使用Python爬取网页源代码的方法有几种,最常见的有以下几种:使用requests库、使用urllib库、使用selenium库。 其中,使用requests库是最简单且高效的方法,因为它易于使用且速度快。使用requests库可以轻松发送HTTP请求,并获取网页的HTML源代码。
下面详细介绍如何使用requests库来爬取网页源代码:
一、使用Requests库
Requests库是一个强大且用户友好的HTTP库,适用于Python。它可以轻松发送HTTP请求,并获取网页的HTML源代码。
安装Requests库
首先,确保您已安装Requests库。如果尚未安装,可以使用pip进行安装:
pip install requests
使用Requests库获取网页源代码
下面是一个简单的示例,展示了如何使用Requests库获取网页的HTML源代码:
import requests
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print(f'Failed to retrieve the webpage. Status code: {response.status_code}')
在上面的示例中,我们使用requests.get()
方法发送HTTP GET请求,并获取响应对象。然后,我们检查响应状态码是否为200(表示请求成功),如果成功,则打印网页的HTML源代码。
处理请求头
有时,您可能需要发送自定义请求头,例如User-Agent,以模拟浏览器行为。可以通过传递headers
参数来实现:
import requests
url = 'https://www.example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
response = requests.get(url, headers=headers)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print(f'Failed to retrieve the webpage. Status code: {response.status_code}')
在这个示例中,我们添加了User-Agent
头部,使请求看起来像是来自实际浏览器。这有助于避免某些网站阻止非浏览器请求。
处理Cookies
有些网站可能需要处理Cookies才能正常访问。Requests库可以轻松管理Cookies:
import requests
url = 'https://www.example.com'
session = requests.Session()
response = session.get(url)
if response.status_code == 200:
html_content = response.text
print(html_content)
else:
print(f'Failed to retrieve the webpage. Status code: {response.status_code}')
在这个示例中,我们创建了一个Session
对象,以便在后续请求中自动管理Cookies。
二、使用Urllib库
Urllib库是Python内置的HTTP库,适用于发送HTTP请求和处理响应。虽然Urllib库功能强大,但使用起来稍微复杂一些。
使用Urllib库获取网页源代码
下面是一个使用Urllib库的示例:
import urllib.request
url = 'https://www.example.com'
response = urllib.request.urlopen(url)
html_content = response.read().decode('utf-8')
print(html_content)
在这个示例中,我们使用urllib.request.urlopen()
方法发送HTTP GET请求,并获取响应对象。然后,我们读取响应内容并解码为字符串。
处理请求头
与Requests库类似,我们可以通过Request
对象添加自定义请求头:
import urllib.request
url = 'https://www.example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/91.0.4472.124 Safari/537.36'
}
request = urllib.request.Request(url, headers=headers)
response = urllib.request.urlopen(request)
html_content = response.read().decode('utf-8')
print(html_content)
在这个示例中,我们创建了一个Request
对象,并添加了自定义请求头。
处理Cookies
Urllib库也可以处理Cookies,但需要使用http.cookiejar
模块:
import urllib.request
import http.cookiejar
url = 'https://www.example.com'
cookie_jar = http.cookiejar.CookieJar()
opener = urllib.request.build_opener(urllib.request.HTTPCookieProcessor(cookie_jar))
response = opener.open(url)
html_content = response.read().decode('utf-8')
print(html_content)
在这个示例中,我们创建了一个CookieJar
对象,并使用它来处理Cookies。
三、使用Selenium库
Selenium库适用于需要与JavaScript交互或模拟浏览器行为的复杂网页。它可以控制实际的浏览器(如Chrome、Firefox),并执行各种操作。
安装Selenium库和WebDriver
