在Python中查看.txt文件编码有几种常见的方法:使用chardet
库、使用cchardet
库、利用BOM(Byte Order Mark)判断编码格式。下面将详细介绍其中一种方法——使用chardet
库。
使用chardet库:
chardet
是一个流行的用于检测文件编码的Python库。它通过分析文件的字节模式来猜测编码。使用chardet
库的步骤如下:
- 安装
chardet
库:
pip install chardet
- 读取文件并检测编码:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
return encoding
file_path = 'example.txt'
encoding = detect_encoding(file_path)
print(f"The encoding of the file is: {encoding}")
详细描述:
chardet
库的detect
方法会返回一个包含编码信息的字典,其中encoding
键对应的值就是文件的编码格式。通过这种方式,可以轻松地检测文件的编码格式。
一、使用chardet库检测文件编码
chardet
库是一个非常强大的工具,可以检测几乎所有常见的编码格式。它的工作原理是通过分析文件的字节模式来猜测编码。以下是详细的步骤和示例代码。
1. 安装chardet库
要使用chardet
库,首先需要安装它。可以使用pip进行安装:
pip install chardet
2. 编写检测编码的函数
下面是一个示例函数,用于检测文件的编码:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
return encoding
这个函数首先以二进制模式打开文件,读取所有内容,然后使用chardet.detect
方法检测编码,最后返回检测到的编码格式。
3. 使用函数检测文件编码
可以使用上面的函数来检测任意.txt文件的编码:
file_path = 'example.txt'
encoding = detect_encoding(file_path)
print(f"The encoding of the file is: {encoding}")
二、使用cchardet库检测文件编码
cchardet
是chardet
的C语言实现版本,速度更快。使用方法与chardet
类似。
1. 安装cchardet库
可以使用pip进行安装:
pip install cchardet
2. 编写检测编码的函数
下面是一个示例函数,用于检测文件的编码:
import cchardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = cchardet.detect(raw_data)
encoding = result['encoding']
return encoding
3. 使用函数检测文件编码
可以使用上面的函数来检测任意.txt文件的编码:
file_path = 'example.txt'
encoding = detect_encoding(file_path)
print(f"The encoding of the file is: {encoding}")
三、利用BOM判断文件编码
BOM(Byte Order Mark)是一种用于标识文本文件编码格式的特殊字符,通常出现在文件开头。通过检查文件的BOM,可以快速判断文件的编码格式。
1. 编写检查BOM的函数
下面是一个示例函数,用于检查文件的BOM:
def check_bom(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read(4)
if raw_data.startswith(b'\xff\xfe\x00\x00'):
return 'utf-32le'
elif raw_data.startswith(b'\x00\x00\xfe\xff'):
return 'utf-32be'
elif raw_data.startswith(b'\xff\xfe'):
return 'utf-16le'
elif raw_data.startswith(b'\xfe\xff'):
return 'utf-16be'
elif raw_data.startswith(b'\xef\xbb\xbf'):
return 'utf-8-sig'
else:
return 'unknown'
这个函数会读取文件的前四个字节,并检查它们是否与某些已知的BOM模式匹配。如果匹配,则返回相应的编码格式。
2. 使用函数检查文件的BOM
可以使用上面的函数来检查任意.txt文件的BOM:
file_path = 'example.txt'
encoding = check_bom(file_path)
print(f"The BOM detected encoding of the file is: {encoding}")
四、结合多种方法提高准确性
为了提高检测编码的准确性,可以将多种方法结合起来使用。首先检查BOM,如果没有检测到BOM,再使用chardet
