使用Python做无人机编队,需要掌握无人机编程接口、通信协议、多线程编程、以及路径规划算法等。其中,掌握无人机编程接口是最重要的,因为这是实现无人机与计算机通信、控制无人机飞行的基础。无人机编队通常需要使用ROS(机器人操作系统)进行通信和控制,通过Python编写控制代码实现多机协同飞行。下面详细介绍如何用Python实现无人机编队。
一、无人机编程接口
无人机编程接口是实现无人机编队的基础,常见的无人机编程接口包括DJI SDK、MAVSDK等。以DJI SDK为例,用户可以通过其提供的API实现对无人机的各种控制。使用Python编写代码时,可以利用DJI提供的Python库来实现对无人机的控制。
1. 安装DJI SDK
首先,需要安装DJI SDK的Python库,可以通过pip进行安装:
pip install dji-sdk
2. 初始化无人机
初始化无人机是实现编队控制的第一步。通过DJI SDK,可以实现无人机的初始化和基本控制。以下是一个简单的初始化代码示例:
from dji_sdk import dji
初始化无人机对象
drone = dji.Drone()
连接无人机
drone.connect()
3. 控制无人机
通过DJI SDK提供的API,可以实现对无人机的各种控制,比如起飞、降落、悬停等。以下是一个简单的控制无人机起飞和降落的代码示例:
# 起飞
drone.takeoff()
悬停5秒
time.sleep(5)
降落
drone.land()
二、通信协议
在无人机编队中,通信协议是实现多机协同飞行的关键。常用的通信协议包括ROS(机器人操作系统)和MAVLink。通过这些协议,可以实现多个无人机之间的数据通信和协同控制。
1. ROS通信
ROS是一个开源的机器人操作系统,通过其提供的通信机制,可以实现多个无人机之间的协同控制。以下是一个简单的ROS通信示例:
import rospy
from std_msgs.msg import String
初始化ROS节点
rospy.init_node('drone_commander', anonymous=True)
创建发布者
pub = rospy.Publisher('drone_commands', String, queue_size=10)
发布消息
command = "takeoff"
pub.publish(command)
2. MAVLink通信
MAVLink是一种轻量级的通信协议,广泛应用于无人机的控制。通过MAVLink,可以实现多个无人机之间的通信和协同控制。以下是一个简单的MAVLink通信示例:
import pymavlink
创建MAVLink连接
connection = pymavlink.mavutil.mavlink_connection('udp:127.0.0.1:14550')
发送起飞命令
connection.mav.command_long_send(
connection.target_system,
connection.target_component,
pymavlink.mavutil.mavlink.MAV_CMD_NAV_TAKEOFF,
0,
0, 0, 0, 0, 0, 0, 10
)
三、多线程编程
在无人机编队中,为了实现多个无人机的并行控制,可以使用Python的多线程编程。通过多线程,可以实现多个无人机的并行控制和数据通信。
1. 创建多线程
通过Python的threading
模块,可以轻松创建多线程。以下是一个简单的多线程示例:
import threading
定义线程函数
def control_drone(drone_id):
print(f"Controlling drone {drone_id}")
创建线程
thread1 = threading.Thread(target=control_drone, args=(1,))
thread2 = threading.Thread(target=control_drone, args=(2,))
启动线程
thread1.start()
thread2.start()
等待线程结束
thread1.join()
thread2.join()
2. 并行控制无人机
通过多线程,可以实现多个无人机的并行控制。以下是一个并行控制无人机的代码示例:
import threading
import time
定义控制函数
def control_drone(drone):
drone.takeoff()
time.sleep(5)
drone.land()
创建无人机对象
drone1 = dji.Drone()
drone2 = dji.Drone()
连接无人机
drone1.connect()
drone2.connect()
创建线程
thread1 = threading.Thread(target=control_drone, args=(drone1,))
thread2 = threading.Thread(target=control_drone, args=(drone2,))
启动线程
thread1.start()
thread2.start()
等待线程结束
thread1.join()
thread2.join()
四、路径规划算法
在无人机编队中,路径规划算法是实现多机协同飞行的核心。常见的路径规划算法包括A*算法、Dijkstra算法、RRT算法等。通过这些算法,可以实现无人机的路径规划和避障。
1. A*算法
A算法是一种常用的路径规划算法,通过启发式函数,可以高效地找到最优路径。以下是一个简单的A算法实现示例:
import heapq
def a_star(start, goal, grid):
open_list = []
heapq.heappush(open_list, (0, start))
came_from = {}
g_score = {start: 0}
f_score = {start: heuristic(start, goal)}
while open_list:
current = heapq.heappop(open_list)[1]
if current == goal:
return reconstruct_path(came_from, current)
for neighbor in get_neighbors(current, grid):
tentative_g_score = g_score[current] + 1
if neighbor not in g_score or tentative_g_score < g_score[neighbor]:
came_from[neighbor] = current
g_score[neighbor] = tentative_g_score
f_score[neighbor] = g_score[neighbor] + heuristic(neighbor, goal)
heapq.heappush(open_list, (f_score[neighbor], neighbor))
return None
def heuristic(a, b):
return abs(a[0] - b[0]) + abs(a[1] - b[1])
def get_neighbors(node, grid):
neighbors = []
for dx, dy in [(-1, 0), (1, 0), (0, -1), (0, 1)]:
x, y = node[0] + dx, node[1] + dy
if 0 <= x < len(grid) and 0 <= y < len(grid[0]) and grid[x][y] == 0:
