Python调用父类中的属性,可以使用super()函数、直接使用父类名调用属性、使用子类对象访问父类属性。其中,最常用的方法是使用super()函数。这种方法不仅简洁,而且更安全,因为它可以自动处理多重继承的情况。
一、使用super()函数调用父类属性
Python中的super()
函数是一个内置函数,它返回父类(超类)对象的一个代理对象,从而能够调用父类的方法和属性。下面是一个使用super()
调用父类属性的例子:
class Parent:
def __init__(self):
self.attribute = "I am a parent attribute"
class Child(Parent):
def __init__(self):
super().__init__() # Call the parent class constructor
print(self.attribute)
child = Child()
在这个例子中,Child
类调用了Parent
类的构造函数,并访问了父类的属性attribute
。
二、直接使用父类名调用属性
除了使用super()
函数外,还可以直接使用父类名来调用父类的属性。这种方法虽然不推荐,但在某些特殊情况下可能会用到。
class Parent:
def __init__(self):
self.attribute = "I am a parent attribute"
class Child(Parent):
def __init__(self):
Parent.__init__(self) # Call the parent class constructor
print(self.attribute)
child = Child()
在这个例子中,我们直接使用Parent.__init__(self)
来调用父类的构造函数,并访问父类的属性。
三、使用子类对象访问父类属性
如果父类的属性是公开的,也可以直接通过子类对象来访问父类的属性。
class Parent:
def __init__(self):
self.attribute = "I am a parent attribute"
class Child(Parent):
def __init__(self):
super().__init__() # Call the parent class constructor
child = Child()
print(child.attribute) # Accessing parent class attribute directly
在这个例子中,我们直接通过子类对象child
来访问父类的属性attribute
。
四、在多重继承中的应用
在多重继承的情况下,super()
函数的使用显得尤为重要。它可以确保调用正确的父类方法,避免潜在的冲突。
class A:
def __init__(self):
self.attr_A = "Attribute of A"
class B(A):
def __init__(self):
super().__init__()
self.attr_B = "Attribute of B"
class C(B):
def __init__(self):
super().__init__()
self.attr_C = "Attribute of C"
c = C()
print(c.attr_A) # Accessing attribute from class A
print(c.attr_B) # Accessing attribute from class B
print(c.attr_C) # Accessing attribute from class C
在这个例子中,类C
通过super()
函数依次调用其父类B
和A
的构造函数,并成功访问各个父类的属性。
五、父类属性的保护和私有化
在实际编程中,通常会使用保护(protected)和私有(private)属性来控制属性的访问权限。在Python中,前置一个下划线_
表示保护属性,前置两个下划线__
表示私有属性。
class Parent:
def __init__(self):
self._protected_attribute = "I am a protected attribute"
self.__private_attribute = "I am a private attribute"
class Child(Parent):
def __init__(self):
super().__init__()
print(self._protected_attribute)
# print(self.__private_attribute) # This will raise an AttributeError
child = Child()
在这个例子中,我们可以在子类中访问父类的保护属性,但不能直接访问私有属性。
六、利用getter和setter方法
为了更好地控制父类属性的访问,可以使用getter和setter方法。这不仅提高了代码的安全性,还增强了属性的可维护性。
class Parent:
def __init__(self):
self.__attribute = "I am a private attribute"
def get_attribute(self):
return self.__attribute
def set_attribute(self, value):
self.__attribute = value
class Child(Parent):
def __init__(self):
super().__init__()
child = Child()
print(child.get_attribute()) # Accessing private attribute via getter method
child.set_attribute("New value")
print(child.get_attribute()) # Accessing updated attribute via getter method
在这个例子中,我们通过getter和setter方法来访问和更新父类的私有属性。
七、总结
Python调用父类中的属性有多种方法,最常用的是super()
函数。其他方法包括直接使用父类名调用属性和通过子类对象访问父类属性。在多重继承的情况下,super()
函数显得尤为重要。此外,通过getter和setter方法可以更好地控制父类属性的访问权限。了解并掌握这些方法,有助于编写更健壮和可维护的代码。
相关问答FAQs:
如何在子类中访问父类的属性?
在Python中,子类可以通过self
关键字直接访问父类的属性。如果父类的属性是公开的(没有以双下划线开头),子类可以直接使用self.属性名
的方式访问。如果父类的属性是私有的(以双下划线开头),需要通过父类的方法来访问。
使用super()函数有什么好处?
使用super()
函数可以让你更方便地调用父类的方法和属性。它不仅可以避免直接引用父类的名称,还能支持多重继承,使得代码更加灵活和易于维护。在子类中调用父类的构造函数时,可以使用super().__init__()
来确保父类的初始化逻辑被执行。
如何处理父类中的私有属性?
私有属性通常不建议直接在子类中访问。为了安全和封装,应该在父类中提供公共方法来获取和设置这些私有属性。这样可以确保对属性的访问是受控的,从而维护数据的完整性和一致性。子类可以通过调用这些公共方法来实现对父类私有属性的访问。
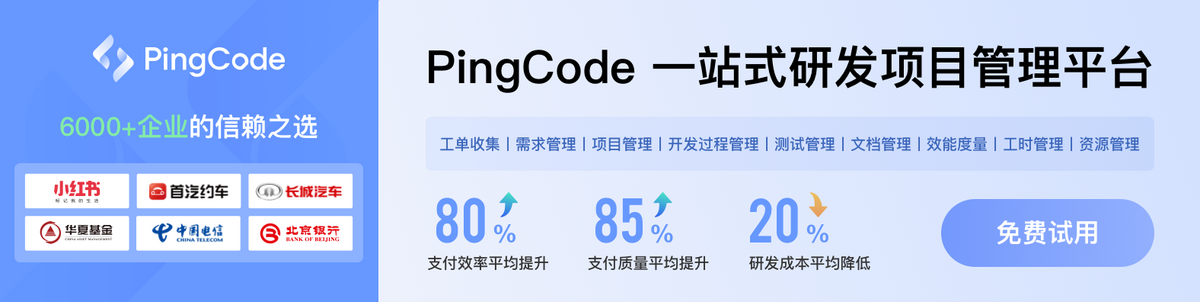