或cchardet
进行检测。
1. 编写综合检测函数
下面是一个综合检测编码的函数:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
# Check BOM
if raw_data.startswith(b'\xff\xfe\x00\x00'):
return 'utf-32le'
elif raw_data.startswith(b'\x00\x00\xfe\xff'):
return 'utf-32be'
elif raw_data.startswith(b'\xff\xfe'):
return 'utf-16le'
elif raw_data.startswith(b'\xfe\xff'):
return 'utf-16be'
elif raw_data.startswith(b'\xef\xbb\xbf'):
return 'utf-8-sig'
# Use chardet as fallback
result = chardet.detect(raw_data)
encoding = result['encoding']
return encoding
这个函数首先检查文件的BOM,如果没有检测到已知的BOM模式,再使用chardet
进行检测。
2. 使用综合函数检测文件编码
可以使用上面的综合函数来检测任意.txt文件的编码:
file_path = 'example.txt'
encoding = detect_encoding(file_path)
print(f"The detected encoding of the file is: {encoding}")
五、处理不同编码格式的文件
在实际应用中,除了检测文件编码,还需要根据检测到的编码正确处理文件内容。以下是一些常见的处理方式。
1. 读取不同编码格式的文件
可以使用检测到的编码来正确读取文件内容:
def read_file(file_path):
encoding = detect_encoding(file_path)
with open(file_path, 'r', encoding=encoding) as file:
content = file.read()
return content
file_path = 'example.txt'
content = read_file(file_path)
print(content)
2. 转换文件编码
有时需要将文件从一种编码格式转换为另一种编码格式。以下是一个示例函数,用于将文件编码转换为UTF-8:
def convert_to_utf8(file_path, output_path):
encoding = detect_encoding(file_path)
with open(file_path, 'r', encoding=encoding) as file:
content = file.read()
with open(output_path, 'w', encoding='utf-8') as file:
file.write(content)
input_file = 'example.txt'
output_file = 'example_utf8.txt'
convert_to_utf8(input_file, output_file)
六、总结
在Python中查看.txt文件编码有多种方法,其中使用chardet
库、cchardet
库和利用BOM判断编码是最常见的三种方法。通过结合多种方法,可以提高检测编码的准确性。在实际应用中,可以根据检测到的编码正确处理文件内容,甚至将文件从一种编码格式转换为另一种编码格式。通过上述方法,能够有效地解决文件编码问题,提高程序的健壮性和兼容性。
相关问答FAQs:
如何在Python中识别.txt文件的编码格式?
可以使用chardet
库来检测.txt文件的编码。首先,需要安装该库,通过命令pip install chardet
进行安装。接着,可以使用以下代码读取文件并检测编码:
import chardet
with open('your_file.txt', 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
print(result['encoding'])
这样就能获得文件的编码格式了。
是否有其他方法可以查看.txt文件的编码?
除了使用chardet
,你还可以使用cchardet
库,它通常比chardet
更快。安装方式为pip install cchardet
。使用方式与chardet
类似,能快速识别文件编码。
如何避免因编码问题导致的读取错误?
在读取.txt文件时,使用open()
函数时可以指定编码格式,例如utf-8
或gbk
。如果不确定编码,可以先使用上述方法检测编码,然后再选择合适的编码格式读取文件。例如:
with open('your_file.txt', encoding='utf-8') as file:
content = file.read()
这样可以避免因编码不匹配而导致的读取错误。
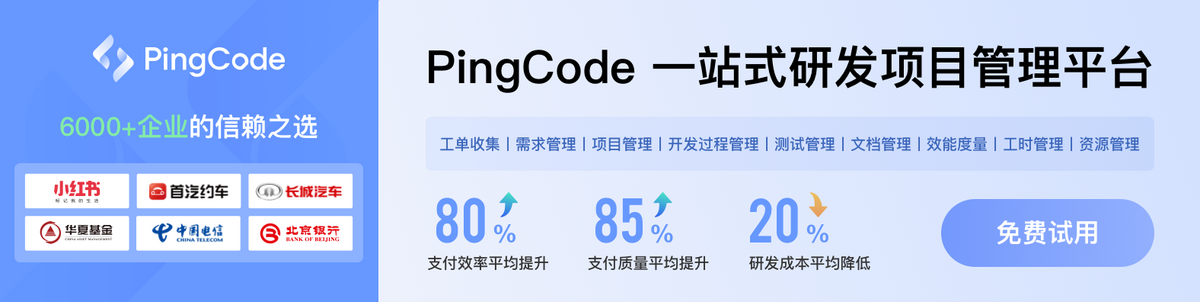