首先,确保您已安装Selenium库和相应的WebDriver。以Chrome为例:
pip install selenium
下载ChromeDriver并将其添加到系统路径:https://sites.google.com/a/chromium.org/chromedriver/
使用Selenium库获取网页源代码
下面是一个使用Selenium库的示例:
from selenium import webdriver
url = 'https://www.example.com'
driver_path = '/path/to/chromedriver'
driver = webdriver.Chrome(executable_path=driver_path)
driver.get(url)
html_content = driver.page_source
print(html_content)
driver.quit()
在这个示例中,我们创建了一个Chrome浏览器实例,并使用driver.get()
方法导航到指定URL。然后,我们获取网页的HTML源代码,并打印出来。最后,关闭浏览器实例。
处理动态内容和等待
Selenium库可以处理动态内容和等待页面加载完成。可以使用WebDriverWait
进行显式等待:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
url = 'https://www.example.com'
driver_path = '/path/to/chromedriver'
driver = webdriver.Chrome(executable_path=driver_path)
driver.get(url)
wait = WebDriverWait(driver, 10)
element = wait.until(EC.presence_of_element_located((By.ID, 'element_id')))
html_content = driver.page_source
print(html_content)
driver.quit()
在这个示例中,我们使用WebDriverWait
等待特定元素加载完成,然后获取网页的HTML源代码。
四、使用BeautifulSoup解析HTML
无论使用Requests、Urllib还是Selenium获取网页源代码,解析和提取网页内容通常需要使用BeautifulSoup库。
安装BeautifulSoup
首先,确保您已安装BeautifulSoup库:
pip install beautifulsoup4
使用BeautifulSoup解析HTML
下面是一个使用BeautifulSoup解析HTML的示例:
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
print(soup.prettify())
else:
print(f'Failed to retrieve the webpage. Status code: {response.status_code}')
在这个示例中,我们使用BeautifulSoup
解析获取的HTML源代码,并使用prettify()
方法格式化输出。
提取特定元素
使用BeautifulSoup可以轻松提取特定元素,例如标题、链接等:
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
if response.status_code == 200:
html_content = response.text
soup = BeautifulSoup(html_content, 'html.parser')
# 提取标题
title = soup.title.string
print(f'Title: {title}')
# 提取所有链接
links = soup.find_all('a')
for link in links:
print(link.get('href'))
else:
print(f'Failed to retrieve the webpage. Status code: {response.status_code}')
在这个示例中,我们提取了网页的标题和所有链接,并逐一打印链接URL。
总结:
使用Python爬取网页源代码的方法有多种,最常见的有:使用Requests库、使用Urllib库、使用Selenium库。每种方法都有其适用场景和优势。Requests库适用于简单且高效的HTTP请求,Urllib库是内置的HTTP库,功能强大但稍微复杂,Selenium库适用于需要与JavaScript交互或模拟浏览器行为的复杂网页。结合使用BeautifulSoup库,可以轻松解析和提取网页内容。根据具体需求选择合适的方法,可以高效完成网页源代码的爬取任务。
相关问答FAQs:
如何选择合适的库来爬取网页源代码?
Python中有多个库可以用于网页爬取,如Requests和BeautifulSoup。Requests库用于发送HTTP请求,获取网页内容,而BeautifulSoup则用于解析HTML文档。选择合适的库取决于你的需求,如果只需要获取网页源代码,Requests就足够了。如果需要提取特定数据,则BeautifulSoup会更为有效。
在爬取网页时,如何处理反爬虫机制?
许多网站会采取反爬虫措施,防止自动化程序访问其内容。为了绕过这些限制,可以使用随机的User-Agent来伪装成浏览器请求,增加请求间隔以降低访问频率,或者使用代理IP来隐藏真实IP地址。这些方法可以提高爬取成功的概率。
如何保存爬取到的网页源代码?
在使用Python爬取网页源代码后,可以将其保存为文本文件。可以使用Python的内置函数open()来创建文件,并使用write()方法将源代码写入文件中。确保在保存文件时使用合适的编码格式,例如UTF-8,以避免字符编码问题。
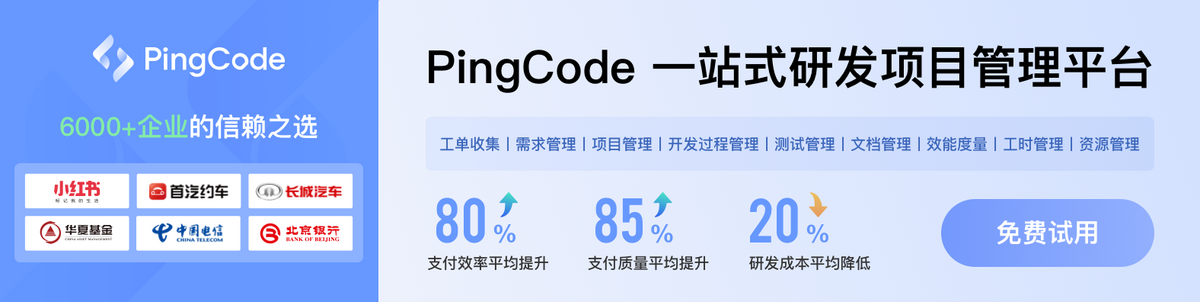