如何用Python调用CMD命令行
使用Python调用CMD命令行有几种方法:os.system()、subprocess模块、popen()方法、run()函数。
在这里我们详细描述如何使用subprocess模块来调用命令行,因为subprocess模块是处理子进程的一个强大工具,提供了更强的灵活性和更好的错误处理机制。
一、subprocess模块
subprocess模块是Python 3.5之后引入的一个更强大的替代品,用于处理子进程的创建和管理。它允许你启动一个新的进程,连接其输入/输出/错误管道,并获取它的返回码。
1、使用subprocess.run()函数
subprocess.run()是最常用的函数之一,它运行一个命令并等待它完成。以下是一个示例:
import subprocess
result = subprocess.run(['dir'], shell=True, capture_output=True, text=True)
print(result.stdout)
在这个示例中,我们使用subprocess.run()函数运行了dir命令,并将其输出捕获到result.stdout中。需要注意的是,使用shell=True参数将命令传递给shell解释器。
2、使用subprocess.Popen()方法
subprocess.Popen()方法提供了更多的灵活性,可以让我们同时处理输入和输出。以下是一个示例:
import subprocess
process = subprocess.Popen(['dir'], shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print(stdout)
print(stderr)
在这个示例中,我们使用subprocess.Popen()方法启动了dir命令,并捕获了它的标准输出和错误输出。
二、os.system()
os.system()是一个简单但功能有限的函数,它只能运行命令并返回其退出状态。以下是一个示例:
import os
exit_code = os.system('dir')
print('Exit code:', exit_code)
尽管os.system()使用起来非常简单,但它不推荐用于复杂的任务,因为它缺乏灵活性和错误处理机制。
三、捕获输出
有时,我们需要捕获命令的输出,subprocess模块提供了几种方法来实现这一点:
1、capture_output参数
在subprocess.run()中,使用capture_output=True参数可以捕获命令的输出。以下是一个示例:
import subprocess
result = subprocess.run(['dir'], shell=True, capture_output=True, text=True)
print(result.stdout)
2、stdout和stderr参数
在subprocess.Popen()中,使用stdout和stderr参数可以捕获命令的标准输出和错误输出。以下是一个示例:
import subprocess
process = subprocess.Popen(['dir'], shell=True, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print(stdout)
print(stderr)
四、处理错误
处理错误是编写健壮代码的关键。subprocess模块提供了几种方法来处理错误:
1、检查返回码
在subprocess.run()中,可以通过检查返回码来判断命令是否成功。以下是一个示例:
import subprocess
result = subprocess.run(['dir'], shell=True, capture_output=True, text=True)
if result.returncode == 0:
print('Command succeeded')
else:
print('Command failed with return code', result.returncode)
2、捕获异常
在subprocess.run()中,可以通过捕获异常来处理错误。以下是一个示例:
import subprocess
try:
result = subprocess.run(['dir'], shell=True, capture_output=True, text=True, check=True)
print('Command succeeded')
except subprocess.CalledProcessError as e:
print('Command failed with return code', e.returncode)
在这个示例中,我们使用了check=True参数,它会在命令失败时引发CalledProcessError异常。
五、传递输入
有时,我们需要向命令传递输入。subprocess模块提供了几种方法来实现这一点:
1、input参数
在subprocess.run()中,使用input参数可以向命令传递输入。以下是一个示例:
import subprocess
result = subprocess.run(['python'], input='print("Hello, world!")', shell=True, capture_output=True, text=True)
print(result.stdout)
2、stdin参数
在subprocess.Popen()中,使用stdin参数可以向命令传递输入。以下是一个示例:
import subprocess
process = subprocess.Popen(['python'], shell=True, stdin=subprocess.PIPE, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate(input='print("Hello, world!")')
