Python3查询可调用函数的方法有多种,包括使用内置函数、模块和工具来确定对象是否可调用、列出模块中的所有可调用函数,以及分析类中的方法。
通过使用内置函数 callable()
, 使用 dir()
列出模块中的所有函数, 使用 inspect
模块列出类中的所有方法,是查询可调用函数的常用方法。这里详细介绍其中的 callable()
方法。
callable()
函数是 Python 的一个内置函数,用于检查对象是否是可调用的。可调用的对象包括函数、方法、类实例和实现了 __call__
方法的对象。当我们传递一个对象给 callable()
函数时,它将返回 True
或 False
,分别表示该对象是否是可调用的。
一、使用 callable()
函数
callable()
函数是确定对象是否可调用的最简单方法。这对于检查函数、方法或其他可调用对象非常有用。
def example_function():
pass
class ExampleClass:
def __call__(self):
pass
example_instance = ExampleClass()
print(callable(example_function)) # 输出: True
print(callable(ExampleClass)) # 输出: True
print(callable(example_instance)) # 输出: True
print(callable(42)) # 输出: False
在上面的例子中,example_function
是一个函数,因此是可调用的。ExampleClass
是一个类,并且实现了 __call__
方法,所以它的实例 example_instance
也是可调用的。然而,整数 42
不是可调用的,因此 callable(42)
返回 False
。
二、使用 dir()
列出模块中的所有函数
dir()
函数返回指定对象的所有属性和方法的列表,包括模块中的所有函数。我们可以过滤这些结果,以仅保留可调用的对象。
import math
functions = [func for func in dir(math) if callable(getattr(math, func))]
print(functions)
在这个例子中,我们导入了 math
模块,并使用列表推导式来筛选出所有可调用的对象。getattr(math, func)
获取对象的属性值,然后使用 callable()
来检查它是否可调用。
三、使用 inspect
模块列出类中的所有方法
inspect
模块提供了多个函数来获取对象的有用信息。我们可以使用 inspect.getmembers()
函数来获取类的所有成员,并筛选出可调用的方法。
import inspect
class ExampleClass:
def method_one(self):
pass
def method_two(self):
pass
methods = [member for member, value in inspect.getmembers(ExampleClass) if callable(value)]
print(methods)
在这个例子中,我们定义了一个 ExampleClass
,并使用 inspect.getmembers()
函数获取其所有成员。然后,我们筛选出所有可调用的成员,这些成员即为类的方法。
四、使用 types
模块检查特定类型的可调用对象
types
模块定义了多个与 Python 对象类型相关的名称。我们可以使用这些名称来检查特定类型的可调用对象。
import types
def example_function():
pass
generator_expression = (x for x in range(10))
print(isinstance(example_function, types.FunctionType)) # 输出: True
print(isinstance(generator_expression, types.GeneratorType)) # 输出: True
在这个例子中,我们使用 types.FunctionType
来检查对象是否是函数,并使用 types.GeneratorType
来检查对象是否是生成器表达式。
五、使用 __call__
方法
在 Python 中,类实例可以通过实现 __call__
方法变为可调用。这允许我们创建行为类似于函数的对象。
class CallableClass:
def __call__(self):
print("Instance is called")
instance = CallableClass()
instance() # 输出: Instance is called
print(callable(instance)) # 输出: True
在这个例子中,我们定义了一个 CallableClass
,并在其 __call__
方法中定义了行为。实例化该类后,实例 instance
就变为了可调用对象。
六、结合 getattr()
和 hasattr()
函数
getattr()
函数用于获取对象的属性值,而 hasattr()
函数用于检查对象是否具有指定属性。我们可以结合这两个函数来检查对象是否具有特定的可调用方法。
class ExampleClass:
def example_method(self):
pass
example_instance = ExampleClass()
