在Python中,有几种常用的方法来输出字符串,包括使用print函数、格式化字符串以及使用日志模块等。下面将详细介绍其中一种方法,即使用print函数。
print函数:这是Python中最常用的输出字符串的方法。它可以将指定的字符串或变量的值输出到控制台。
print("Hello, World!")
这种方法非常简单直观,对于大多数基本输出需求都能满足。下面我们将详细讲解其他几种输出字符串的方法,并介绍它们的用法和适用场景。
一、使用print函数
基本用法
在Python中,print()
函数是最基本的输出字符串的方法。你只需要将字符串作为参数传递给print()
函数,它就会将字符串输出到控制台。
print("Hello, World!")
print('Python is fun!')
输出多个字符串
你可以在print()
函数中传递多个参数,它们之间会用空格分隔。
print("Hello", "World", "from", "Python")
使用变量
你可以将字符串赋值给变量,然后使用print()
函数输出变量的值。
greeting = "Hello, World!"
print(greeting)
转义字符
在字符串中使用转义字符(例如\n
表示换行,\t
表示制表符)来控制输出格式。
print("Hello, \nWorld!")
print("Name:\tJohn")
二、使用格式化字符串
% 格式化
这种方法类似于C语言的字符串格式化,使用 %
操作符。
name = "John"
age = 30
print("My name is %s and I am %d years old." % (name, age))
str.format() 方法
str.format()
方法提供了一种更强大和灵活的字符串格式化方式。
name = "John"
age = 30
print("My name is {} and I am {} years old.".format(name, age))
f-strings (Python 3.6+)
f-strings 是Python 3.6中引入的一种更简洁的字符串格式化方式。
name = "John"
age = 30
print(f"My name is {name} and I am {age} years old.")
三、使用日志模块
对于更复杂的应用,特别是需要记录程序运行日志时,Python提供了logging
模块。
import logging
logging.basicConfig(level=logging.DEBUG)
logging.debug("This is a debug message")
logging.info("This is an info message")
logging.warning("This is a warning message")
logging.error("This is an error message")
logging.critical("This is a critical message")
自定义日志格式
你可以自定义日志的输出格式,以适应不同的需求。
import logging
logging.basicConfig(
level=logging.DEBUG,
format='%(asctime)s - %(levelname)s - %(message)s'
)
logging.debug("This is a debug message")
四、写入文件
除了将字符串输出到控制台,你还可以将字符串写入文件。
基本写入文件
使用 open()
函数打开文件,并使用 write()
方法写入字符串。
with open("output.txt", "w") as file:
file.write("Hello, World!")
追加写入文件
使用 open()
函数并指定模式为 a
,可以将字符串追加到文件末尾。
with open("output.txt", "a") as file:
file.write("\nHello again!")
五、使用sys模块
有时你可能需要更细粒度地控制输出,例如将输出重定向到标准错误。
输出到标准错误
使用 sys.stderr
可以将字符串输出到标准错误。
import sys
sys.stderr.write("This is an error message\n")
输出到标准输出
虽然 print()
函数默认输出到标准输出,但你也可以使用 sys.stdout
进行更细粒度的控制。
import sys
sys.stdout.write("This is a standard output message\n")
六、使用字符串拼接
在某些情况下,你可能需要动态地构建输出字符串,这可以通过字符串拼接实现。
使用 + 操作符
part1 = "Hello"
part2 = "World"
message = part1 + ", " + part2 + "!"
print(message)
使用 join() 方法
对于多段字符串的拼接,join()
方法更高效。
parts = ["Hello", "World"]
message = ", ".join(parts)
print(message)
七、使用模板字符串
Python的 string
模块提供了 Template
类,用于更高级的字符串替换。
基本用法
from string import Template
template = Template("My name is $name and I am $age years old.")
message = template.substitute(name="John", age=30)
print(message)
使用字典进行替换
from string import Template
template = Template("My name is $name and I am $age years old.")
data = {"name": "John", "age": 30}
message = template.substitute(data)
print(message)
八、使用第三方库
在某些情况下,内置的字符串处理功能可能不够强大,你可以使用第三方库如 tabulate
、prettytable
来处理更复杂的输出。
使用 tabulate
from tabulate import tabulate
data = [["Name", "Age"], ["John", 30], ["Jane", 25]]
print(tabulate(data, headers="firstrow", tablefmt="grid"))
使用 prettytable
from prettytable import PrettyTable
table = PrettyTable()
table.field_names = ["Name", "Age"]
table.add_row(["John", 30])
table.add_row(["Jane", 25])
print(table)
总结
在Python中,输出字符串的方法有很多种,每种方法都有其适用的场景和优缺点。通过使用print函数、格式化字符串、日志模块、文件写入、sys模块、字符串拼接、模板字符串以及第三方库,你可以根据具体需求选择合适的方法来输出字符串。无论是简单的控制台输出,还是复杂的日志记录和格式化输出,Python都提供了丰富的工具来满足你的需求。
相关问答FAQs:
如何在Python中打印字符串到控制台?
在Python中,可以使用内置的print()
函数来输出字符串。只需将要输出的字符串作为参数传递给print()
函数。例如,print("Hello, World!")
将会在控制台上显示“Hello, World!”。这个方法非常简单且常用,是Python编程的基础之一。
是否可以在输出字符串时使用格式化?
是的,Python支持多种字符串格式化方式。可以使用f-字符串(在字符串前加f),str.format()
方法,或者百分号(%)格式化。例如,使用f-字符串可以这样写:name = "Alice"; print(f"Hello, {name}!")
,这将输出“Hello, Alice!”。这种格式化方式使得在输出中插入变量变得更为直观和方便。
如何在输出字符串时添加换行符或其他特殊字符?
在Python中,可以使用转义字符来添加换行符或其他特殊字符。例如,使用\n
可以在字符串中插入换行,使用\t
可以插入制表符。示例代码如下:print("Hello,\nWorld!")
将会输出两行内容,“Hello,”和“World!”分别在不同的行中显示。这使得输出内容更加灵活和可读。
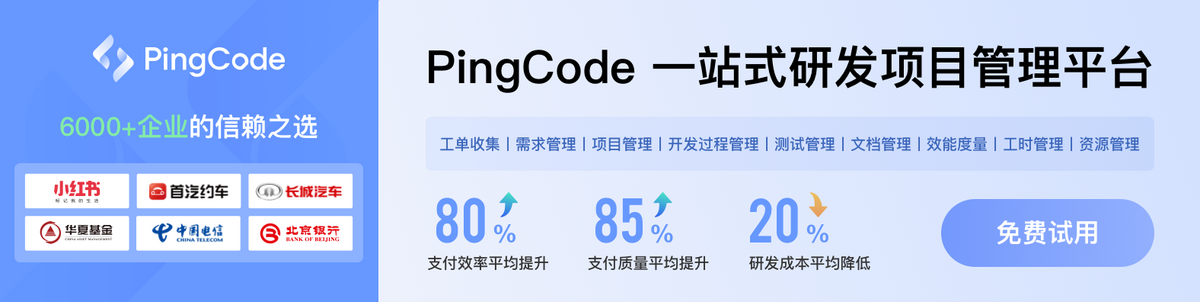