Python序列化对象到文件的几种方法包括:使用pickle模块、使用json模块、使用shelve模块。本文将重点介绍这几种方法,并详细描述如何使用它们将Python对象序列化到文件中。
一、PICKLE模块
1、介绍
Pickle是Python内置的模块之一,用于将Python对象序列化成字节流,并将其写入文件或其他数据流中。反序列化则是从字节流中恢复Python对象。Pickle模块可以序列化几乎所有的Python对象,包括自定义类的实例。
2、序列化与反序列化
使用pickle模块进行序列化和反序列化非常简单,下面是一个基本的例子:
import pickle
定义一个简单的类
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
创建一个Person对象
person = Person("Alice", 30)
序列化对象到文件
with open("person.pkl", "wb") as file:
pickle.dump(person, file)
反序列化对象从文件
with open("person.pkl", "rb") as file:
loaded_person = pickle.load(file)
print(f"Name: {loaded_person.name}, Age: {loaded_person.age}")
在这个例子中,我们创建了一个名为Person
的类,并创建了一个Person
对象。然后使用pickle.dump()
方法将对象序列化并写入文件person.pkl
。接着使用pickle.load()
方法从文件中读取对象并反序列化。
3、使用注意事项
虽然pickle模块非常方便,但也有一些需要注意的事项:
- 安全性:不要反序列化来自不信任或不安全来源的数据,因为pickle模块可以执行任意代码。
- 兼容性:Pickle数据格式在不同Python版本之间可能不兼容,因此在不同版本的Python之间传递数据时需要注意。
二、JSON模块
1、介绍
JSON(JavaScript Object Notation)是一种轻量级的数据交换格式,非常易于人类阅读和编写,同时也易于机器解析和生成。Python的json模块提供了将Python对象转换为JSON格式的功能,反之亦然。
2、序列化与反序列化
使用json模块进行序列化和反序列化也很简单,下面是一个基本的例子:
import json
定义一个简单的类
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
创建一个Person对象
person = Person("Alice", 30)
将对象转换为字典
person_dict = {"name": person.name, "age": person.age}
序列化对象到文件
with open("person.json", "w") as file:
json.dump(person_dict, file)
反序列化对象从文件
with open("person.json", "r") as file:
loaded_person_dict = json.load(file)
loaded_person = Person(loaded_person_dict)
print(f"Name: {loaded_person.name}, Age: {loaded_person.age}")
在这个例子中,我们将Person
对象转换为字典,然后使用json.dump()
方法将字典序列化并写入文件person.json
。接着使用json.load()
方法从文件中读取字典并反序列化。
3、自定义类的序列化
由于JSON模块不能直接序列化自定义类的实例,因此需要将自定义类的实例转换为字典或使用自定义的序列化函数。例如,可以使用类的__dict__
属性来获取对象的属性字典:
# 序列化对象到文件
with open("person.json", "w") as file:
json.dump(person.__dict__, file)
三、SHELVE模块
1、介绍
Shelve模块提供了一个简单的持久化存储方法,可以将Python对象存储在一个类似于数据库的文件中。Shelve模块实际上使用pickle模块来序列化对象,并使用dbm模块来存储字节流。
2、序列化与反序列化
使用shelve模块进行序列化和反序列化也很简单,下面是一个基本的例子:
import shelve
定义一个简单的类
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
创建一个Person对象
person = Person("Alice", 30)
序列化对象到文件
with shelve.open("person_shelve.db") as db:
db["person"] = person
反序列化对象从文件
with shelve.open("person_shelve.db") as db:
loaded_person = db["person"]
print(f"Name: {loaded_person.name}, Age: {loaded_person.age}")
在这个例子中,我们使用shelve.open()
方法打开一个数据库文件person_shelve.db
,然后将Person
对象存储在数据库中。接着,我们再次打开数据库并读取对象。
3、使用注意事项
