利用Python进行文字匹配,可以通过正则表达式、字符串方法、使用第三方库如difflib等方式来实现。字符串方法简单易用、正则表达式功能强大、difflib库适用于相似度计算。接下来将详细介绍其中的一种方法——正则表达式,它是处理文字匹配的强大工具。
一、正则表达式基础
正则表达式(Regular Expression,简称regex)是一种用于匹配字符串的模式。Python提供了re模块来支持正则表达式操作。通过正则表达式,可以轻松实现复杂的文字匹配和替换任务。
1、基本使用
在Python中使用正则表达式,首先需要导入re模块。re模块提供了几个关键函数:match、search、findall和sub。
import re
match:从字符串的起始位置开始匹配
pattern = r'\d+' # 匹配一个或多个数字
text = '123abc456'
match_obj = re.match(pattern, text)
if match_obj:
print(f"Match found: {match_obj.group()}")
search:扫描整个字符串并返回第一个成功的匹配
search_obj = re.search(pattern, text)
if search_obj:
print(f"Search found: {search_obj.group()}")
findall:返回字符串中所有非重叠匹配的列表
findall_obj = re.findall(pattern, text)
print(f"Findall found: {findall_obj}")
sub:替换字符串中的匹配项
replaced_text = re.sub(pattern, '#', text)
print(f"Sub result: {replaced_text}")
2、元字符与模式
正则表达式中的元字符可以用来构建复杂的匹配模式。常用的元字符包括:^
、$
、.
、*
、+
、?
、[]
、{}
、()
、|
。
.
:匹配任意单个字符(除换行符)^
:匹配字符串的开始$
:匹配字符串的结束*
:匹配前一个字符0次或多次+
:匹配前一个字符1次或多次?
:匹配前一个字符0次或1次[]
:匹配括号内的任意一个字符{}
:匹配前一个字符的指定次数()
:分组匹配|
:匹配前后任意一个模式
pattern = r'^[a-zA-Z0-9_]+@[a-zA-Z]+\.[a-zA-Z]{2,3}$'
email = 'example123@example.com'
if re.match(pattern, email):
print("Valid email address")
else:
print("Invalid email address")
3、分组与捕获
使用括号()
可以将正则表达式中的一部分括起来,形成一个分组。分组可以通过编号来引用,编号从1开始。
pattern = r'(\d{3})-(\d{2})-(\d{4})'
text = 'My number is 123-45-6789'
match_obj = re.search(pattern, text)
if match_obj:
print(f"Full match: {match_obj.group(0)}")
print(f"Area code: {match_obj.group(1)}")
print(f"Prefix: {match_obj.group(2)}")
print(f"Line number: {match_obj.group(3)}")
4、贪婪与非贪婪匹配
正则表达式默认为贪婪匹配,即尽可能多地匹配字符。通过在量词后加上?
可以实现非贪婪匹配,即尽可能少地匹配字符。
text = '<html><head><title>Title</title>'
pattern_greedy = r'<.*>'
pattern_non_greedy = r'<.*?>'
match_greedy = re.search(pattern_greedy, text)
match_non_greedy = re.search(pattern_non_greedy, text)
print(f"Greedy match: {match_greedy.group()}")
print(f"Non-greedy match: {match_non_greedy.group()}")
二、字符串方法匹配
虽然正则表达式非常强大,但对于一些简单的文字匹配任务,Python内置的字符串方法也是十分有用的。
1、find和rfind
find方法返回子字符串在字符串中首次出现的位置,如果未找到则返回-1。rfind方法则返回子字符串最后一次出现的位置。
text = 'hello world'
substring = 'o'
first_occurrence = text.find(substring)
last_occurrence = text.rfind(substring)