neighbors.append((x, y))
return neighbors
def reconstruct_path(came_from, current):
path = [current]
while current in came_from:
current = came_from[current]
path.append(current)
return path[::-1]
2. RRT算法
RRT(快速随机树)算法是一种常用的采样基路径规划算法,通过随机采样,可以高效地生成路径。以下是一个简单的RRT算法实现示例:
import random
class Node:
def __init__(self, x, y):
self.x = x
self.y = y
self.parent = None
def rrt(start, goal, grid, max_iter=1000):
start_node = Node(start[0], start[1])
goal_node = Node(goal[0], goal[1])
tree = [start_node]
for _ in range(max_iter):
rand_node = Node(random.randint(0, len(grid) - 1), random.randint(0, len(grid[0]) - 1))
nearest_node = min(tree, key=lambda node: distance(node, rand_node))
new_node = steer(nearest_node, rand_node, grid)
if new_node:
tree.append(new_node)
if distance(new_node, goal_node) < 1:
return reconstruct_path(new_node)
return None
def distance(node1, node2):
return ((node1.x - node2.x) <strong> 2 + (node1.y - node2.y) </strong> 2) 0.5
def steer(from_node, to_node, grid):
new_node = Node(to_node.x, to_node.y)
new_node.parent = from_node
if is_valid(new_node, grid):
return new_node
return None
def is_valid(node, grid):
if 0 <= node.x < len(grid) and 0 <= node.y < len(grid[0]) and grid[node.x][node.y] == 0:
return True
return False
def reconstruct_path(node):
path = [(node.x, node.y)]
while node.parent:
node = node.parent
path.append((node.x, node.y))
return path[::-1]
五、无人机编队控制
通过结合上述内容,可以实现无人机编队控制。以下是一个简单的无人机编队控制示例:
1. 定义无人机类
首先,定义一个无人机类,实现无人机的基本控制和通信:
import threading
import time
class Drone:
def __init__(self, id):
self.id = id
self.position = (0, 0)
self.connected = False
def connect(self):
print(f"Drone {self.id} connected")
self.connected = True
def takeoff(self):
if self.connected:
print(f"Drone {self.id} taking off")
else:
print(f"Drone {self.id} not connected")
def land(self):
if self.connected:
print(f"Drone {self.id} landing")
else:
print(f"Drone {self.id} not connected")
def move_to(self, position):
if self.connected:
print(f"Drone {self.id} moving to {position}")
self.position = position
else:
print(f"Drone {self.id} not connected")
2. 实现编队控制
通过多线程实现多个无人机的编队控制:
def control_drone(drone, path):
drone.connect()
drone.takeoff()
for position in path:
drone.move_to(position)
time.sleep(1)
drone.land()
创建无人机对象
drone1 = Drone(1)
drone2 = Drone(2)
定义路径
path1 = [(0, 0), (1, 1), (2, 2), (3, 3)]
path2 = [(0, 0), (1, 0), (2, 0), (3, 0)]
创建线程
thread1 = threading.Thread(target=control_drone, args=(drone1, path1))
thread2 = threading.Thread(target=control_drone, args=(drone2, path2))
启动线程
thread1.start()
thread2.start()
等待线程结束
thread1.join()
thread2.join()
通过以上步骤,可以实现无人机的基本编队控制。根据实际需求,还可以进一步优化代码,实现更复杂的编队控制和路径规划。
相关问答FAQs:
如何使用Python进行无人机编队的基本步骤是什么?
要使用Python进行无人机编队,首先需要选择合适的无人机平台和开发工具。常见的工具包括DroneKit和ROS(机器人操作系统)。可以通过这些工具控制无人机的飞行路径、速度和相对位置。接着,设计编队算法,确保无人机能够在飞行中保持相对位置,例如使用加速度、速度控制和通信协议来实现编队效果。确保测试和调试代码,以便在实际飞行中保持安全和有效的编队。
Python在无人机编队中的优势有哪些?
Python因其简洁的语法和强大的库而受到广泛欢迎。在无人机编队中,Python可以通过其丰富的库(如NumPy和SciPy)进行高效的数据处理和数学计算。此外,Python的可读性和易于调试的特性使得开发者能够快速迭代和优化编队算法。利用Python的网络库,还可以实现无人机之间的实时通信,从而提高编队的协调性和灵活性。
如何解决无人机编队中的通信问题?
在无人机编队中,通信是确保各无人机协调飞行的关键。可以使用基于Python的通信库,如Socket或MQTT,来建立无人机之间的通信通道。此外,确保每架无人机都能接收和发送状态信息,实时更新位置和速度。为了增强通信的可靠性,可以考虑使用多种通信方式(如Wi-Fi、蓝牙或LoRa)来降低信号丢失的风险,并设计冗余方案以处理潜在的通信中断问题。
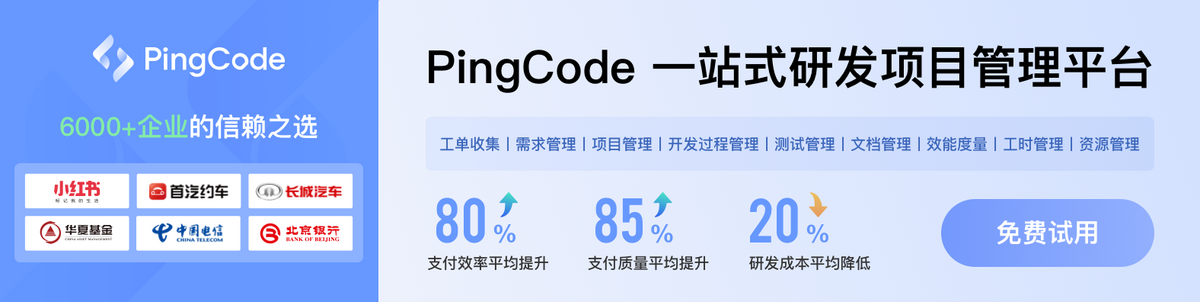