要合并两个txt文档,可以使用Python中的文件操作方法。
Python提供了多种方式来处理文件操作,如open函数、with语句、read和write方法等。以下是一个详细的例子,展示了如何用Python合并两个txt文档:
def merge_txt_files(file1, file2, output_file):
# 打开第一个文件并读取内容
with open(file1, 'r', encoding='utf-8') as f1:
content1 = f1.read()
# 打开第二个文件并读取内容
with open(file2, 'r', encoding='utf-8') as f2:
content2 = f2.read()
# 将两个文件的内容合并
merged_content = content1 + "\n" + content2
# 将合并后的内容写入到输出文件中
with open(output_file, 'w', encoding='utf-8') as f_out:
f_out.write(merged_content)
使用示例
file1 = 'file1.txt'
file2 = 'file2.txt'
output_file = 'merged.txt'
merge_txt_files(file1, file2, output_file)
在以上代码中,我们使用了with语句来确保文件在使用完毕后自动关闭,避免资源泄露。 通过读取两个文件的内容并将它们合并到一个新的文件中,我们可以轻松地实现两个txt文档的合并。
一、使用open函数
open函数是Python中打开文件的基本方法。 它允许我们以不同的模式打开文件,如读取模式('r')、写入模式('w')、追加模式('a')等。
# 打开文件
file1 = open('file1.txt', 'r', encoding='utf-8')
file2 = open('file2.txt', 'r', encoding='utf-8')
读取文件内容
content1 = file1.read()
content2 = file2.read()
合并内容
merged_content = content1 + "\n" + content2
写入到新文件
output_file = open('merged.txt', 'w', encoding='utf-8')
output_file.write(merged_content)
关闭文件
file1.close()
file2.close()
output_file.close()
使用open函数时,需要手动关闭文件。 这可能会导致文件资源未被释放的问题,因此建议使用with语句来自动管理文件资源。
二、使用with语句
with语句可以确保文件在使用完毕后自动关闭。 这有助于避免资源泄露问题,并使代码更加简洁。
with open('file1.txt', 'r', encoding='utf-8') as file1, open('file2.txt', 'r', encoding='utf-8') as file2:
content1 = file1.read()
content2 = file2.read()
with open('merged.txt', 'w', encoding='utf-8') as output_file:
output_file.write(content1 + "\n" + content2)
使用with语句可以让代码更加简洁和安全。 它确保文件在使用完毕后自动关闭,无需手动调用close方法。
三、处理大文件
在处理大文件时,直接读取整个文件内容可能会导致内存不足的问题。 我们可以通过逐行读取和写入的方式来处理大文件。
def merge_large_files(file1, file2, output_file):
with open(file1, 'r', encoding='utf-8') as f1, open(file2, 'r', encoding='utf-8') as f2, open(output_file, 'w', encoding='utf-8') as f_out:
for line in f1:
f_out.write(line)
f_out.write("\n")
for line in f2:
f_out.write(line)
使用示例
file1 = 'large_file1.txt'
file2 = 'large_file2.txt'
output_file = 'merged_large.txt'
merge_large_files(file1, file2, output_file)
通过逐行读取和写入,可以有效地处理大文件,避免内存不足的问题。
四、使用pandas库
pandas库是一个强大的数据处理库,适用于处理结构化数据。 虽然txt文件不一定是结构化数据,但我们可以使用pandas来处理具有特定格式的txt文件。
import pandas as pd
读取txt文件
df1 = pd.read_csv('file1.txt', header=None)
df2 = pd.read_csv('file2.txt', header=None)
合并数据框
merged_df = pd.concat([df1, df2], ignore_index=True)
写入到新文件