if hasattr(example_instance, 'example_method') and callable(getattr(example_instance, 'example_method')):
print("example_method is callable")
else:
print("example_method is not callable")
在这个例子中,我们使用 hasattr()
函数检查 example_instance
是否具有 example_method
属性,然后使用 getattr()
函数获取该属性的值,并使用 callable()
函数检查其是否可调用。
七、使用 __code__
属性检查函数信息
函数对象具有一个名为 __code__
的属性,它包含函数的字节码和其他信息。我们可以使用这个属性来检查函数的参数和其他详细信息。
def example_function(param1, param2):
pass
code = example_function.__code__
print(code.co_varnames) # 输出: ('param1', 'param2')
print(code.co_argcount) # 输出: 2
在这个例子中,我们获取了 example_function
的 __code__
属性,并输出了函数的参数名称和参数数量。
八、使用 dis
模块反编译函数字节码
dis
模块用于反编译 Python 字节码,可以帮助我们分析函数的内部结构。
import dis
def example_function(param1, param2):
result = param1 + param2
return result
dis.dis(example_function)
在这个例子中,我们定义了一个简单的函数 example_function
,并使用 dis.dis()
函数反编译其字节码,输出结果将显示函数的详细操作步骤。
九、使用 functools
模块的工具
functools
模块提供了多个高阶函数和可调用对象的工具。例如,我们可以使用 functools.partial
创建部分可调用对象。
from functools import partial
def example_function(param1, param2):
return param1 + param2
partial_function = partial(example_function, param1=10)
print(partial_function(param2=5)) # 输出: 15
在这个例子中,我们使用 functools.partial
创建了一个部分可调用对象 partial_function
,将 param1
参数固定为 10
。
十、总结
通过以上方法,我们可以灵活地查询和检查 Python 中的可调用函数和对象。无论是使用内置函数、模块工具,还是反编译和分析函数字节码,这些方法都提供了强大的功能,帮助我们深入理解和操作 Python 中的可调用对象。
相关问答FAQs:
在Python3中,如何查看一个对象的可调用属性?
可以使用内置的callable()
函数来检查一个对象是否可调用。只需将对象作为参数传递给callable()
,如果返回True
,则表示该对象是可调用的。例如:
def my_function():
pass
print(callable(my_function)) # 输出: True
如何获取一个模块中所有可调用的函数?
可以使用inspect
模块来查找模块中的可调用对象。首先导入模块并使用inspect.getmembers()
配合inspect.isfunction()
,这样可以列出模块中所有的函数。示例代码如下:
import inspect
import some_module # 替换为实际模块名
functions_list = inspect.getmembers(some_module, inspect.isfunction)
print(functions_list)
怎样查询一个类中的可调用方法?
使用dir()
函数可以列出类的所有属性和方法。然后可以使用inspect
模块过滤出可调用的方法。以下是一个示例:
import inspect
class MyClass:
def method_one(self):
pass
def method_two(self):
pass
methods_list = [method for method in dir(MyClass) if callable(getattr(MyClass, method)) and not method.startswith("__")]
print(methods_list)
如何识别一个函数的参数和返回值类型?
可以使用inspect.signature()
函数来获取函数的签名信息,包括参数和返回值类型。以下是一个例子:
import inspect
def example_function(a: int, b: str) -> bool:
return True
signature = inspect.signature(example_function)
print(signature) # 输出: (a: int, b: str) -> bool
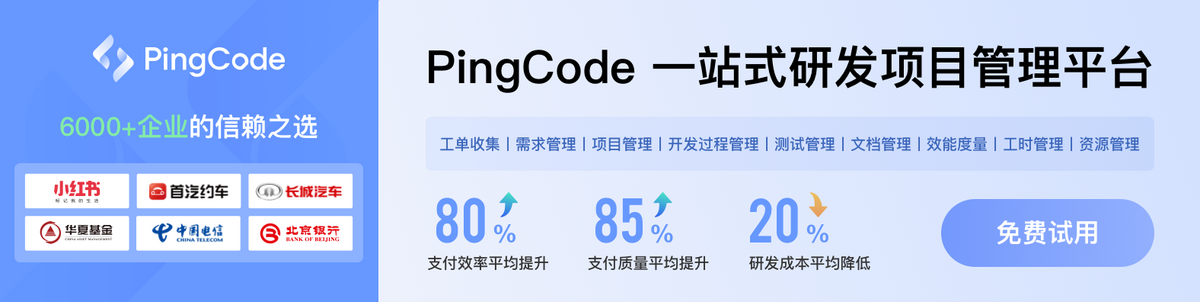