Python远程连接MySQL数据库的方法包括安装必要的库、配置数据库连接、使用连接池优化性能。其中,最常用的库是mysql-connector-python
,它提供了简便的方法来连接和操作MySQL数据库。接下来,我们将详细探讨如何在Python中实现远程连接到MySQL数据库,并讨论一些最佳实践和性能优化技巧。
一、安装必要的库
在开始之前,你需要确保你的Python环境中安装了mysql-connector-python
库。你可以通过以下命令安装该库:
pip install mysql-connector-python
如果你更倾向于使用其他库,比如pymysql
或MySQLdb
,也可以根据需要进行安装和配置。
二、配置数据库连接
- 使用
mysql-connector-python
库连接MySQL数据库
import mysql.connector
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'remote_host_ip',
'database': 'database_name',
'raise_on_warnings': True
}
try:
cnx = mysql.connector.connect(config)
cursor = cnx.cursor()
cursor.execute("SELECT DATABASE();")
row = cursor.fetchone()
print("Connected to database:", row)
except mysql.connector.Error as err:
print("Error: {}".format(err))
finally:
if cnx.is_connected():
cursor.close()
cnx.close()
print("Connection closed.")
三、使用连接池优化性能
- 连接池的概念与优势
连接池是一种资源管理模式,旨在减少建立和关闭数据库连接的开销。它允许多个客户端共享固定数量的数据库连接,从而提高应用程序的性能和响应速度。
- 使用
mysql-connector-python
实现连接池
from mysql.connector import pooling
dbconfig = {
'user': 'your_username',
'password': 'your_password',
'host': 'remote_host_ip',
'database': 'database_name'
}
try:
pool = mysql.connector.pooling.MySQLConnectionPool(pool_name="mypool",
pool_size=5,
dbconfig)
cnx = pool.get_connection()
cursor = cnx.cursor()
cursor.execute("SELECT DATABASE();")
row = cursor.fetchone()
print("Connected to database:", row)
except mysql.connector.Error as err:
print("Error: {}".format(err))
finally:
if cnx.is_connected():
cursor.close()
cnx.close()
print("Connection closed.")
四、处理常见错误和异常
- 用户认证失败
当连接到远程MySQL数据库时,可能会遇到用户认证失败的问题。确保你提供的用户名和密码是正确的,并且你的用户在MySQL服务器上具有适当的权限。
except mysql.connector.Error as err:
if err.errno == mysql.connector.errorcode.ER_ACCESS_DENIED_ERROR:
print("Something is wrong with your user name or password")
else:
print(err)
- 网络连接错误
网络连接错误可能由于防火墙、网络配置或服务器未启动等原因引起。你可以通过检查网络连接和服务器状态来解决这个问题。
except mysql.connector.Error as err:
if err.errno == mysql.connector.errorcode.ER_BAD_HOST_ERROR:
print("Network connection error")
else:
print(err)
五、执行数据库操作
连接到数据库后,你可以执行各种数据库操作,如查询、插入、更新和删除数据。
- 查询数据
try:
cnx = mysql.connector.connect(config)
cursor = cnx.cursor()
query = "SELECT * FROM your_table"
cursor.execute(query)
for row in cursor.fetchall():
print(row)
except mysql.connector.Error as err:
print("Error: {}".format(err))
finally:
if cnx.is_connected():
cursor.close()
cnx.close()
print("Connection closed.")
- 插入数据
try:
cnx = mysql.connector.connect(config)
cursor = cnx.cursor()
add_data = ("INSERT INTO your_table "
"(column1, column2) "
"VALUES (%s, %s)")
data = ('value1', 'value2')
cursor.execute(add_data, data)
cnx.commit()
print("Data inserted")
except mysql.connector.Error as err:
print("Error: {}".format(err))
finally:
if cnx.is_connected():
cursor.close()
cnx.close()
print("Connection closed.")
