判断Python中的字符串的方法有:使用内置函数 type() 检查类型、使用 isinstance() 函数判断类型、使用正则表达式验证字符串内容。
其中,使用 isinstance() 函数判断类型 是一个非常常见且简便的方法。isinstance() 函数是 Python 提供的一个内置函数,它可以用来判断一个对象是否是已知的某种数据类型。它的语法是 isinstance(object, classinfo)
,其中 object
是你需要检查的对象,classinfo
是你期望的类型。在判断字符串时,classinfo
可以是 str
。例如:isinstance("Hello, World!", str)
会返回 True
,表明这是一个字符串。
以下将详细介绍上述几种方法,并探讨更多关于字符串判断的相关内容。
一、使用 type() 检查类型
type()
是 Python 的内置函数,用于返回对象的类型。我们可以利用 type()
函数来检查一个变量是否是字符串。
example = "Hello, World!"
if type(example) is str:
print("This is a string.")
else:
print("This is not a string.")
在这个例子中,type(example)
会返回 str
,所以输出会是 "This is a string."。这个方法直观且易于理解,但在一些情况下可能不如 isinstance()
方便。
二、使用 isinstance() 函数判断类型
isinstance()
是一个更强大的函数,可以判断一个对象是否是某种类型或某个类型的子类。
example = "Hello, World!"
if isinstance(example, str):
print("This is a string.")
else:
print("This is not a string.")
与 type()
不同,isinstance()
还可以判断一个对象是否是某个类的子类。比如,如果你定义了一个新的字符串类,isinstance()
也能正确判断。
class MyStr(str):
pass
example = MyStr("Hello, World!")
print(isinstance(example, str)) # True
三、使用正则表达式验证字符串内容
正则表达式(regular expression,regex)是用于匹配字符串的一种模式。你可以使用正则表达式来验证一个字符串是否符合特定格式。
import re
example = "Hello, World!"
pattern = r"^[A-Za-z\s,]+$"
if re.match(pattern, example):
print("This is a valid string.")
else:
print("This is not a valid string.")
在这个例子中,re.match()
函数会检查 example
是否符合 pattern
。pattern
是一个正则表达式,^[A-Za-z\s,]+$
表示字符串只能包含字母、空格和逗号。
四、字符串判断的更多细节
1、处理不同数据类型的输入
在实际编程中,你可能会遇到各种数据类型的输入。为了确保程序的健壮性,你需要处理这些不同类型的数据。
def check_string(input_data):
if isinstance(input_data, str):
print("This is a string.")
else:
print("This is not a string.")
check_string("Hello, World!") # This is a string.
check_string(12345) # This is not a string.
check_string([1, 2, 3]) # This is not a string.
2、处理编码问题
在处理字符串时,编码问题可能会引起一些困扰。Python 3 默认使用 Unicode 字符串,但在处理来自不同来源的数据时,仍然可能会遇到编码问题。
example = b"Hello, World!"
if isinstance(example, bytes):
example = example.decode('utf-8')
if isinstance(example, str):
print("This is a string.")
else:
print("This is not a string.")
在这个例子中,如果 example
是一个字节串(bytes),我们会先将其解码为字符串(str),然后再进行判断。
五、使用第三方库
除了 Python 内置的方法外,一些第三方库也提供了强大的字符串处理功能。例如,six
库可以帮助你编写兼容 Python 2 和 Python 3 的代码。
import six
example = "Hello, World!"
if isinstance(example, six.string_types):
print("This is a string.")
else:
print("This is not a string.")
在这个例子中,six.string_types
在 Python 3 中相当于 (str,)
,在 Python 2 中相当于 (basestring,)
。使用 six
库可以让你的代码更加兼容不同的 Python 版本。
六、字符串的常见操作
在实际编程中,判断一个字符串是否为字符串只是第一步。你还需要对字符串进行各种操作。以下是一些常见的字符串操作及其实现方法。
1、字符串拼接
在 Python 中,你可以使用 +
操作符或 join()
方法来拼接字符串。
str1 = "Hello"
str2 = "World"
使用 + 操作符
result = str1 + ", " + str2 + "!"
print(result) # Hello, World!