print(stdout)
print(stderr)
六、示例:运行批处理文件
有时,我们需要运行批处理文件。以下是一个示例:
import subprocess
result = subprocess.run(['path/to/your/batchfile.bat'], shell=True, capture_output=True, text=True)
print(result.stdout)
在这个示例中,我们使用subprocess.run()运行了一个批处理文件,并捕获了它的输出。
七、示例:运行带参数的命令
有时,我们需要运行带参数的命令。以下是一个示例:
import subprocess
result = subprocess.run(['python', '-c', 'print("Hello, world!")'], shell=True, capture_output=True, text=True)
print(result.stdout)
在这个示例中,我们使用subprocess.run()运行了一个带参数的命令,并捕获了它的输出。
八、示例:设置环境变量
有时,我们需要设置环境变量。以下是一个示例:
import subprocess
import os
my_env = os.environ.copy()
my_env['MY_VARIABLE'] = 'my_value'
result = subprocess.run(['python', '-c', 'import os; print(os.getenv("MY_VARIABLE"))'], shell=True, capture_output=True, text=True, env=my_env)
print(result.stdout)
在这个示例中,我们设置了一个环境变量MY_VARIABLE,并在子进程中读取它。
九、示例:重定向输出到文件
有时,我们需要将输出重定向到文件。以下是一个示例:
import subprocess
with open('output.txt', 'w') as f:
result = subprocess.run(['dir'], shell=True, stdout=f, text=True)
在这个示例中,我们将命令的输出重定向到了一个文件output.txt中。
十、示例:在后台运行命令
有时,我们需要在后台运行命令。以下是一个示例:
import subprocess
process = subprocess.Popen(['dir'], shell=True)
在这个示例中,我们使用subprocess.Popen()在后台运行了一个命令。
总结
通过以上的详细介绍,我们了解了如何使用Python调用CMD命令行,包括使用subprocess模块、os.system()、捕获输出、处理错误、传递输入、运行批处理文件、运行带参数的命令、设置环境变量、重定向输出到文件和在后台运行命令。这些技巧和示例将帮助我们在Python中更有效地处理命令行任务。
相关问答FAQs:
如何在Python中执行系统命令?
在Python中,可以使用subprocess
模块来执行系统命令。这个模块提供了强大的功能,能够启动新的进程并与其进行交互。具体而言,可以使用subprocess.run()
方法来运行命令并获取输出。例如,subprocess.run(['cmd', '/c', 'your_command'], capture_output=True, text=True)
可以帮助你执行cmd命令并捕获输出。
在Windows和Linux系统中执行命令有什么区别?
Windows系统常用的命令行工具是cmd,而Linux系统则使用bash或sh。具体在Python中调用命令时,Windows命令通常需要使用cmd /c
前缀来执行,而Linux命令可以直接调用。了解这些差异可以帮助用户更好地编写跨平台的代码。
如何处理Python中cmd命令的输出和错误?
使用subprocess.run()
时,可以通过capture_output=True
参数捕获标准输出和标准错误输出。输出结果会以result.stdout
和result.stderr
形式返回。处理这些输出可以帮助用户在执行命令时更好地调试和分析结果。对于复杂的命令,建议使用try-except
块来捕获异常,从而确保程序的稳定性。
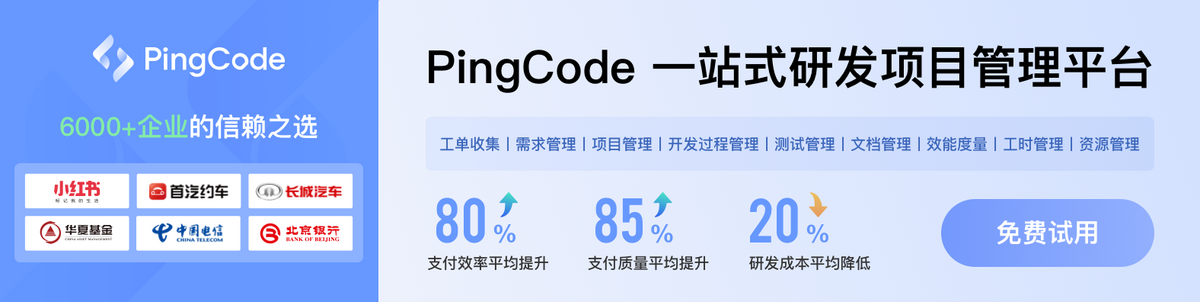