六、确保安全性
在远程连接MySQL数据库时,安全性是一个重要的考虑因素。确保你的连接是通过加密通道进行的,以防止数据在传输过程中被窃取。你可以使用SSL/TLS来加密MySQL连接。
- 配置SSL/TLS连接
config = {
'user': 'your_username',
'password': 'your_password',
'host': 'remote_host_ip',
'database': 'database_name',
'ssl_ca': '/path/to/ca-cert.pem',
'ssl_cert': '/path/to/client-cert.pem',
'ssl_key': '/path/to/client-key.pem'
}
try:
cnx = mysql.connector.connect(config)
cursor = cnx.cursor()
cursor.execute("SELECT DATABASE();")
row = cursor.fetchone()
print("Connected to database:", row)
except mysql.connector.Error as err:
print("Error: {}".format(err))
finally:
if cnx.is_connected():
cursor.close()
cnx.close()
print("Connection closed.")
七、优化查询性能
- 使用索引
为了提高查询性能,确保在你的表中使用索引。索引可以显著减少查询时间,特别是对于大型数据集。
CREATE INDEX idx_column1 ON your_table (column1);
- 避免使用SELECT *
在查询中指定具体的列,而不是使用SELECT *
,可以减少传输的数据量,从而提高查询性能。
query = "SELECT column1, column2 FROM your_table WHERE condition"
八、日志和监控
- 启用数据库日志
启用数据库日志可以帮助你监控和调试数据库操作。你可以配置MySQL服务器以记录所有查询和连接信息。
SET GLOBAL general_log = 'ON';
SET GLOBAL general_log_file = '/path/to/logfile.log';
- 使用监控工具
使用监控工具,如Prometheus、Grafana或MySQL Enterprise Monitor,可以帮助你实时监控数据库性能和健康状况。
通过以上步骤,你可以在Python中实现远程连接到MySQL数据库,并进行各种数据库操作。记住要优化你的查询、使用连接池和确保连接的安全性,以提高应用程序的性能和可靠性。
相关问答FAQs:
如何在Python中设置远程MySQL数据库连接?
要在Python中连接远程MySQL数据库,您需要安装MySQL的Python驱动程序,如mysql-connector-python
或PyMySQL
。安装完成后,可以使用以下代码示例进行连接:
import mysql.connector
connection = mysql.connector.connect(
host='远程主机地址',
user='数据库用户名',
password='数据库密码',
database='数据库名称'
)
cursor = connection.cursor()
cursor.execute("SELECT DATABASE();")
print(cursor.fetchone())
cursor.close()
connection.close()
确保在代码中替换为实际的连接信息。
在连接MySQL数据库时,如何处理异常情况?
在进行数据库连接时,处理异常是非常重要的,可以使用try-except
块来捕获可能出现的错误。例如:
try:
connection = mysql.connector.connect(...)
except mysql.connector.Error as err:
print(f"连接错误: {err}")
通过这种方式,您可以获得详细的错误信息,从而方便调试和修复问题。
如何确保Python程序在远程连接MySQL数据库时的安全性?
为了确保连接的安全性,建议使用SSL加密连接、限制数据库用户的权限、定期更改密码以及使用防火墙限制访问IP。此外,最好不要在代码中硬编码敏感信息,而是使用环境变量或配置文件存储这些数据。
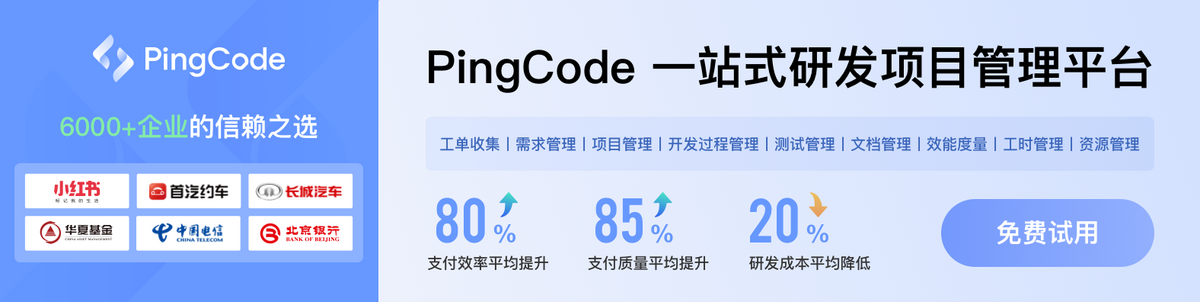