使用 join() 方法
result = ", ".join([str1, str2]) + "!"
print(result) # Hello, World!
2、字符串分割
你可以使用 split()
方法将字符串按指定分隔符分割成一个列表。
example = "Hello, World!"
result = example.split(", ")
print(result) # ['Hello', 'World!']
3、字符串替换
你可以使用 replace()
方法将字符串中的某些部分替换为新的内容。
example = "Hello, World!"
result = example.replace("World", "Python")
print(result) # Hello, Python!
4、字符串查找
你可以使用 find()
或 index()
方法在字符串中查找子字符串的位置。
example = "Hello, World!"
position = example.find("World")
print(position) # 7
如果子字符串不存在,find() 返回 -1,而 index() 会引发 ValueError
position = example.find("Python")
print(position) # -1
position = example.index("Python") # ValueError: substring not found
5、字符串格式化
你可以使用 format()
方法或 f-string 来格式化字符串。
name = "World"
result = "Hello, {}!".format(name)
print(result) # Hello, World!
使用 f-string(Python 3.6 及以上版本)
result = f"Hello, {name}!"
print(result) # Hello, World!
七、总结
在 Python 中判断字符串的方法有多种,主要包括使用 type()
函数、isinstance()
函数和正则表达式等。其中,使用 isinstance()
函数判断类型是一种高效且便捷的方法。此外,在实际编程中,你还需要处理不同数据类型的输入、编码问题以及进行各种字符串操作。通过掌握这些技巧,你可以更好地处理和操作字符串,提高程序的健壮性和可读性。
相关问答FAQs:
如何在Python中检查字符串的类型和内容?
在Python中,可以使用内置函数isinstance()
来判断一个变量是否为字符串类型。通过传入变量和str
类型,可以快速判断。例如:isinstance(my_variable, str)
。此外,可以使用type(my_variable)
来获取变量的类型。
如何处理和比较Python字符串?
字符串比较可以使用==
运算符来判断两个字符串是否相等。对于大小写不敏感的比较,可以使用.lower()
或.upper()
方法将字符串转换为统一的格式再进行比较。此外,startswith()
和endswith()
方法可以用来检查字符串是否以特定子串开头或结尾。
在Python中如何检测字符串的特定模式或内容?
可以使用正则表达式来检测字符串中的特定模式。Python的re
模块提供了多种方法,例如re.search()
可以用于查找字符串中是否存在某个模式,re.match()
则用于检查字符串的开头部分是否符合某个模式。此外,字符串本身也有很多内置方法,如in
运算符可以用来判断一个子串是否在字符串中。
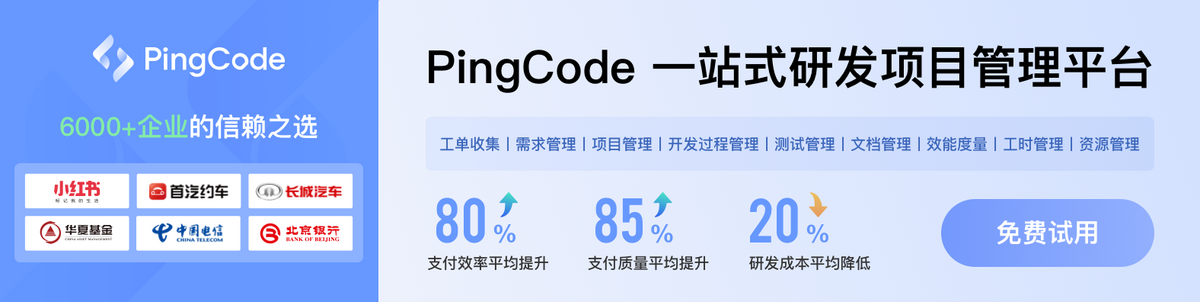