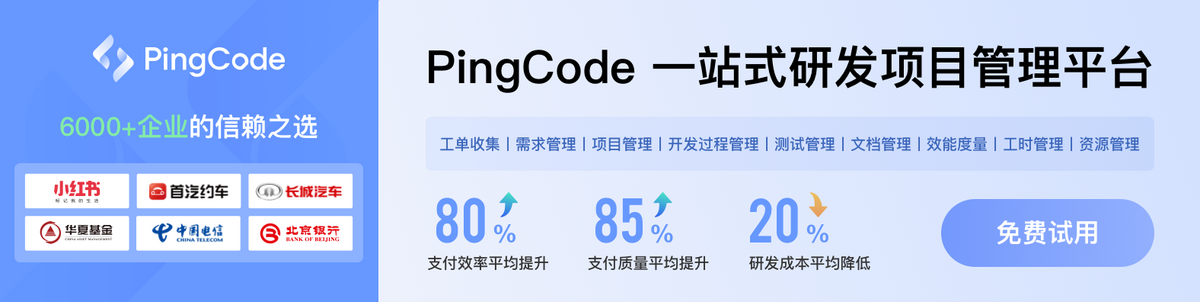