- 文件锁定:Shelve模块在多线程或多进程环境中使用时需要注意文件锁定问题,以防止数据损坏。
- 性能:Shelve模块适用于存储中小规模的数据,对于大型数据集可能会有性能问题。
四、其他序列化方法
除了上述几种常用的序列化方法,Python还有其他序列化方法,如使用第三方库(如msgpack
、cbor
等)进行序列化。这些库通常具有更高的性能或更紧凑的存储格式,可以根据具体需求选择合适的库。
1、Msgpack
Msgpack(MessagePack)是一种高效的二进制序列化格式,比JSON更紧凑。使用msgpack库可以高效地序列化和反序列化Python对象。
import msgpack
定义一个简单的类
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
创建一个Person对象
person = Person("Alice", 30)
将对象转换为字典
person_dict = {"name": person.name, "age": person.age}
序列化对象到文件
with open("person.msgpack", "wb") as file:
file.write(msgpack.packb(person_dict))
反序列化对象从文件
with open("person.msgpack", "rb") as file:
loaded_person_dict = msgpack.unpackb(file.read())
loaded_person = Person(loaded_person_dict)
print(f"Name: {loaded_person.name}, Age: {loaded_person.age}")
2、CBOR
CBOR(Concise Binary Object Representation)是一种紧凑的二进制数据格式,类似于JSON,但更适合于机器解析和生成。
import cbor
定义一个简单的类
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
创建一个Person对象
person = Person("Alice", 30)
将对象转换为字典
person_dict = {"name": person.name, "age": person.age}
序列化对象到文件
with open("person.cbor", "wb") as file:
file.write(cbor.dumps(person_dict))
反序列化对象从文件
with open("person.cbor", "rb") as file:
loaded_person_dict = cbor.loads(file.read())
loaded_person = Person(loaded_person_dict)
print(f"Name: {loaded_person.name}, Age: {loaded_person.age}")
五、总结
在Python中,将对象序列化到文件的方法有很多,每种方法都有其优缺点。pickle模块适用于序列化几乎所有的Python对象,但在安全性和兼容性方面需要注意;json模块适用于与其他语言进行数据交换,但不能直接序列化自定义类的实例;shelve模块提供了一个简单的持久化存储方法,但在多线程或多进程环境中需要注意文件锁定问题;msgpack和cbor等第三方库则提供了更高效或更紧凑的序列化方法。
根据具体的需求,选择合适的序列化方法可以提高数据存储和传输的效率,同时保证数据的完整性和安全性。
相关问答FAQs:
如何在Python中实现对象的序列化?
在Python中,可以使用pickle
模块来实现对象的序列化。该模块提供了dump
和load
函数,可以将对象序列化为字节流,并保存到文件中。示例代码如下:
import pickle
# 定义一个示例对象
data = {'name': 'Alice', 'age': 30}
# 将对象序列化并写入文件
with open('data.pkl', 'wb') as file:
pickle.dump(data, file)
通过以上方法,您可以将任何Python对象(如列表、字典、自定义类等)序列化并保存到文件中。
序列化对象后如何反序列化回Python对象?
反序列化是指将序列化的字节流转换回Python对象。使用pickle
模块的load
函数可以轻松实现。以下是反序列化的示例代码:
import pickle
# 从文件中读取序列化的对象
with open('data.pkl', 'rb') as file:
loaded_data = pickle.load(file)
print(loaded_data) # 输出: {'name': 'Alice', 'age': 30}
通过这种方式,您可以恢复之前保存的对象,便于后续处理。
使用pickle
序列化时有哪些注意事项?
在使用pickle
模块进行序列化时,应注意以下几点:
- 安全性:从不可信来源加载的数据可能会导致安全风险,建议仅加载可信数据。
- 兼容性:不同版本的Python可能会导致序列化文件不兼容,因此在不同环境中使用时需要谨慎。
- 性能:对于大型对象,序列化和反序列化可能会耗费较多时间和内存,选择合适的数据格式(如JSON)可能会更高效。
了解这些注意事项可以帮助您更好地使用对象序列化功能。
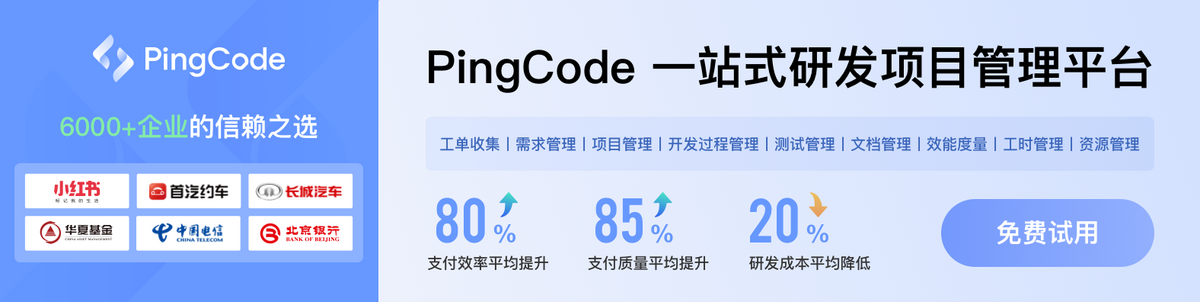