在Python中,可以通过使用 zip()
函数、itertools.zip_longest()
函数、列表索引和列表解析等方法来同时遍历四个列表。 其中,使用 zip()
函数是最常见的方法,它能够将多个可迭代对象打包成一个可迭代的元组,适用于长度相同的列表。而 itertools.zip_longest()
函数则可以处理长度不同的列表,它会将较短列表用填充值补齐。接下来,我们将详细介绍这些方法。
一、使用 zip()
函数
zip()
函数是Python内置的一个函数,它能够接受多个可迭代对象作为参数,并将对应元素打包成一个个元组。使用 zip()
函数可以很方便地同时遍历多个列表。
例如:
list1 = [1, 2, 3, 4]
list2 = ['a', 'b', 'c', 'd']
list3 = [0.1, 0.2, 0.3, 0.4]
list4 = ['one', 'two', 'three', 'four']
for a, b, c, d in zip(list1, list2, list3, list4):
print(a, b, c, d)
在上面的代码中,zip(list1, list2, list3, list4)
会将四个列表的对应元素打包成一个个元组,并通过 for
循环一次性解包到变量 a, b, c, d
中,这样我们就能同时遍历四个列表。
二、使用 itertools.zip_longest()
函数
如果四个列表的长度不一致,可以使用 itertools.zip_longest()
函数。该函数会将较短的列表用指定的填充值(默认为 None
)补齐,使得我们能够遍历所有列表中的每一个元素。
例如:
from itertools import zip_longest
list1 = [1, 2, 3, 4]
list2 = ['a', 'b']
list3 = [0.1, 0.2, 0.3]
list4 = ['one', 'two', 'three', 'four', 'five']
for a, b, c, d in zip_longest(list1, list2, list3, list4, fillvalue=None):
print(a, b, c, d)
在上面的代码中,zip_longest(list1, list2, list3, list4, fillvalue=None)
会将较短的列表用 None
补齐,并返回一个可迭代的元组。
三、使用列表索引
如果希望手动控制遍历索引,可以使用列表索引来遍历四个列表。这种方法适用于列表长度一致的情况。
例如:
list1 = [1, 2, 3, 4]
list2 = ['a', 'b', 'c', 'd']
list3 = [0.1, 0.2, 0.3, 0.4]
list4 = ['one', 'two', 'three', 'four']
for i in range(len(list1)):
print(list1[i], list2[i], list3[i], list4[i])
在上面的代码中,通过 range(len(list1))
生成索引序列,然后使用索引同时访问四个列表中的元素。
四、使用列表解析
列表解析是一种简洁而高效的列表生成方式,也可以用于同时遍历多个列表。
例如:
list1 = [1, 2, 3, 4]
list2 = ['a', 'b', 'c', 'd']
list3 = [0.1, 0.2, 0.3, 0.4]
list4 = ['one', 'two', 'three', 'four']
result = [(a, b, c, d) for a, b, c, d in zip(list1, list2, list3, list4)]
for item in result:
print(item)
在上面的代码中,列表解析生成了一个包含元组的列表,然后通过 for
循环逐个打印这些元组。
五、性能和注意事项
在选择遍历方法时,需要考虑列表长度、可读性和性能等因素。如果列表长度相同且较短,使用 zip()
函数是最简洁和高效的选择。如果列表长度不一致或较长,itertools.zip_longest()
函数会更适用。此外,使用列表索引和列表解析也各有优缺点,前者可读性较好,后者在生成新列表时更加简洁。
需要注意的是,在遍历过程中修改列表的内容可能会导致不可预知的行为, 因此在遍历列表时尽量避免修改列表的内容。
通过以上几种方法,我们可以灵活地同时遍历四个列表,以满足不同场景的需求。希望这些方法能够帮助你更好地处理多列表遍历的问题。
相关问答FAQs:
如何在Python中实现同时遍历四个列表?
在Python中,可以使用zip
函数轻松地同时遍历多个列表。通过将四个列表作为参数传递给zip
,您将获得一个包含元组的迭代器,每个元组对应于四个列表中的元素。例如:
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
list3 = [True, False, True]
list4 = [0.1, 0.2, 0.3]
for item1, item2, item3, item4 in zip(list1, list2, list3, list4):
print(item1, item2, item3, item4)
上述代码将同时打印四个列表中的对应元素。
使用zip函数时如何处理不同长度的列表?
如果您使用zip
函数遍历的列表长度不一致,zip
将根据最短的列表进行遍历。这意味着多余的元素将会被忽略。如果您希望遍历所有元素,可以使用itertools.zip_longest
,它会用None
填充缺失的值:
from itertools import zip_longest
list1 = [1, 2, 3]
list2 = ['a', 'b']
list3 = [True]
list4 = [0.1, 0.2, 0.3]
for item1, item2, item3, item4 in zip_longest(list1, list2, list3, list4):
print(item1, item2, item3, item4)
在遍历四个列表时如何获取索引?
如果您需要在遍历四个列表的同时获取索引,可以使用enumerate
结合zip
。通过这种方式,您可以轻松获取当前元素的索引:
list1 = [10, 20, 30]
list2 = ['x', 'y', 'z']
list3 = [1.1, 2.2, 3.3]
list4 = [True, False, True]
for index, (item1, item2, item3, item4) in enumerate(zip(list1, list2, list3, list4)):
print(index, item1, item2, item3, item4)
这种方式可以帮助您在处理数据时保持对元素位置的清晰认识。
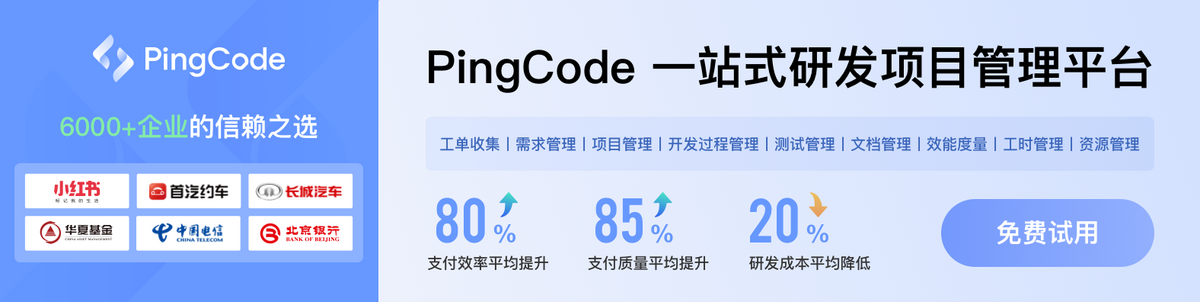