merged_df.to_csv('merged.txt', index=False, header=False)
pandas库提供了强大的数据处理功能,适用于处理具有特定格式的txt文件。 通过读取txt文件并将其合并为一个数据框,我们可以轻松地实现文件的合并。
五、使用Pathlib库
Pathlib库是Python 3.4引入的用于处理文件和目录路径的库。 它提供了一种面向对象的方式来操作文件和目录。
from pathlib import Path
file1 = Path('file1.txt')
file2 = Path('file2.txt')
output_file = Path('merged.txt')
读取文件内容
content1 = file1.read_text(encoding='utf-8')
content2 = file2.read_text(encoding='utf-8')
合并内容
merged_content = content1 + "\n" + content2
写入到新文件
output_file.write_text(merged_content, encoding='utf-8')
Pathlib库提供了简洁的API来处理文件和目录路径。 它可以简化文件操作,使代码更加易读。
六、处理不同编码的文件
有时我们需要处理不同编码的文件。 为了确保文件内容正确读取和写入,我们需要指定正确的编码。
def merge_files_with_encoding(file1, file2, output_file, encoding1='utf-8', encoding2='utf-8', output_encoding='utf-8'):
with open(file1, 'r', encoding=encoding1) as f1, open(file2, 'r', encoding=encoding2) as f2, open(output_file, 'w', encoding=output_encoding) as f_out:
for line in f1:
f_out.write(line)
f_out.write("\n")
for line in f2:
f_out.write(line)
使用示例
file1 = 'file1.txt'
file2 = 'file2.txt'
output_file = 'merged.txt'
merge_files_with_encoding(file1, file2, output_file, encoding1='utf-8', encoding2='utf-16', output_encoding='utf-8')
通过指定不同的编码,我们可以确保文件内容正确读取和写入。 这对于处理多种语言和字符集的文件非常重要。
七、处理文件名列表
有时我们需要合并多个文件。 我们可以通过处理文件名列表来实现这一点。
def merge_multiple_files(file_list, output_file):
with open(output_file, 'w', encoding='utf-8') as f_out:
for file in file_list:
with open(file, 'r', encoding='utf-8') as f:
for line in f:
f_out.write(line)
f_out.write("\n")
使用示例
file_list = ['file1.txt', 'file2.txt', 'file3.txt']
output_file = 'merged_multiple.txt'
merge_multiple_files(file_list, output_file)
通过处理文件名列表,我们可以轻松地合并多个文件。 这在批量处理文件时非常有用。
八、总结
合并两个txt文档在Python中可以通过多种方式实现。 我们可以使用open函数、with语句、逐行读取和写入、pandas库、Pathlib库、处理不同编码的文件以及处理文件名列表等方法来实现文件的合并。
选择合适的方法取决于具体的需求和场景。 对于小文件,可以直接读取和写入;对于大文件,建议逐行处理;对于具有特定格式的文件,可以使用pandas库;对于不同编码的文件,需要指定正确的编码;对于多个文件,可以处理文件名列表。
通过以上方法,我们可以灵活地处理和合并txt文档,满足不同的需求。
相关问答FAQs:
如何在Python中读取多个txt文件的内容?
在Python中,可以使用内置的open()
函数来读取多个txt文件的内容。通过循环遍历文件名列表,依次打开每个文件,并使用read()
或readlines()
方法读取内容。可以将读取的内容存储在一个列表中,以便后续处理。
合并txt文件时如何处理重复内容?
在合并txt文件时,如果不希望出现重复的内容,可以在读取文件时将内容存入一个集合(set)。集合会自动去重,从而确保合并后的文件中每行内容都是唯一的。最后,将集合中的内容写入新文件时,可以使用writelines()
方法。
合并多个txt文件时,如何添加分隔符?
在合并多个txt文件的过程中,可以在每个文件的内容之间添加分隔符。可以在读取每个文件内容后,手动插入分隔符字符串。例如,可以在每个文件内容后添加一行“— 分隔 —”,这样在查看合并后的文件时,能够清晰区分不同文件的内容。
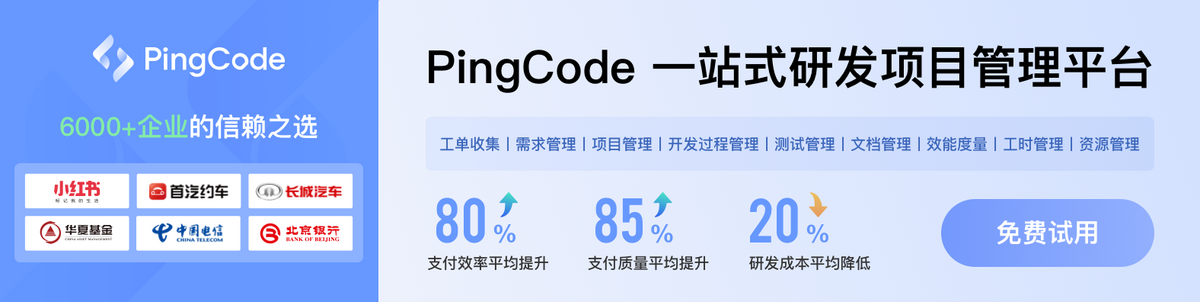