print(f"First occurrence: {first_occurrence}")
print(f"Last occurrence: {last_occurrence}")
2、startswith和endswith
startswith方法检查字符串是否以指定子字符串开头,endswith方法检查字符串是否以指定子字符串结尾。
text = 'hello world'
if text.startswith('hello'):
print("The text starts with 'hello'")
if text.endswith('world'):
print("The text ends with 'world'")
3、count
count方法返回子字符串在字符串中出现的次数。
text = 'hello hello hello'
substring = 'hello'
count = text.count(substring)
print(f"The substring '{substring}' appears {count} times")
4、replace
replace方法用于替换字符串中的子字符串。
text = 'hello world'
new_text = text.replace('world', 'Python')
print(f"Replaced text: {new_text}")
三、difflib库匹配
difflib库提供了很多实用的工具来比较序列,包括字符串。它的SequenceMatcher类可以用于计算两个字符串的相似度。
1、计算相似度
SequenceMatcher类可以用来计算两个字符串的相似度,返回一个0到1之间的浮点数,表示相似度。
from difflib import SequenceMatcher
text1 = 'hello world'
text2 = 'hello Python'
similarity = SequenceMatcher(None, text1, text2).ratio()
print(f"Similarity: {similarity}")
2、获取相似度匹配块
get_matching_blocks方法返回一个三元组列表,表示两个字符串中的匹配块。
matching_blocks = SequenceMatcher(None, text1, text2).get_matching_blocks()
print(f"Matching blocks: {matching_blocks}")
3、获取差异
get_opcodes方法返回一个操作码列表,表示如何将text1转换为text2。
opcodes = SequenceMatcher(None, text1, text2).get_opcodes()
for tag, i1, i2, j1, j2 in opcodes:
print(f"{tag}: text1[{i1}:{i2}] -> text2[{j1}:{j2}]")
总结
通过正则表达式、字符串方法、difflib库,Python提供了丰富的文字匹配工具。正则表达式适用于复杂的匹配任务、字符串方法适用于简单的匹配任务、difflib库适用于相似度计算。根据具体需求选择合适的方法,可以高效地完成文字匹配任务。掌握这些工具,可以大大提升文本处理的能力和效率。
相关问答FAQs:
如何使用Python进行简单的字符串匹配?
在Python中,最常见的字符串匹配方法是使用内置的in
运算符和str.find()
方法。in
运算符可以检查一个字符串是否包含另一个字符串,而str.find()
方法则返回子字符串的起始索引,如果未找到则返回-1。示例代码如下:
text = "Hello, welcome to Python programming."
if "Python" in text:
print("Found 'Python' in the text!")
Python有哪些库可以增强文字匹配的功能?
Python提供了一些强大的库来增强文字匹配功能。re
库用于正则表达式匹配,可以处理复杂的模式匹配需求。fuzzywuzzy
库则用于模糊匹配,适合处理拼写错误或近似匹配的场景。这些库可以帮助开发者在处理文本数据时更加灵活和高效。
如何处理大文本文件中的匹配任务?
处理大文本文件时,可以使用逐行读取的方法来提高效率。使用with open()
语句可以确保文件在使用后被正确关闭。通过这种方式,可以避免一次性加载整个文件带来的内存问题。示例代码如下:
with open("large_file.txt", "r") as file:
for line in file:
if "search_term" in line:
print("Found in line:", line)
这种方法让你能够高效地搜索并匹配大文本文件中的特定内容。
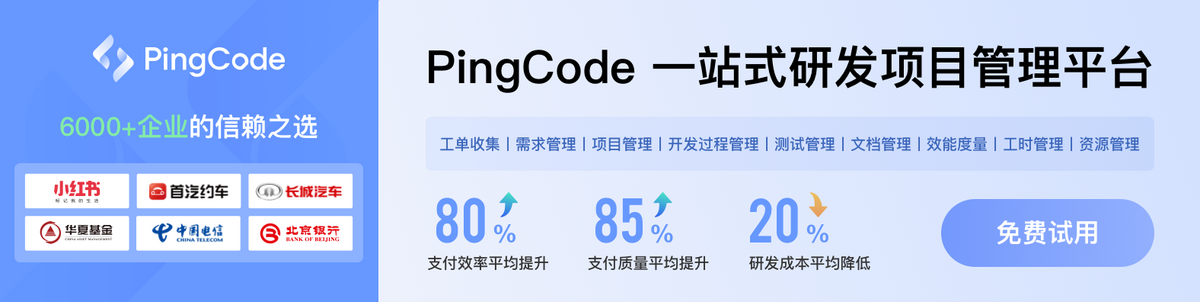